Bind a single attribute
Basic binding
To customize the component CustomInput
Example
<script setup> const txt = ref(''); </script> <template> <CustomInput v-model="txt" /> </template>
v-model
It will be expanded into the following form
<CustomInput :modelValue="txt" @update:modelValue="newValue => txt = newValue" />
<custominput></custominput>
Two things need to be done inside the component:
Convert the internal native
<input>
Thevalue
attribute of the element is bound to themodelValue
prop- ## when the native
input
When the event is triggered, a
update:modelValuecustom event carrying a new value is triggered
<script setup> const props = defineProps({ 'modelValue': String, }) const emit = defineEmits(["update:modelValue"]) </script> <template> <input :value="modelValue" @input="$emit('update:modelValue', $event.target.value)" /> </template>Some people You will feel that this way of writing is too cumbersome and will cause the tag code to become lengthy.Another way to implement
v-model within the component is to use a writable one that has both getter and The
computed attribute of the setter
computed attribute, the
get method needs to return
modelValue prop, and the
set method needs to trigger the corresponding event
<script setup> const value = computed({ get() { return props.modelValue }, set(value) { emit("update:modelValue", value) } }) </script> <template> <input v-model="value" /> </template>This writing method can simplify the attributes in the tag, and the logic is clearA single attribute can be used
v-model Easy to do, what if multiple attributes need two-way binding?
v- model uses
modelValue as prop on the components, and uses
update:modelValue as the corresponding event
v -model Specify a parameter to change these names:
<template> <CustomInput v-model:first-name="first" v-model:last-name="last" /> </template>Similarly, it can also be bound in two ways, but
prop is changed from the original
modelValue Changed to the passed in parameter name, the corresponding event also changed to
update: parameter name
<script setup> const props = defineProps({ firstName: String, lastName: String, }) // 在computed中 使用 const emit = defineEmits(['update:firstName', 'update:lastName']) </script> <template> <input type="text" :value="firstName" @input="$emit('update:firstName', $event.target.value)" /> <input type="text" :value="lastName" @input="$emit('update:lastName', $event.target.value)" /> </template>Binding objectIn a complex component, if there are multiple A field requires two-way binding. If you use the method shown above, it will be a bit cumbersome.Introducing two methods of two-way binding objectsDefine the parent component
searchBar For a complex form component
<script setup> import { ref } from "vue" const modelValue = ref({ keyword: "123", selectValue: "", options: [ { label: "全部", value: "" }, { label: "a1", value: "1" }, { label: "a2", value: "2" }, ] }) </script> <template> <searchBar v-model="modelValue" /> </template>Then in the
searchBar component, we receive
modelValue and define the type as
Object
<template> <div> <!-- <input type="text" v-model="modelValue.keyword"> 可以实现双向绑定 --> <input type="text" :value="modelValue.keyword" @input="handleKeywordChange" > <select v-model="modelValue.selectValue"> <option v-for="o in modelValue.options" :key="o.value" :value="o.value"> {{ o.label }} </option> </select> </div> </template> <script lang="ts" setup> const props = defineProps({ modelValue: { type: Object, default: () => ({}) } }) const emit = defineEmits(["update:modelValue"]); // 以 input 举例 const handleKeywordChange=(val)=>{ emit("update:modelValue",{ ...props.modelValue, keyword:val.target.value }) } </script>
If an object is passed in, as described in the commentsis the same as the
Although it can be done directly in both directions Binding, but this will destroy the
single data flow
emit trigger event above, but the passed data becomes an object
computed prxoy
computed binding, you may write this code
<template> <input type="text" v-model="model.keyword"> </template> <script lang="ts" setup> const model = computed({ get() { return props.modelValue }, set(value) { // console.log(value) // 发现没有打印 emit("update:modelValue", { ...props.modelValue, keyword: value }) } }) <script>But when you enter, you will find that it is not triggered
setter, because
computed will be a layer of proxy, the proxy object is not modified
setter, as shown below:
// 只有这样才会变化 model.value = { keyword:"asdfad" }This method cannot trigger
setter, so two-way binding cannot be done. What should I do?
proxy object in getter
! Return a proxy object in getter
! Return a proxy object in getter
!
proxy the proxy object is consistent with the properties of the proxied object, so we use
proxy to wrap the original object
v-model is bound to the object behind the proxy. If the properties of the proxy object change, the
set method in the proxy object will be triggered. At this time we can trigger
emit
const model = computed({ get() { return new Proxy(props.modelValue, { set(obj, name, val) { emit("update:modelValue", { ...obj, [name]: val }) return true } }) }, set(value) { emit("update:modelValue", { ...props.modelValue, keyword: value }) } })ModifiersWe know that
v-model has some built-in modifiers, such as
.trim,
.number and
.lazy.
v-model to support custom modifiers.
capitalize, which will automatically convert the first letter of the string value of the
v-model binding input to uppercase :
<CustomInput v-model.capitalize="txt" />We added the
capitalize modifier, which will be automatically passed into the
modelModifiers
in prop
中
<script setup> const props = defineProps({ modelValue: String, modelModifiers: { default: () => ({}) } }) const emitValue = (e) => { let value = e.target.value; // 使用 修饰符 if (props.modelModifiers.capitalize) { value = value.charAt(0).toUpperCase() + value.slice(1) } emit('update:modelValue', value) } </script> <template> <input :value="modelValue" @input="emitValue" /> </template>
The above is the detailed content of How to use v-model in vue3. For more information, please follow other related articles on the PHP Chinese website!
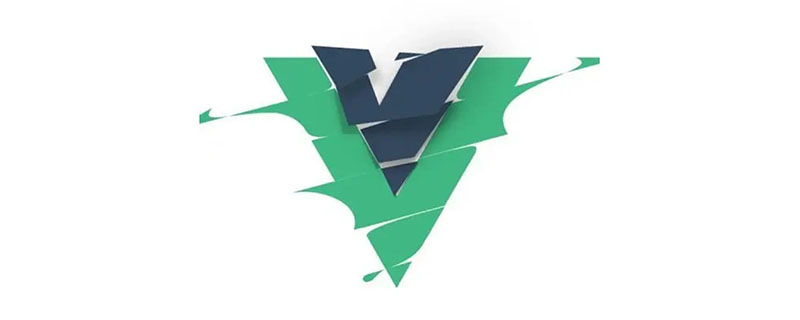
前端有没有现成的库,可以直接用来绘制 Flowable 流程图的?下面本篇文章就跟小伙伴们介绍一下这两个可以绘制 Flowable 流程图的前端库。
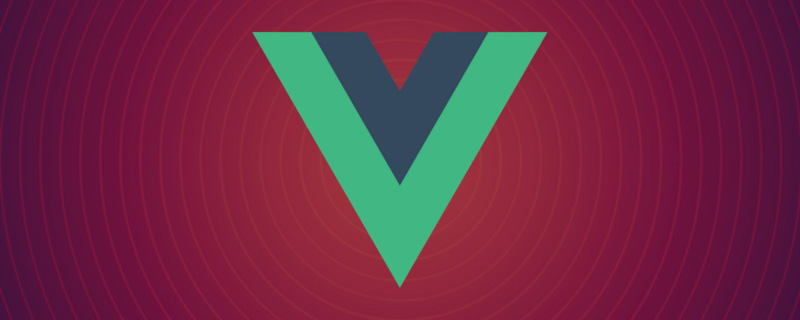
vue不是前端css框架,而是前端JavaScript框架。Vue是一套用于构建用户界面的渐进式JS框架,是基于MVVM设计模式的前端框架,且专注于View层。Vue.js的优点:1、体积小;2、基于虚拟DOM,有更高的运行效率;3、双向数据绑定,让开发者不用再去操作DOM对象,把更多的精力投入到业务逻辑上;4、生态丰富、学习成本低。
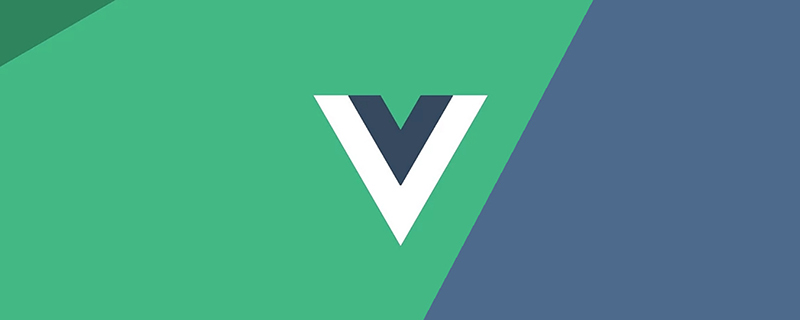
Vue3如何更好地使用qrcodejs生成二维码并添加文字描述?下面本篇文章给大家介绍一下Vue3+qrcodejs生成二维码并添加文字描述,希望对大家有所帮助。
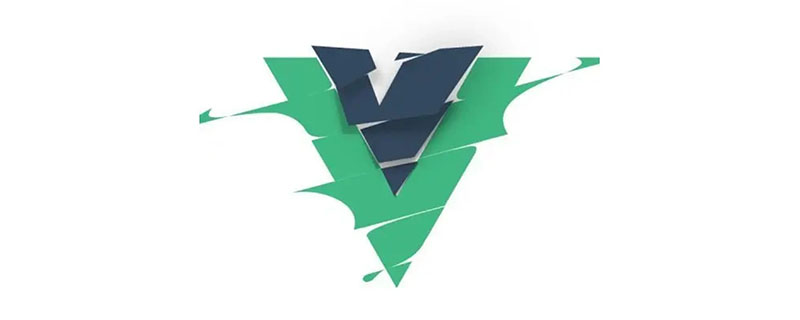
本篇文章我们来了解 Vue2.X 响应式原理,然后我们来实现一个 vue 响应式原理(写的内容简单)实现步骤和注释写的很清晰,大家有兴趣可以耐心观看,希望对大家有所帮助!


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
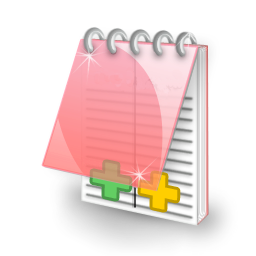
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
