In PHP, arrays are a very common data type that are often used to store and process large amounts of data. In web development, we usually need to display the data in the array onto an HTML page so that users can easily view and manipulate the data. This article will explain how to use PHP to iterate through an array and display it on an HTML page.
1. Basic method of traversing an array
Traversing an array refers to the process of accessing array elements in a certain order. In PHP, there are many ways to traverse arrays. Here are some commonly used methods.
- Use for loop to traverse
Using for loop is the most common way to traverse an array. Its basic syntax is as follows:
for ($i = 0; $i <p>Among them, $arr is the array to be traversed, and the count function is used to obtain the length of the array, that is, the number of elements. $i is a loop counter that traverses the array elements starting from 0 until the value of $i is equal to the array length. </p><p>In the loop body, you can use the array subscript to access the value of the array element, for example: </p><pre class="brush:php;toolbar:false">for ($i = 0; $i "; }
The above code will output the value of the array element in order, and add after each value newline character.
- Use foreach to traverse
Using foreach is a simpler way to traverse an array. The basic syntax is as follows:
foreach ($arr as $value) { //处理数组元素 }
where $arr is The array to be traversed, $value is the value of the array element currently traversed by the loop. foreach will automatically start traversing from the first element of the array until all elements have been traversed.
In the loop body, you can directly use the $value variable to access the value of the array element, for example:
foreach ($arr as $value) { echo $value . "<br>"; }
- Use a while loop to traverse
Use The while loop can also traverse the array. Its basic syntax is as follows:
$i = 0; while ($i <p>Similar to the for loop, the while loop requires a counter $i to control the number of loops. The difference is that the counter value $i needs to be manually updated in the loop body. Within the loop body, you can also use array subscripts to access the values of array elements. </p><p>2. Display the array data on the HTML page</p><p>After using PHP to traverse the array, we usually need to display the data in the array on the HTML page. To do this, we can use a combination of HTML tags and PHP scripts to generate dynamic HTML code. Two commonly used methods are introduced below. </p><ol><li>Use echo to output HTML code</li></ol><p>Using echo is the simplest and most commonly used method to output data to an HTML page. HTML tags and PHP scripts can be embedded in echo statements. For example, we can use the following code to output array elements to a table: </p><pre class="brush:php;toolbar:false">echo "
" . $value . " |
The above code first outputs a
- Use PHP to generate HTML code
In addition to using the echo statement to output HTML code, we can also use the PHP generator to generate dynamic HTML code. The PHP generator is a special PHP syntax that separates PHP code and HTML tags, making the code clearer and easier to maintain.
The following is a sample code that uses the PHP generator to generate a dynamic table:
The above code uses the HTML tag
tags during the traversal process, and output the values of array elements. Finally, endforeach is used to end the traversal, and the |
The advantage of using the PHP generator is that the code is more concise and easy to maintain, avoiding a large number of HTML tags and echo statements, making the code easier to write and modify. However, you also need to pay attention to the syntax specifications when using the PHP generator, especially the closing of the tags when switching between HTML tags and PHP scripts.
3. Summary
This article introduces two methods of PHP traversing arrays and displaying their data on HTML pages: using for loops, foreach loops and while loops to traverse arrays, and using echo statement or PHP generator to generate dynamic HTML code. Traversing arrays in PHP is a basic programming skill that can help us process and display data more flexibly and improve the efficiency of web development.
The above is the detailed content of How to traverse an array in php and display it to html. For more information, please follow other related articles on the PHP Chinese website!
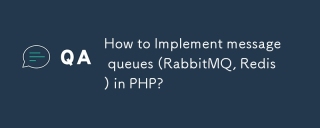
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
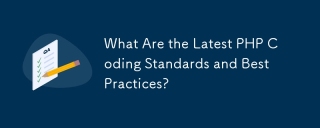
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
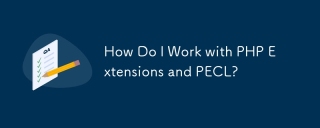
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
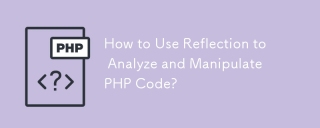
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
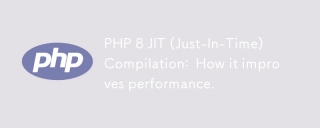
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
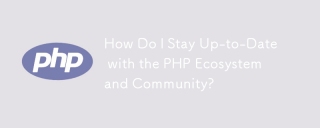
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
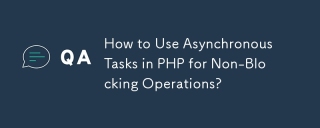
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
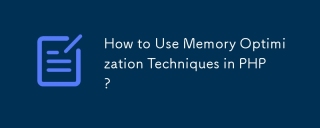
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
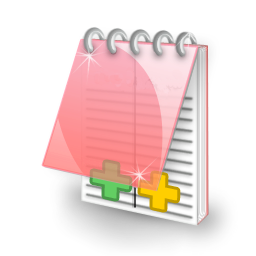
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
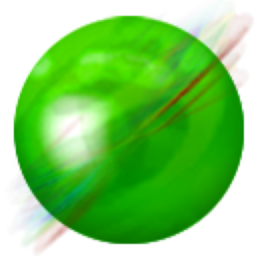
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
