In the field of cloud computing, container technology is favored for its lightweight, fast operation, portability and efficiency. As a representative of container technology, Docker has become a popular tool in cloud computing, DevOps and other fields by providing a lightweight way to package and deploy applications. For enterprise-level applications, Docker clusters are needed to achieve high availability, elastic scaling and other functions. This article introduces how to use Golang to build a Docker cluster.
1. Overview of Docker cluster
Docker cluster refers to the cooperation of multiple Docker hosts to achieve functions such as deployment, management and monitoring of applications. Docker clusters usually consist of the following basic concepts:
- Docker host
Docker host refers to the computer or virtual machine running the Docker engine. Each Docker host can deploy and run multiple Docker containers.
- Docker Swarm
Docker Swarm is a container orchestration tool officially provided by Docker. It can manage containers on multiple Docker hosts and implement it by defining concepts such as services and tasks. Application deployment and management.
- Service
Service is a group of containers in a Docker cluster with common functions and specifications, such as Web services, database services, etc. Service can define multiple replica instances to achieve functions such as high availability and load balancing.
- Task
Task is an instance of Service, that is, a container running on a certain Docker host. Tasks can be scheduled and managed by Docker Swarm to realize automated deployment and management of containers.
- Node
Node is a Docker host in the Docker cluster and can run multiple Tasks and Services.
2. Golang implements Docker Swarm
Docker Swarm provides RESTful API and CLI tools to manage and control Docker clusters. Golang, as an efficient, concurrent, cross-platform programming language, is widely used in system programming and network programming. The following describes how to use Golang to implement the basic functions of Docker Swarm.
- Install Docker SDK for Golang
Docker SDK for Golang is the official client provided by Docker and can easily communicate with the Docker server. Docker SDK for Golang can be installed using the following command:
go get -u github.com/docker/docker/client
- Implementing Docker Swarm API encapsulation
The Docker Swarm API can be called through HTTP requests and returns data in JSON format. We can use Golang to encapsulate the Docker Swarm API for quick and convenient calls. For example, define the following structure:
type SwarmClient struct { cli *client.Client ctx context.Context } type SwamService struct { ID string `json:"ID"` Name string `json:"Name"` Endpoint Endpoint `json:"Endpoint"` } type Endpoint struct { Spec EndpointSpec `json:"Spec"` } type EndpointSpec struct { Ports []PortConfig `json:"Ports"` } type PortConfig struct { Protocol string `json:"Protocol"` TargetPort uint32 `json:"TargetPort"` PublishedPort uint32 `json:"PublishedPort"` }
We can use Golang's HTTP package to implement corresponding HTTP request operations such as GET, POST, PUT, and DELETE.
- Implement Docker Swarm CLI tool
In addition to using RESTful API calls, we can also implement Docker Swarm CLI tool to facilitate Docker Swarm cluster more intuitively management and operations. For example, implement the following command:
docker-swarm service create [OPTIONS] IMAGE [COMMAND] [ARG...]
This command can create a Service service using the specified image and command parameters. We can use Golang to implement corresponding operations, for example:
func createService(image string, command []string, port uint32) { service := &swarm.ServiceSpec{ TaskTemplate: swarm.TaskSpec{ ContainerSpec: swarm.ContainerSpec{ Image: image, Command: command, Env: []string{"PORT=" + strconv.Itoa(int(port))}, }, }, EndpointSpec: &swarm.EndpointSpec{ Ports: []swarm.PortConfig{ swarm.PortConfig{ Protocol: swarm.PortConfigProtocolTCP, TargetPort: uint32(port), PublishedPort: uint32(port), }, }, }, } cli, ctx := initCli() serviceCreateResponse, err := cli.ServiceCreate(ctx, *service, types.ServiceCreateOptions{}) if err != nil { panic(err) } }
This function can use Docker SDK for Golang to create a Service service and specify parameters such as image, command and port.
- Implement monitoring and logging of the Docker Swarm cluster
During the running process of the Docker Swarm cluster, we need to monitor it in real time and view the logs. We can use Golang to implement corresponding programs and obtain cluster status and container logs by using the API provided in Docker SDK for Golang. For example:
func listServices() { cli, ctx := initCli() services, err := cli.ServiceList(ctx, types.ServiceListOptions{}) if err != nil { panic(err) } for _, service := range services { fmt.Printf("[Service] ID:%s Name:%s ", service.ID, service.Spec.Name) } } func getServiceLogs(serviceID string) { cli, ctx := initCli() reader, err := cli.ServiceLogs(ctx, serviceID, types.ContainerLogsOptions{}) if err != nil { panic(err) } defer reader.Close() scanner := bufio.NewScanner(reader) for scanner.Scan() { fmt.Println(scanner.Text()) } }
The above code implements operations such as obtaining the Service list in the Docker Swarm cluster and obtaining the logs of the specified Service.
3. Use Docker Compose to implement Docker Swarm cluster
Docker Compose is a container orchestration tool provided by Docker, which can manage multiple containers and services by defining compose files. We can use Docker Compose to quickly build and manage Docker Swarm clusters. For example, define the following compose file:
version: '3' services: web: image: nginx deploy: mode: replicated replicas: 3 resources: limits: cpus: "0.1" memory: 50M reservations: cpus: "0.05" memory: 30M restart_policy: condition: on-failure delay: 5s max_attempts: 3 ports: - "80:80" networks: - webnet visualizer: image: dockersamples/visualizer:stable ports: - "8080:8080" stop_grace_period: 30s volumes: - /var/run/docker.sock:/var/run/docker.sock deploy: placement: constraints: [node.role == manager] networks: - webnet networks: webnet:
This compose file defines a web service and a visualization tool, using the nginx image and dockersamples/visualizer image as services. Among them, the Web service usage mode is replicated service deployment method, which will use 3 replica instances, and set CPU and memory resource limits, restart policy and other configurations. The visualization tool uses the Docker host node with node.role as manager as the deployment node to easily view the Docker Swarm cluster status.
We can use the following command to start Docker Compose:
docker stack deploy -c docker-compose.yml webapp
This command will create the corresponding Service and Task instances based on the configuration items defined in the compose file, and start the Docker Swarm cluster. We can view the real-time status of the Docker Swarm cluster by accessing http://localhost:8080.
Summary
This article introduces how to use Golang to implement the basic functions of a Docker Swarm cluster and how to use Docker Compose to quickly build and manage a Docker Swarm cluster. In practical applications, Docker Swarm clusters can provide high availability, elastic scaling and other functions, and can achieve efficient management and deployment of containerized applications.
The above is the detailed content of dokcer cluster golang build. For more information, please follow other related articles on the PHP Chinese website!
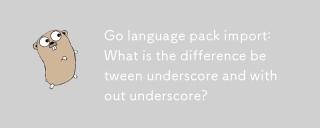
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
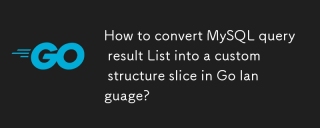
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
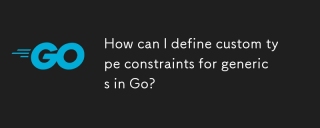
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
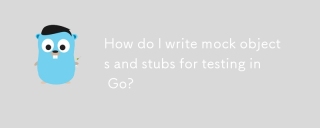
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
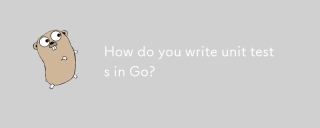
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
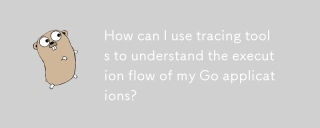
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
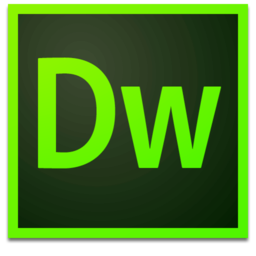
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
