In PHP, we can convert arrays to strings. Because sometimes when transmitting or storing data, it is necessary to convert the data from an array to a string format. Here are several ways to convert arrays into strings.
- implode() function
implode() function can concatenate all elements of an array into a string using specified symbols. The syntax of this function is as follows:
string implode ( string $glue , array $pieces )
Among them, the $glue parameter represents the string used to connect the array elements. The $pieces parameter is the array to be connected.
Sample code:
$arr = array('apple', 'banana', 'orange'); $str = implode(',', $arr); echo $str; // 输出: apple,banana,orange
In this example, we concatenate all elements of the array $arr into a string $str with commas.
- join() function
The functions of the join() function and the implode() function are completely consistent. It can also concatenate all elements of an array into a string using specified symbols. The syntax of this function is as follows:
string join ( string $glue , array $pieces )
The same as the implode() function, the $glue parameter represents the string used to connect the array elements. The $pieces parameter is the array to be connected.
Sample code:
$arr = array('apple', 'banana', 'orange'); $str = join(',', $arr); echo $str; // 输出: apple,banana,orange
This example also uses commas to connect all elements of the array $arr into a string $str.
- Using a foreach loop
We can use a foreach loop to traverse all the elements of the array, and then concatenate each element to finally form a string. Sample code:
$arr = array('apple', 'banana', 'orange'); $str = ''; foreach ($arr as $value) { $str .= $value . ','; } $str = rtrim($str, ','); echo $str; // 输出: apple,banana,orange
In this example, we iterate through all the elements of the array $arr, connect each element with a comma, and finally remove the comma at the end of the string to get the final result.
Through the above three methods, we can convert the array into a string and achieve our needs. In actual development, we can choose the right method to achieve our purpose according to the specific situation.
The above is the detailed content of Convert array to string in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
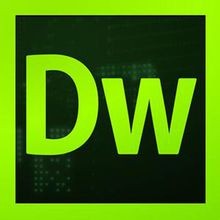
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
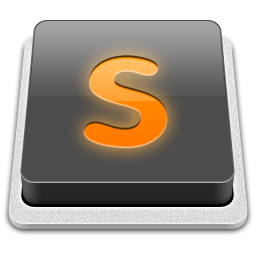
SublimeText3 Mac version
God-level code editing software (SublimeText3)
