1. Introduction
Linked List
is part of theCollection
framework in thejava.util
package.The implementation of LinkedList data structure, which is a linear data structure in which elements
are not stored in consecutive positions
, and each element is a separate object, Has data part and address part.Elements are linked using pointers and addresses. Each element is called a
node
2. Java linked list methods
Method | Description |
add(int index, E element) | This method inserts the specified element at the specified position in this list. |
add(E e) | This method appends the specified element to the end of this list. |
addAll(int index, Collection c) | This method inserts all elements in the specified collection into this list, starting at the specified position. |
addAll(Collection c) | This method appends all elements in the specified collection to the end of this list, in the order they are returned by the iterator of the specified collection . |
addFirst(E e) | This method inserts the specified element at the beginning of this list. |
addLast(E e) | This method appends the specified element to the end of this list. |
clear() | This method removes all elements from this list. |
clone() | This method returns a shallow copy of this LinkedList. |
contains(Object o) | This method returns true if this list contains the specified element. |
descendingIterator() | This method returns an iterator of the elements in this deque in reverse order. |
element() | This method retrieves but does not remove the head (first element) of this list. |
get(int index) | This method returns the element at the specified position in this list. |
getFirst() | This method returns the first element in this list. |
getLast() | This method returns the last element in this list. |
indexOf(Object o) | This method returns the index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element . |
lastIndexOf(Object o) | This method returns the index of the last occurrence of the specified element in this list, or -1 if this list does not contain the element. |
listIterator(int index) | This method returns a list iterator of the elements in this list (in appropriate order), starting at the specified position in the list. |
offer(E e) | This method adds the specified element to the tail (last element) of this list. |
offerFirst(E e) | This method inserts the specified element at the front of this list. |
offerLast(E e) | This method inserts the specified element at the end of this list. |
peek() | This method retrieves but does not remove the head (first element) of this list. |
peekFirst() | This method retrieves but does not delete the first element of this list, or returns null if this list is empty. |
peekLast() | This method retrieves but does not delete the last element of this list, or returns null if this list is empty. |
poll() | This method retrieves and removes the head (first element) of this list. |
pollFirst() | This method retrieves and removes the first element of this list, or returns null if this list is empty. |
pollLast() | This method retrieves and removes the last element of this list, or returns null if this list is empty. |
pop() | This method pops an element from the stack represented by this list. |
push(E e) | This method pushes an element onto the stack represented by this list. |
remove() | This method retrieves and removes the head (first element) of this list. |
remove(int index) | This method removes the element at the specified position in this list. |
remove(Object o) | This method removes the first occurrence of the specified element from this list, if it exists. |
removeFirst() | This method removes and returns the first element from the list. |
removeFirstOccurrence(Object o) | This method removes the first occurrence of the specified element in this list (when traversing the list from beginning to end). |
removeLast() | This method removes and returns the last element from the list. |
removeLastOccurrence(Object o) | This method removes the last occurrence of the specified element in this list (when the list is traversed from beginning to end). |
set(int index, E element) | This method replaces the element at the specified position in this list with the specified element. |
size() | This method returns the number of elements in this list. |
spliterator() | This method creates a late-bound and fail-fast Spliterator on the elements in this list. |
toArray() | This method returns an array containing all the elements in this list in correct order (from the first element to the last element). |
toArray(T[] a) | This method returns an array containing this list in correct order (from first element to last element) all elements of the returned array; the runtime type of the returned array is the type of the specified array. |
toString() | This method returns a string containing all the elements in this list in the correct order (from the first element to the last element) , each element is separated by commas, and the string is enclosed in square brackets. |
3. Code
public class T1 { public static void main(String[] args) { LinkedList<String> ll = new LinkedList<String>(); ll.add("B"); ll.addLast("C"); ll.addFirst("D"); ll.add(2, "E"); System.out.println(ll); } }
[D, B, E, C]
The above is the detailed content of How to implement LinkedList data structure in Java?. For more information, please follow other related articles on the PHP Chinese website!
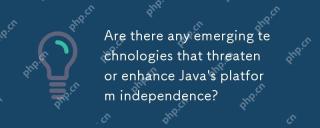
Emerging technologies pose both threats and enhancements to Java's platform independence. 1) Cloud computing and containerization technologies such as Docker enhance Java's platform independence, but need to be optimized to adapt to different cloud environments. 2) WebAssembly compiles Java code through GraalVM, extending its platform independence, but it needs to compete with other languages for performance.
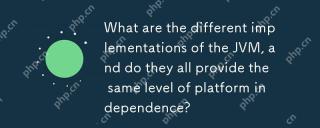
Different JVM implementations can provide platform independence, but their performance is slightly different. 1. OracleHotSpot and OpenJDKJVM perform similarly in platform independence, but OpenJDK may require additional configuration. 2. IBMJ9JVM performs optimization on specific operating systems. 3. GraalVM supports multiple languages and requires additional configuration. 4. AzulZingJVM requires specific platform adjustments.
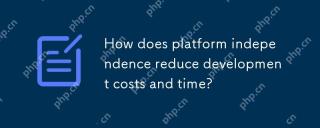
Platform independence reduces development costs and shortens development time by running the same set of code on multiple operating systems. Specifically, it is manifested as: 1. Reduce development time, only one set of code is required; 2. Reduce maintenance costs and unify the testing process; 3. Quick iteration and team collaboration to simplify the deployment process.
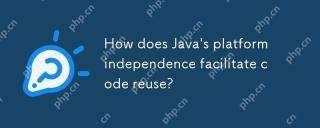
Java'splatformindependencefacilitatescodereusebyallowingbytecodetorunonanyplatformwithaJVM.1)Developerscanwritecodeonceforconsistentbehavioracrossplatforms.2)Maintenanceisreducedascodedoesn'tneedrewriting.3)Librariesandframeworkscanbesharedacrossproj
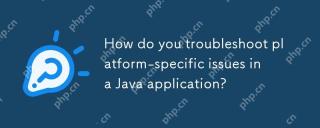
To solve platform-specific problems in Java applications, you can take the following steps: 1. Use Java's System class to view system properties to understand the running environment. 2. Use the File class or java.nio.file package to process file paths. 3. Load the local library according to operating system conditions. 4. Use VisualVM or JProfiler to optimize cross-platform performance. 5. Ensure that the test environment is consistent with the production environment through Docker containerization. 6. Use GitHubActions to perform automated testing on multiple platforms. These methods help to effectively solve platform-specific problems in Java applications.
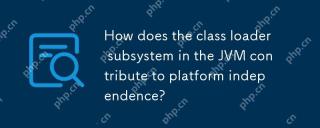
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
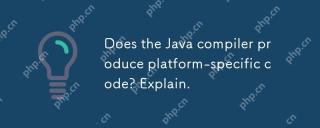
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
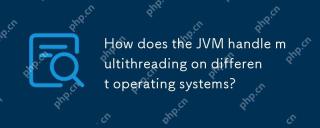
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
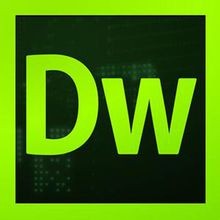
Dreamweaver CS6
Visual web development tools
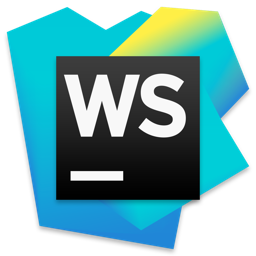
WebStorm Mac version
Useful JavaScript development tools
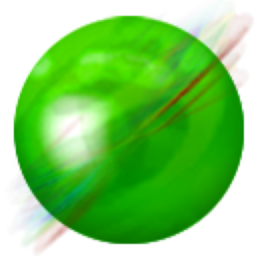
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
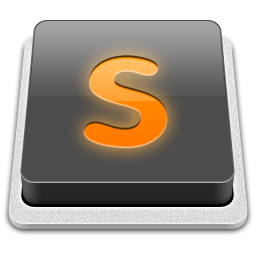
SublimeText3 Mac version
God-level code editing software (SublimeText3)