In the Go language, error handling is an important task. Error handling means you need to be able to check that the function performed the correct operation, and in some cases, you need to take action to avoid further errors or damage. In this article, I will discuss the error handling mechanism in golang and how to catch error types.
The error handling mechanism of Go language is handled through the error interface. Error is a predefined interface type in the Go language, defined as follows:
type error interface { Error() string }
All types that implement the Error() method implement the error interface, and therefore can be regarded as error types. In golang, when a function fails to execute or encounters an error, it usually returns a value of type error. For example:
func foo() error { if err := doSomething(); err != nil { return err } return nil }
In the above example, the function foo() will return the error value when the doSomething() function returns an error, otherwise it will return nil.
When we are using functions, we can use if statements to check if an error occurs and take appropriate action as needed. Here is an example:
func main() { err := foo() if err != nil { log.Printf("An error occurred: %v", err) } }
In the above code, if the foo() function returns an error, it will be logged, otherwise nothing will be done.
In some cases we need to check the type of error so that we can take appropriate action. For example, in an http application, we might need to return different HTTP status codes based on the type of error. To do this, we can use type assertions to convert the error type to a specific type of error. For example:
func handleRequest(w http.ResponseWriter, r *http.Request) { … if err != nil { switch err := err.(type) { case *myCustomError: http.Error(w, err.Error(), http.StatusBadRequest) case *otherCustomError: http.Error(w, err.Error(), http.StatusInternalServerError) default: http.Error(w, err.Error(), http.StatusInternalServerError) } } }
In the above code, we first use type assertion to convert the error into a specific type of error. Then, in the switch statement, different HTTP status codes are returned based on the error type. If the error type is not any of the known ones, we can return a default status code.
Through type assertion, we can convert the error type into other specific types of error types. The following is a sample code:
type myCustomError struct { message string } func (e *myCustomError) Error() string { return e.message } func main() { err := &myCustomError{message: "Something went wrong!"} if err != nil { if customErr, ok := err.(*myCustomError); ok { log.Printf("A custom error occurred: %s", customErr.message) } } }
In this example, we first create a custom error type myCustomError, which implements the Error() method. In the main() function we convert the error to myCustomError type and check its type. If this type of error occurs, log it. Otherwise, no action is performed.
In short, through the error interface and type assertion mechanism of the Go language, we can easily handle errors and capture error types. I hope this article can help you better understand the error handling mechanism in the Go language.
The above is the detailed content of golang capture error type. For more information, please follow other related articles on the PHP Chinese website!
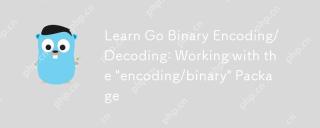
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
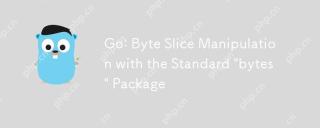
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
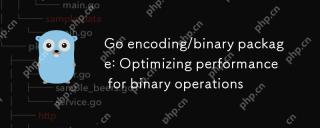
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
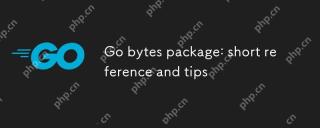
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
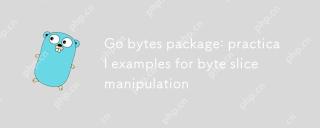
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.
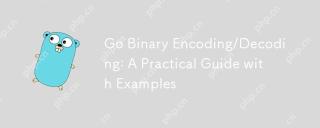
Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian byte order and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when data is transmitted between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
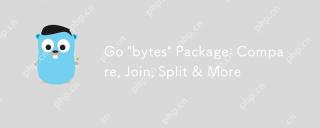
The"bytes"packageinGoisessentialbecauseitoffersefficientoperationsonbyteslices,crucialforbinarydatahandling,textprocessing,andnetworkcommunications.Byteslicesaremutable,allowingforperformance-enhancingin-placemodifications,makingthispackage
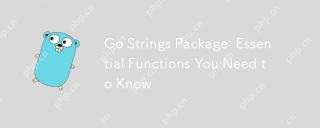
Go'sstringspackageincludesessentialfunctionslikeContains,TrimSpace,Split,andReplaceAll.1)Containsefficientlychecksforsubstrings.2)TrimSpaceremoveswhitespacetoensuredataintegrity.3)SplitparsesstructuredtextlikeCSV.4)ReplaceAlltransformstextaccordingto


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor
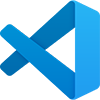
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
