JavaScript is a widely used programming language that is dynamic and flexible. In JavaScript, objects are one of its most powerful and commonly used language constructs. This article will introduce the usage, characteristics and common applications of JavaScript objects.
1. Introduction to JavaScript objects
JavaScript objects are a data type used to encapsulate multiple attributes and form a set of data. Its syntax can be created using object literals, constructors, or the Object.create() method.
- Object literal method
Object literal method is the simplest and most commonly used method to create objects in JavaScript. The syntax is as follows:
var obj = { propertyName1: value1, propertyName2: value2, ... propertyNameN: valueN };
Among them, propertyName
is the property name and value
is the property value. Object literals can be thought of as a JSON-like way of creating objects.
- Constructor method
The constructor method is a method of creating an object by defining a constructor. The syntax is as follows:
function Object(propertyName1, propertyName2, ..., propertyNameN) { this.propertyName1 = propertyName1; this.propertyName2 = propertyName2; ... this.propertyNameN = propertyNameN; } var obj = new Object(value1, value2, ..., valueN);
Among them, the this
keyword represents the current object, and the attribute value is passed in through parameters.
- Object.create() method
Object.create() is a method to create an object. It can be used to create a new object and specify the prototype of the object. object. The syntax is as follows:
var obj = Object.create(proto, [propertiesObject])
Among them, proto
represents the prototype object, and propertiesObject
represents the properties to be added to the object and their property values.
2. JavaScript object characteristics
JavaScript objects have the following characteristics:
- Object properties
JavaScript objects consist of a set of Attribute composition. Property names can be strings or symbols, and property values can be any type of data, including simple types and object types. Use the property name to access the property value, for example:
var obj = {name: "Tom", age: 20}; console.log(obj.name); // 输出:"Tom"
- Property descriptor
Each object property has some descriptors, including enumerable, writable, Configurable and values etc. These property descriptors can be obtained using the Object.getOwnPropertyDescriptor() method.
- Object methods
JavaScript objects can also contain methods, which are a special type of object property whose value is a function. Methods can be used to manipulate objects and their properties. For example:
var obj = { name: "Tom", age: 20, sayHello: function() { console.log("Hello, my name is " + this.name + ", I am " + this.age + " years old."); } }; obj.sayHello(); // 输出:"Hello, my name is Tom, I am 20 years old."
- Object prototype
Objects in JavaScript can inherit properties and methods through prototypes. Every object has a prototype object, and you can use the Object.getPrototypeOf() method to get the prototype of the object.
var parent = {x: 1}; var child = Object.create(parent); console.log(child.x); // 输出:1
3. Common applications of JavaScript objects
- Batch creation of objects
Using the object literal method, objects can be created in batches.
var arrOfObj = [ {name: "Tom", age: 20}, {name: "Jerry", age: 21}, {name: "Mickey", age: 22} ];
- Accessing and modifying object properties
Use .
and []
to access and modify object properties.
var obj = {name: "Tom", age: 20}; console.log(obj.name); // 输出:"Tom" obj.age = 21; console.log(obj["age"]); // 输出:21
- Passing objects as function parameters
JavaScript objects can be used as function parameters to pass information. For example:
function printObjInfo(obj) { console.log("Object name is " + obj.name + ", and age is " + obj.age); } var obj = {name: "Tom", age: 20}; printObjInfo(obj); // 输出:"Object name is Tom, and age is 20"
- Inheritance
Through prototypal inheritance, objects can share properties and methods. For example:
function Person(name, age) { this.name = name; this.age = age; } Person.prototype.sayHello = function() { console.log("Hello, my name is " + this.name + ", I am " + this.age + " years old."); }; function Student(name, age, grade) { Person.call(this, name, age); this.grade = grade; } Student.prototype = Object.create(Person.prototype); Student.prototype.constructor = Student; Student.prototype.study = function() { console.log(this.name + " is studying in grade " + this.grade); }; var stu = new Student("Tom", 20, 3); stu.sayHello(); // 输出:"Hello, my name is Tom, I am 20 years old." stu.study(); // 输出:"Tom is studying in grade 3"
4. Summary
JavaScript object is a very important language structure used to encapsulate data and behavior. Object literals, constructors, and Object.create() are the three ways to create objects. Objects have many characteristics, including properties, methods, property descriptors, prototypes, etc. Objects can be used to create, access and modify properties in batches, pass parameters and implement inheritance, etc. Proficient in basic object-related knowledge can help developers quickly create, manipulate, and manage complex data structures in JavaScript code.
The above is the detailed content of javascript object usage. For more information, please follow other related articles on the PHP Chinese website!
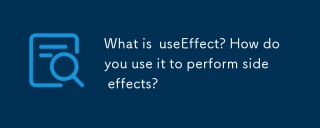
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
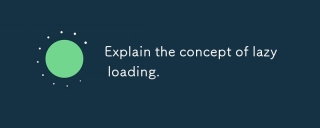
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
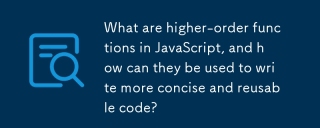
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
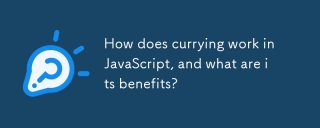
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
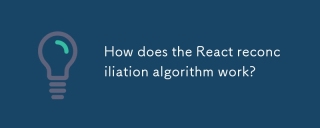
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
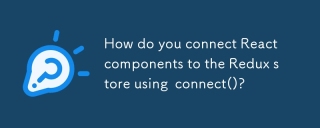
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
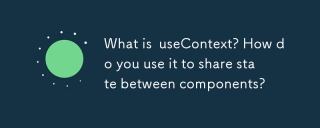
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
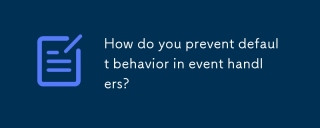
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
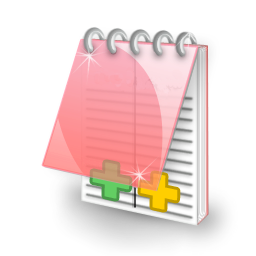
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
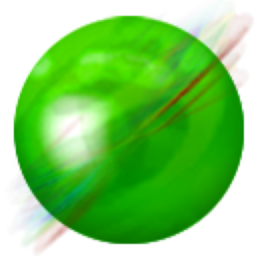
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
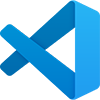
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
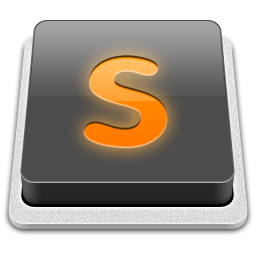
SublimeText3 Mac version
God-level code editing software (SublimeText3)
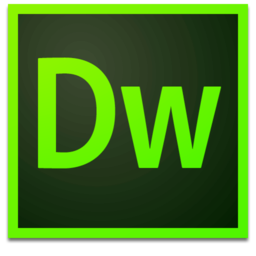
Dreamweaver Mac version
Visual web development tools