Go language (Golang) is an open source statically strongly typed programming language with the characteristics of efficiency, concurrency, and simplicity. With the popularity of Go language, more and more programmers have begun to learn and use this language. In the Go language, functions are a crucial component. This article will introduce in detail what Golang functions are and how to use them.
First of all, what is a function?
In computer science, a function is a block of code that specifies a task, has input parameters, and a return value that produces output. Functions are often used to separate and organize blocks of code together, thereby improving code reusability and maintainability. In Golang, a function definition starts with the keyword "func", followed by the function name, parameter list, function body and return value.
The following is the definition of a basic Golang function:
func add(x, y int) int { return x + y }
This function is named "add". It receives two int type parameters (x, y) and compares them. Add and return the result. The last line of the function definition specifies the return value type, here it is of type int. If the function does not require a return value, the return value type can be omitted.
Golang supports multiple parameters and multiple return values, for example:
func swap(x, y string) (string, string) { return y, x }
This function is called "swap", which receives two string type parameters (x, y) and returns A tuple containing two string types (y, x).
In Golang, you can also use variable-length parameters, which can receive an indefinite number of parameters. Variable-length parameters are generally used for functions that need to process large amounts of data, such as calculating sums, averages, etc.
func sum(nums ...int) int { total := 0 for _, num := range nums { total += num } return total }
This function is called "sum". It receives any number of int type parameters, uses a for loop to iterate each parameter, calculate their sum, and finally returns the sum value.
There is also a special function type called anonymous function. An anonymous function is a function without a name, usually used as a function variable or closure. Anonymous functions can be defined and executed at any time, for example:
func main() { func() { fmt.Println("Hello, world!") }() }
This example defines an anonymous function that prints the "Hello, world!" message and then executes it immediately in the main function.
In Golang, you can also pass functions as parameters to other functions, which is called functional programming. This programming style can greatly simplify the code, for example:
func apply(nums []int, f func(int) int) []int { result := make([]int, len(nums)) for i, num := range nums { result[i] = f(num) } return result } func double(x int) int { return x * 2 } func main() { nums := []int{1, 2, 3} doubled := apply(nums, double) fmt.Println(doubled) // Output: [2 4 6] }
In this example, the apply function receives an array of type int and a function as parameters, which will modify each element in the array. The double function defines an operation that doubles an argument of type int. In the main function, we use the apply function to apply the double function to the array nums and return the result.
In short, functions are a very important and basic concept in Golang. In addition to the common uses mentioned above, functions can also be used for many other purposes in Golang. When writing Golang programs, it is crucial to understand how to define and use functions to make your code more efficient, concise, and easy to maintain.
The above is the detailed content of what is golang function. For more information, please follow other related articles on the PHP Chinese website!
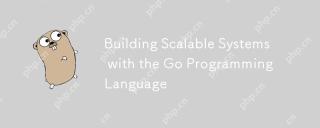
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
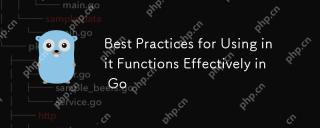
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
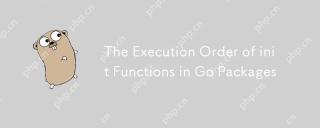
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations
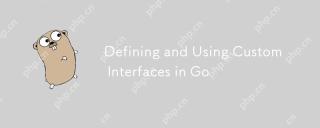
CustominterfacesinGoarecrucialforwritingflexible,maintainable,andtestablecode.Theyenabledeveloperstofocusonbehavioroverimplementation,enhancingmodularityandrobustness.Bydefiningmethodsignaturesthattypesmustimplement,interfacesallowforcodereusabilitya
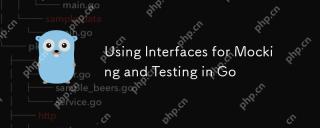
The reason for using interfaces for simulation and testing is that the interface allows the definition of contracts without specifying implementations, making the tests more isolated and easy to maintain. 1) Implicit implementation of the interface makes it simple to create mock objects, which can replace real implementations in testing. 2) Using interfaces can easily replace the real implementation of the service in unit tests, reducing test complexity and time. 3) The flexibility provided by the interface allows for changes in simulated behavior for different test cases. 4) Interfaces help design testable code from the beginning, improving the modularity and maintainability of the code.
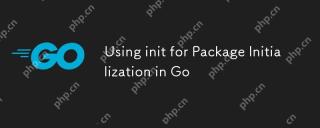
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
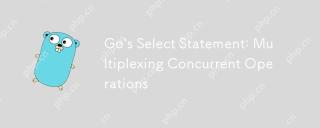
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
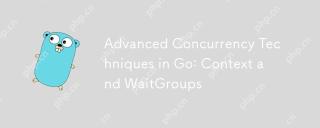
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
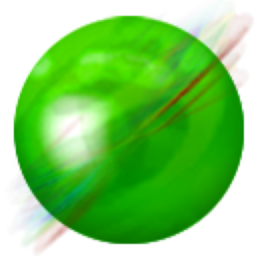
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
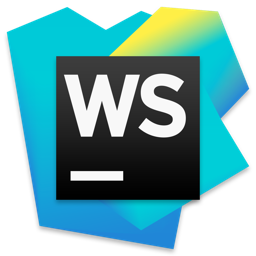
WebStorm Mac version
Useful JavaScript development tools
