In web design, carousel is a common visual effect used to display multiple pictures or content. HTML provides a variety of methods to implement carousel functions, and this article will introduce several of them.
1. Use CSS3 animation to implement carousel
CSS3 animation is a simple method to implement carousel. By setting animations, pictures or content can automatically loop. The specific implementation steps are as follows:
- Set the carousel image container in HTML, as shown below:
<div class="carousel"> <img src="/static/imghwm/default1.png" data-src="image1.jpg" class="lazy" alt=""> <img src="/static/imghwm/default1.png" data-src="image2.jpg" class="lazy" alt=""> <img src="/static/imghwm/default1.png" data-src="image3.jpg" class="lazy" alt=""> </div>
- Set the container style in CSS and set animation :
.carousel { position: relative; width: 100%; height: 500px; overflow: hidden; } .carousel img { position: absolute; top: 0; left: 0; opacity: 0; transition: opacity 1s ease-in-out; } .carousel img:first-child { opacity: 1; } .carousel img.active { opacity: 1; } @keyframes carousel { 0% { transform: translateX(0%); } 20% { transform: translateX(-100%); } 40% { transform: translateX(-200%); } 60% { transform: translateX(-300%); } 80%{ transform:translateX(-400%); } 100% { transform: translateX(0%); } }
- Set the carousel logic in JS:
let activeImage = 0; setInterval(function() { const images = document.querySelectorAll('.carousel img'); images[activeImage].classList.remove('active'); activeImage++; if (activeImage >= images.length) { activeImage = 0; } images[activeImage].classList.add('active'); }, 5000);
Through the above steps, the effect of the carousel image can be achieved.
2. Use JavaScript to implement carousels
JavaScript is also a common method to implement carousels. The specific implementation steps are as follows:
- Set the carousel image container in HTML, as shown below:
<div class="carousel"> <img src="/static/imghwm/default1.png" data-src="image1.jpg" class="lazy" alt=""> <img src="/static/imghwm/default1.png" data-src="image2.jpg" class="lazy" alt=""> <img src="/static/imghwm/default1.png" data-src="image3.jpg" class="lazy" alt=""> </div>
- Set the container style in CSS:
.carousel { position: relative; width: 100%; height: 500px; overflow: hidden; } .carousel img { position: absolute; top: 0; left: 0; opacity: 0; transition: opacity 1s ease-in-out; } .carousel img:first-child { opacity: 1; }
- Set the carousel logic in JS and add event monitoring:
let activeImage = 0; const images = document.querySelectorAll('.carousel img'); setInterval(function() { images[activeImage].classList.remove('active'); activeImage++; if (activeImage >= images.length) { activeImage = 0; } images[activeImage].classList.add('active'); }, 5000); document.addEventListener('DOMContentLoaded', function() { const next = document.querySelector('.carousel .next'); const prev = document.querySelector('.carousel .prev'); next.addEventListener('click', function() { images[activeImage].classList.remove('active'); activeImage++; if (activeImage >= images.length) { activeImage = 0; } images[activeImage].classList.add('active'); }); prev.addEventListener('click', function() { images[activeImage].classList.remove('active'); activeImage--; if (activeImage < 0) { activeImage = images.length - 1; } images[activeImage].classList.add('active'); }); });
Through the above steps, the carousel image has the effect of turning pages forward and backward. Achieved.
3. Use plug-ins to implement carousels
In addition to the above two methods, you can also use ready-made carousel plug-ins to achieve carousel effects. The following are several common carousel plug-ins:
- Slick Slider: A simple and easy-to-use responsive carousel plug-in that supports infinite sliding, adaptive, lazy loading, etc.
- Swiper: A mobile-first carousel plug-in with real hardware acceleration, multiple animation effects and other features.
- Owl Carousel: A feature-rich and highly customizable carousel plug-in that supports multiple layout forms, etc.
The above methods can be used to achieve the carousel effect. It is necessary to choose the appropriate method to implement based on actual needs and technical level.
The above is the detailed content of Implementation of html carousel. For more information, please follow other related articles on the PHP Chinese website!
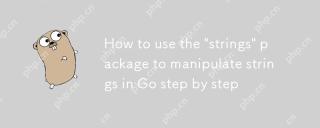
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
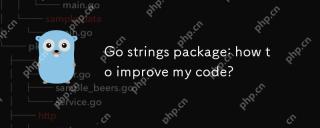
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
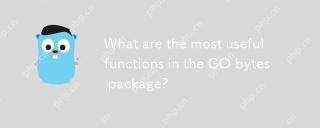
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
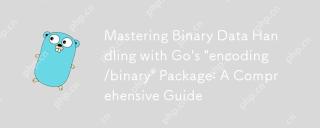
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
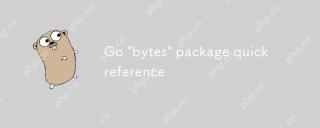
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
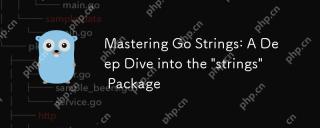
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
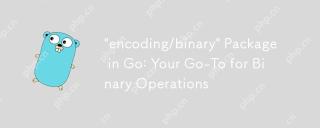
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
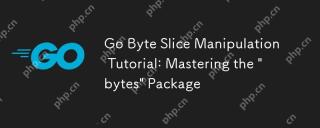
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
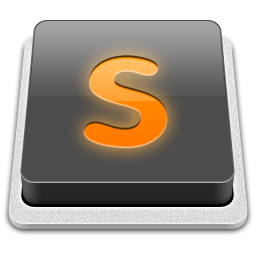
SublimeText3 Mac version
God-level code editing software (SublimeText3)
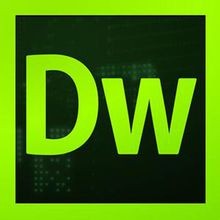
Dreamweaver CS6
Visual web development tools
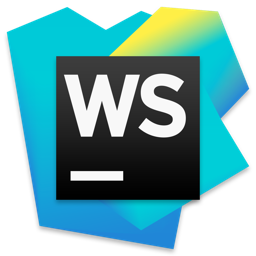
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
