PHP is a programming language widely used in Web development. It is often used to perform database queries and process data in Web applications. JSON is a universal format for data exchange. It stores data in an easy-to-read text format and is widely used in front-end data transfer. This article will introduce how to convert a PHP array into an array of JSON objects for use in web applications.
1. Convert PHP array to JSON object array
In PHP, we can use the json_encode function to convert the PHP array into a JSON format string. This conversion process is very simple. For example, we have a PHP associative array containing name and age:
$person = array( "name" => "Tom", "age" => 25, );
To convert this PHP array into a JSON array of objects, we simply use the following code:
$json = json_encode(array($person)); print_r($json);
will generate the following JSON Format string:
[{"name":"Tom","age":25}]
This JSON object array contains an object containing the "name" and "age" properties. Please note that our process of converting a PHP array to a JSON format string using the json_encode function is very simple and straightforward as this function is one of the PHP built-in functions.
Also, if we have multiple PHP arrays that need to be converted to JSON object arrays, we can just add them to an array and then pass the array to the json_encode function as a parameter of the function. For example:
$person1 = array( "name" => "Tom", "age" => 25, ); $person2 = array( "name" => "John", "age" => 30, ); $json = json_encode(array($person1, $person2)); print_r($json);
This will generate the following JSON format string:
[{"name":"Tom","age":25},{"name":"John","age":30}]
As you can see, in this example, we are adding two PHP arrays to the same array and then This array is passed as a parameter to the json_encode function.
2. Convert JSON object array to PHP array
After we convert the PHP array to a JSON object array, sometimes we need to convert the JSON object array back to an array in PHP format so that it can be used on the Web Access and use this data within the application.
In PHP, we can use the json_decode function to convert a JSON-formatted string into a PHP array. For example, we have an array of JSON objects containing information about two people:
[ {"name": "Tom", "age": 25}, {"name": "John", "age": 30} ]
To convert this array of JSON objects into a PHP array, we can do this:
$json = '[ {"name": "Tom", "age": 25}, {"name": "John", "age": 30} ]'; $people = json_decode($json, true); print_r($people);
will generate the following PHP array :
Array ( [0] => Array ( [name] => Tom [age] => 25 ) [1] => Array ( [name] => John [age] => 30 ) )
Please note that when we use the json_decode function, we set the second parameter to true to ensure that a PHP array is returned. If you don't set the second parameter to true, you will get a PHP object, not a PHP array.
Summary:
Converting PHP arrays to JSON object arrays is one of the important skills in web development. You can easily convert data between PHP and JSON by using the json_encode and json_decode functions. What you need to know is that if you need to convert an array of JSON objects to a PHP array, make sure to set the second parameter to true. This way, you will get an array in PHP format, which will simplify your workflow of accessing and using this data in your web application.
The above is the detailed content of Convert php array to json object array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
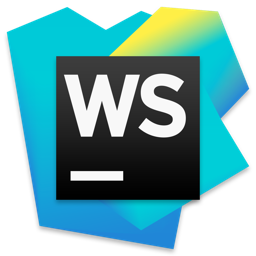
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
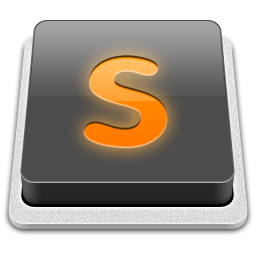
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
