Golang is a statically typed language. Its syntax is somewhat different from other languages. One of its unique language features is interface
, which is also an important concept in Golang. Unlike interfaces in other languages, Golang's interface
is very flexible, and its implementation and meaning are different from other languages. This article will explain interface
in Golang in detail from multiple angles to help readers better understand and use this concept.
- The concept of interface
In Golang, an interface
is a collection of methods. These methods are defined in interface
, but their implementation is implemented by other types. This means that a type can implement multiple interface
, and even if two interface
define the same method, they are different types. This can provide different behaviors for instances of the same type on different occasions, which is very flexible.
- Implementation of interface
In Golang, the way to implement interface
is very flexible. We can implement interface
for specific types, or through struct
.
For example, the code in the following example shows how to implement a simple interface
through a custom type.
package main import "fmt" type MyInt int type MyInterface interface { Print() } func (m MyInt) Print() { fmt.Println(m) } func main() { var i MyInterface = MyInt(5) i.Print() }
In this example, we define a type named MyInt
and an interface named MyInterface
. MyInt
Satisfies the MyInterface
interface by implementing the Print
method defined in MyInterface
. Then, we create a variable of type MyInt
and assign it to a variable of type MyInterface
. The type conversion MyInt(5)
here is necessary because MyInt
and MyInterface
are different types and require explicit conversion.
- Interface Nesting
In Golang, interfaces can be nested within other interfaces. This feature is very convenient because it allows us to split the functionality of the interface into multiple interfaces and then combine them.
For example, the code in the following example shows how to nest multiple interfaces.
package main import "fmt" type ReadInterface interface { Read() string } type WriteInterface interface { Write(data string) } type ReadWriteInterface interface { ReadInterface WriteInterface } type File struct { name string } func (f File) Read() string { return f.name } func (f File) Write(data string) { fmt.Println("writing ", data, " to file ", f.name) } func main() { file := File{name: "test.txt"} var i ReadWriteInterface = file fmt.Println(i.Read()) i.Write("Hello, World!") }
In this example, we define three different interfaces: ReadInterface
, WriteInterface
and ReadWriteInterface
. Then we created a struct
type named File
and implemented the Read
and Write
methods to satisfy the ReadInterface
and WriteInterface
interfaces. Finally, we assign an instance of type File
to a variable of type ReadWriteInterface
and call the Read
and Write
methods.
Such a nesting feature is very useful because it allows us to decompose the interface into smaller parts, each part can be implemented by a different type.
- Empty interface
In Golang, use interface{}
to define an empty interface, which is a superset of all other types. In other words, the interface{}
type can accept any type of value as parameter and return type. Such an empty interface is very flexible and is often used to store arbitrary types of data or when the parameter type is not certain.
For example, the code in the following example shows how to define and use an empty interface.
package main import "fmt" func Describe(i interface{}) { fmt.Printf("Type = %T, Value = %v ", i, i) } func main() { Describe(5) Describe(3.14) Describe("Hello, World!") }
In this example, we define a function named Describe
and use the interface{}
type as its parameter type. Then, we call this function three times, passing integers, floats, and strings as parameters. This function can accept values of any type and print out their type and value.
- Type judgment of empty interface
When using empty interface, sometimes we need to check whether a value meets the requirements of a certain interface, which requires the use of type Type assertion. Using type assertions, you can check at runtime whether the type of a value is the implementation type of an interface.
For example, the code in the following example shows how to type assertion to check whether a value is an implementation type of an interface.
package main import "fmt" type MyInterface interface { Print() } type MyStruct struct{} func (m MyStruct) Print() { fmt.Println("Hello, World!") } func main() { var i interface{} = MyStruct{} value, ok := i.(MyInterface) if ok { fmt.Println("type assertion succeeded") value.Print() } }
In this example, we create an interface named MyInterface
and a struct
type named MyStruct
, and MyStruct
implements the Print
method. Then, we assign an instance of type MyStruct
to an empty interface type variable i
. Next, we use a type assertion to check whether this variable is the implementation type of the MyInterface
interface. If so, output "type assertion succeeded" and call the Print
method. Otherwise, do nothing.
- 接口和类型的转换
在 Golang 中,接口和类型之间的相互转换是一个比较广泛的主题。在实际应用中,经常会出现将一个接口转换成某个类型的需求,或者将一个类型转换成接口的需求。这里我们简单介绍几种常见的转换方式。
下面这个例子展示了如何将 interface{}
类型转换成 string
类型:
package main import "fmt" func main() { var i interface{} = "Hello, World!" s := i.(string) fmt.Println(s) }
这个例子中,我们创建了一个字符串类型的实例,并将其赋值给一个空接口类型的变量 i
。接下来,我们使用类型断言将 i
转换成字符串类型,并将转换结果存放在变量 s
中,最后输出转换后的结果。
下面这个例子展示了如何将一个类型转换成接口类型:
package main import "fmt" type MyInterface interface { Print() } type MyStruct struct{} func (m MyStruct) Print() { fmt.Println("Hello, World!") } func main() { s := MyStruct{} var i MyInterface = s i.Print() }
这个例子中,我们先定义了一个名为 MyInterface
的接口和一个名为 MyStruct
的 struct
类型。MyStruct
实现了 MyInterface
中定义的 Print
方法。然后,我们创建了一个 MyStruct
类型的实例 s
,并将其转换成 MyInterface
接口类型的变量 i
。接下来,我们调用 i
变量的 Print
方法,输出“Hello, World!”。
- 总结
Golang 中的 interface
是一个非常重要的概念,它提供了非常灵活的方法来定义多态行为。在实际应用中,使用 interface
可以帮助我们更好的构建一个简洁、高效的程序,提高代码复用率,提高程序设计的可扩展性和可维护性。掌握 interface
的使用方法是 Golang 程序员必不可少的一项技能。
The above is the detailed content of How golang understands interface. For more information, please follow other related articles on the PHP Chinese website!
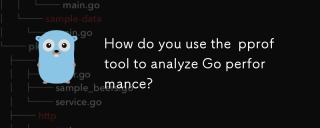
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
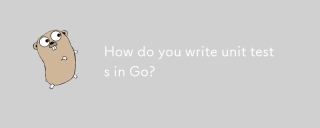
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
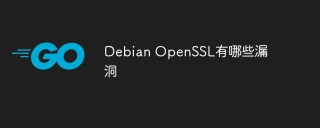
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
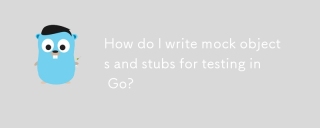
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
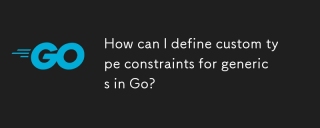
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
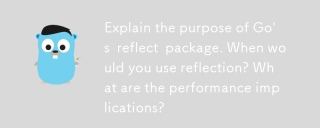
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
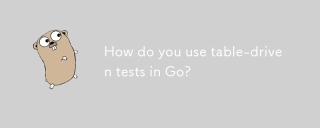
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
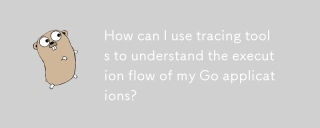
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
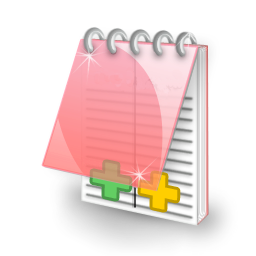
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
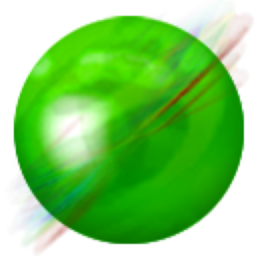
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
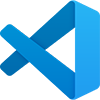
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
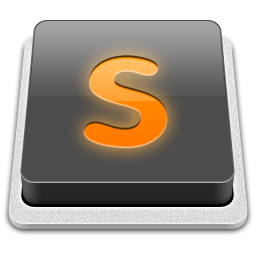
SublimeText3 Mac version
God-level code editing software (SublimeText3)
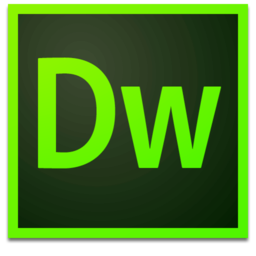
Dreamweaver Mac version
Visual web development tools