Javascript is a common scripting language that can be used to achieve interactive and dynamic effects on web pages. Among them, hiding buttons is a common requirement. In some cases, we need to hide buttons on the page to prevent users from misoperation or to protect the security of the page. This article will introduce how to use Javascript to hide buttons and achieve interactive effects.
1. What is button hiding?
Button hiding refers to hiding the button element in the web page to prevent users from misoperation or to protect the security of the page. There are two common hiding methods, one is to set the display attribute of the button element to none through CSS style, and the other is to dynamically set the style attribute of the button element through Javascript. Since Javascript can achieve more flexible interactive effects, this article will introduce the latter implementation method.
2. Implementation method of Javascript hidden button
- Get button element
We can get the button element in the web page through Javascript, the method is as follows:
var btn = document.getElementsByTagName('button')[0];
The above code will get the first button element on the page. If there are multiple button elements on the page, we can use the querySelectorAll method to select, for example:
var btns = document.querySelectorAll('button'); var btn = btns[0];
The querySelectorAll method here can obtain matching elements based on the CSS selector, and returns a NodeList type object, which can Get the elements by subscript.
- Hide button element
After obtaining the button element, we can hide it by modifying its style attribute. For example, we can hide the button element by setting its display attribute to none:
btn.style.display = 'none';
The above code will hide the button element and will not take up space on the page.
- Display button element
Sometimes, we need to display button element under certain circumstances. In order to achieve interactive effects, we can dynamically modify the style attribute of the button element when we need to display it. For example:
btn.style.display = 'block';
The above code will display the button element and occupy space on the page.
- Switch the display state of the button element
In addition to hiding and showing the button element, we can also achieve interactive effects by switching its display state. For example, you can set the style.display attribute of the button element to none and block to switch between none and block. The code is as follows:
btn.style.display = btn.style.display == 'none' ? 'block' : 'none';
The above code will switch the display state of the button element when it is clicked.
3. Application scenarios of Javascript hidden buttons
- Prevent misoperation
Sometimes, the button element in the page is too sensitive and can easily cause misoperation. , such as some important operations such as deletion and submission. In this case, we can make an interactive effect. When the user clicks the button element, a dialog box pops up to confirm whether the operation is correct. For example:
btn.onclick = function() { if(confirm('是否确认删除此项?')) { // 执行删除操作 } }
The above code will pop up a dialog box when the user clicks the button element, asking the user to confirm the deletion. If the user clicks the OK button, the corresponding delete operation is performed.
- Interface Optimization
Sometimes, the button elements on the page are too complicated, which can cause visual interference to users. In this case, we can optimize the visual effect of the page by hiding some unnecessary button elements. For example:
var btns = document.querySelectorAll('.unnecessary-btn'); for(var i = 0; i < btns.length; i++) { btns[i].style.display = 'none'; }
The above code will get all the button elements with the class name "unnecessary-btn" in the page and hide them.
- Page Security
Sometimes, the button elements in the page involve some sensitive operations, such as changing passwords, making payments, etc. In this case, we can protect the security of the page by hiding these button elements and only display them in specific situations. For example:
if(user.role == 'admin') { var btns = document.querySelectorAll('.sensitive-btn'); for(var i = 0; i < btns.length; i++) { btns[i].style.display = 'block'; } } else { var btns = document.querySelectorAll('.sensitive-btn'); for(var i = 0; i < btns.length; i++) { btns[i].style.display = 'none'; } }
The above code will display all button elements with the class name "sensitive-btn" when the administrator logs in, based on the user's role, and hide them when other users log in.
4. Summary
Javascript hiding buttons is a common requirement and is also very practical in actual development. This article introduces the implementation method and application scenarios of Javascript hidden buttons, and provides relevant code examples. It should be noted that in actual applications, we also need to flexibly use Javascript to hide buttons based on specific business needs and page layout to achieve better interaction effects and user experience.
The above is the detailed content of javascripthidebutton. For more information, please follow other related articles on the PHP Chinese website!

Classselectorsarereusableformultipleelements,whileIDselectorsareuniqueandusedonceperpage.1)Classes,denotedbyaperiod(.),areidealforstylingmultipleelementslikebuttons.2)IDs,denotedbyahash(#),areperfectforuniqueelementslikeanavigationmenu.3)IDshavehighe

In CSS style, the class selector or ID selector should be selected according to the project requirements: 1) The class selector is suitable for reuse and is suitable for the same style of multiple elements; 2) The ID selector is suitable for unique elements and has higher priority, but should be used with caution to avoid maintenance difficulties.

HTML5hasseverallimitationsincludinglackofsupportforadvancedgraphics,basicformvalidation,cross-browsercompatibilityissues,performanceimpacts,andsecurityconcerns.1)Forcomplexgraphics,HTML5'scanvasisinsufficient,requiringlibrarieslikeWebGLorThree.js.2)I

Yes,onestylecanhavemoreprioritythananotherinCSSduetospecificityandthecascade.1)Specificityactsasascoringsystemwheremorespecificselectorshavehigherpriority.2)Thecascadedeterminesstyleapplicationorder,withlaterrulesoverridingearlieronesofequalspecifici

ThesignificantgoalsofHTML5aretoenhancemultimediasupport,ensurehumanreadability,maintainconsistencyacrossdevices,andensurebackwardcompatibility.1)HTML5improvesmultimediawithnativeelementslikeand.2)ItusessemanticelementsforbetterreadabilityandSEO.3)Its

React'slimitationsinclude:1)asteeplearningcurveduetoitsvastecosystem,2)SEOchallengeswithclient-siderendering,3)potentialperformanceissuesinlargeapplications,4)complexstatemanagementasappsgrow,and5)theneedtokeepupwithitsrapidevolution.Thesefactorsshou

Reactischallengingforbeginnersduetoitssteeplearningcurveandparadigmshifttocomponent-basedarchitecture.1)Startwithofficialdocumentationforasolidfoundation.2)UnderstandJSXandhowtoembedJavaScriptwithinit.3)Learntousefunctionalcomponentswithhooksforstate

ThecorechallengeingeneratingstableanduniquekeysfordynamiclistsinReactisensuringconsistentidentifiersacrossre-rendersforefficientDOMupdates.1)Usenaturalkeyswhenpossible,astheyarereliableifuniqueandstable.2)Generatesynthetickeysbasedonmultipleattribute


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
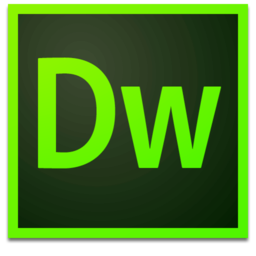
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
