JavaScript is a programming language used to add dynamic effects and interactivity to web pages. It has many useful methods for manipulating elements in web pages, working with strings and values, creating time intervals and animation effects, and more. In this article, we will discuss some important methods in JavaScript, along with their usage and examples.
1. String method
- .length
This method can return the length of any string.
Example:
let string = "Hello World";
console.log(string.length); // The output result is: 11
- .charAt()
This method returns the character at the specified index.
Example:
let string = "Hello World";
console.log(string.charAt(3)); // The output result is: 'l'
- .concat()
This method can concatenate one or more strings and return a new string.
Example:
let string1 = "Hello";
let string2 = "World";
console.log(string1.concat(" ", string2)); / / The output result is: "Hello World"
- .indexOf()
This method returns the position of the first match in the string, or -1 if no match is found. .
Example:
let string = "Hello World";
console.log(string.indexOf("o")); // The output result is: 4
- .replace()
This method can replace the text in the string.
Example:
let string = "Hello World";
console.log(string.replace("World", "JavaScript")); // The output result is: " Hello JavaScript"
- .slice()
This method can return the specified part of the string.
Example:
let string = "Hello World";
console.log(string.slice(1, 5)); // The output result is: "ello"
2. Array method
- .length
This method can return the length of the array.
Example:
let array = [1, 2, 3, 4, 5];
console.log(array.length); // The output result is: 5
- .push()
This method adds one or more elements to the end of the array and returns the new length value.
Example:
let array = [1, 2, 3, 4, 5];
console.log(array.push(6)); // The output result is: 6
- .pop()
This method can delete the element at the end of the array and return the deleted element.
Example:
let array = [1, 2, 3, 4, 5];
console.log(array.pop()); // The output result is: 5
- .join()
This method can combine all elements of the array into a string.
Example:
let array = [1, 2, 3, 4, 5];
console.log(array.join(",")); // Output results It is: "1,2,3,4,5"
- .splice()
This method can add or remove elements from the array.
Example:
let array = [1, 2, 3, 4, 5];
array.splice(2, 0, "a", "b");
console.log(array); //The output result is: [1, 2, "a", "b", 3, 4, 5]
- .sort()
This method sorts an array.
Example:
let array = [3, 1, 4, 2, 5];
console.log(array.sort()); // The output result is: [ 1, 2, 3, 4, 5]
3. Numerical method
- .valueOf()
This method can return the original value of the numerical value.
Example:
let num = 123;
console.log(num.valueOf()); // The output result is: 123
- . toString()
This method can convert a numerical value into a string type.
Example:
let num = 123;
console.log(num.toString()); // The output result is: "123"
- .toFixed()
This method can retain the specified number of decimal places.
Example:
let num = 3.14159;
console.log(num.toFixed(2)); // The output result is: "3.14"
- .parseInt()
This method can convert a string into an integer type.
Example:
let string = "123";
console.log(parseInt(string)); // The output result is: 123
- .parseFloat()
This method can convert a string into a floating point number type.
Example:
let string = "3.14159";
console.log(parseFloat(string)); // The output result is: 3.14159
4. Event Method
- .addEventListener()
This method can add an event listener for the specified element.
Example:
let button = document.querySelector("button");
button.addEventListener("click", function() {
console.log("Hello World");
});
- .removeEventListener()
This method can remove the event listener for the specified element.
Example:
let button = document.querySelector("button");
function handleClick() {
console.log("Hello World");
}
button.addEventListener("click", handleClick);
button.removeEventListener("click", handleClick);
The above are some commonly used methods in JavaScript. Of course there are many other methods, but mastering the above methods can help us become more comfortable in web development.
The above is the detailed content of Various methods in javascript. For more information, please follow other related articles on the PHP Chinese website!

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.

React is a JavaScript library for building user interfaces. Its core idea is to build UI through componentization. 1. Components are the basic unit of React, encapsulating UI logic and styles. 2. Virtual DOM and state management are the key to component work, and state is updated through setState. 3. The life cycle includes three stages: mount, update and uninstall. The performance can be optimized using reasonably. 4. Use useState and ContextAPI to manage state, improve component reusability and global state management. 5. Common errors include improper status updates and performance issues, which can be debugged through ReactDevTools. 6. Performance optimization suggestions include using memo, avoiding unnecessary re-rendering, and using us

Using HTML to render components and data in React can be achieved through the following steps: Using JSX syntax: React uses JSX syntax to embed HTML structures into JavaScript code, and operates the DOM after compilation. Components are combined with HTML: React components pass data through props and dynamically generate HTML content, such as. Data flow management: React's data flow is one-way, passed from the parent component to the child component, ensuring that the data flow is controllable, such as App components passing name to Greeting. Basic usage example: Use map function to render a list, you need to add a key attribute, such as rendering a fruit list. Advanced usage example: Use the useState hook to manage state and implement dynamics

React is the preferred tool for building single-page applications (SPAs) because it provides efficient and flexible ways to build user interfaces. 1) Component development: Split complex UI into independent and reusable parts to improve maintainability and reusability. 2) Virtual DOM: Optimize rendering performance by comparing the differences between virtual DOM and actual DOM. 3) State management: manage data flow through state and attributes to ensure data consistency and predictability.

React is a JavaScript library developed by Meta for building user interfaces, with its core being component development and virtual DOM technology. 1. Component and state management: React manages state through components (functions or classes) and Hooks (such as useState), improving code reusability and maintenance. 2. Virtual DOM and performance optimization: Through virtual DOM, React efficiently updates the real DOM to improve performance. 3. Life cycle and Hooks: Hooks (such as useEffect) allow function components to manage life cycles and perform side-effect operations. 4. Usage example: From basic HelloWorld components to advanced global state management (useContext and

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.

React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through ReactDevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintaining dependability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
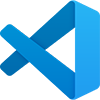
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
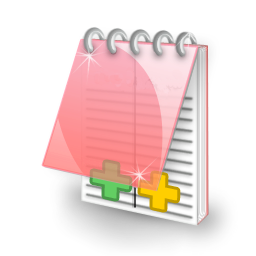
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use