PHP is a scripting language widely used in Web development. It can help us easily handle various types of data, including multi-dimensional arrays. In some advanced applications, we need to convert multi-dimensional arrays into strings for transmission, storage or debugging. In this article, we will introduce how to convert multidimensional array to string in PHP.
I. Definition of multi-dimensional arrays in PHP
In PHP, multi-dimensional arrays are nested from multiple arrays. For example:
$user_info = array( 'name' => '张三', 'age' => '30', 'address' => array( 'province' => '广东省', 'city' => '深圳市', 'district' => '南山区', ), 'hobbies' => array( 'reading', 'singing', 'swimming', ), );
In the above example, $user_info is an array containing text and array data. Among them, $user_info['address'] contains an associative array, and $user_info['hobbies'] contains an index array.
II. Convert PHP multi-dimensional array to string
When we need to convert PHP multi-dimensional array into string, we can use PHP's own function json_encode. This function converts data in JSON format (JSON: JavaScript Object Notation, a lightweight data exchange format) into a string, for example:
$user_info_string = json_encode($user_info); echo $user_info_string;
The above code will output the following content:
{"name":"张三","age":"30","address":{"province":"广东省","city":"深圳市","district":"南山区"},"hobbies":["reading","singing","swimming"]}
In this way, we convert the multidimensional array into a string represented in JSON format.
III. Convert JSON format string back to PHP multi-dimensional array
When we need to convert JSON format string back to PHP multi-dimensional array, we can use PHP's own function json_decode . This function parses JSON format data into a data type that PHP can recognize, for example:
$user_info_array = json_decode($user_info_string, true); print_r($user_info_array);
The above code will output the following content:
Array ( [name] => 张三 [age] => 30 [address] => Array ( [province] => 广东省 [city] => 深圳市 [district] => 南山区 ) [hobbies] => Array ( [0] => reading [1] => singing [2] => swimming ) )
In this way, we convert the JSON format characters The string is converted into a PHP multi-dimensional array, so that we can continue to process data easily.
IV. Other ways to convert PHP multidimensional arrays into strings
In addition to using the json_encode function to convert PHP multidimensional arrays into JSON format strings, there are other ways to convert PHP multidimensional arrays into strings. Convert to a string, for example, use the serialize function, for example:
$user_info_string = serialize($user_info); echo $user_info_string;
The above code will output the following:
a:4:{s:4:"name";s:6:"张三";s:3:"age";s:2:"30";s:7:"address";a:3:{s:8:"province";s:9:"广东省";s:4:"city";s:9:"深圳市";s:8:"district";s:9:"南山区";}s:7:"hobbies";a:3:{i:0;s:7:"reading";i:1;s:7:"singing";i:2;s:8:"swimming";}}
But the string generated by using the serialize function is more verbose than the string in JSON format. Not conducive to transmission and storage.
V. Summary
PHP Multidimensional array is a very common data type in Web development. When we need to convert the multidimensional array into a string, we can use PHP's own function json_encode , convert it into a string in JSON format, or you can use the serialize function to convert it into a string. When we need to convert a JSON format string back into a PHP multidimensional array, we can use the json_decode function to parse the string. The use of these functions can help us process multi-dimensional arrays conveniently and improve the efficiency of back-end development.
The above is the detailed content of Convert php multidimensional array to string. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
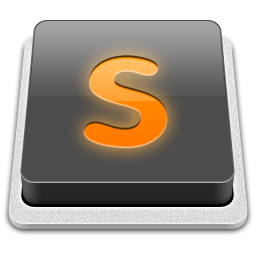
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
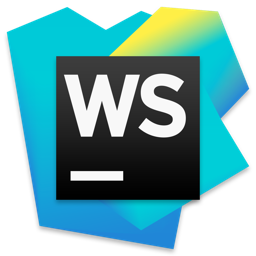
WebStorm Mac version
Useful JavaScript development tools
