PHP Password Change Page
We need to use passwords when performing many business operations. The issue of password confidentiality has always been a very important topic. When it comes to data security, passwords should be encrypted using the highest level of protection. But even if your password is impeccable, if you forget it, your data will become inaccessible. In this case, we need a password change tool to help us reset a new password to ensure that the data can be used in a timely manner.
In PHP, creating a password change page is relatively simple. The following are the main steps and implementation process.
Step 1 - Establish a database connection
The first step is to establish a database connection. Here we can use MySQL database. In PHP's MySQL function, we can use the mysqli_connect() function to connect to the MySQL database.
Sample code:
$dbhost = 'localhost'; //Database host name
$dbname = 'mydatabase'; //Database name
$dbuser = 'root' ; //Database username
$dbpass = ''; //Database password
$conn = mysqli_connect("$dbhost", "$dbuser", "$dbpass") or die ("Connect Error");
mysqli_select_db($conn,"$dbname") or die ("Cannot connect to the database");
If the connection fails, "Connection error" or " Cannot connect to database" and other prompt messages.
Step 2 - Create a password change form
We need to create a password change form in HTML.
Sample code:
at In this form, we define three input boxes to obtain the old password, new password and confirm password. What should be noted here is that we need to set the type attribute in the password input box to "password" so that the password can be hidden. Step 3 - Processing form dataProcessing form data is a very important step. In this step, we must verify whether the data entered by the user meets the specifications. We also need to determine whether the old password entered by the user is correct, and confirm whether the new password entered twice is the same. First, we need to verify whether the data is complete. In this example, all three of our input boxes are required. Therefore, we need to use the HTML5 required attribute to confirm that the user has entered the required fields. Sample code: This required attribute ensures that the input content in the form cannot be empty. Next, we need to get the old password entered by the user and encrypt it. The reason we want to encrypt the password is to save it to the database. In this case, we can use the md5() function in PHP. Sample code: $oldpassword = md5($_POST['oldpassword']);Next, we need to verify whether the old password entered by the user is correct, which requires Query the database. Sample code: $sql = "SELECT * FROM userdata WHERE password = '$oldpassword'";
$result = mysqli_query($conn, $sql) or die(mysqli_error ($conn));
//Old password verification successful
} else {
//Old password verification failed
}
$result = mysqli_query($conn, $sql) or die(mysqli_error($conn));
echo "Password changed successfully";
} else {
echo "Password changed Failed, please try again";
}
Finally, by combining all the codes together, we can create a complete PHP password change page.
Full code:
$dbhost = 'localhost'; //Database host name
$dbname = 'mydatabase'; //Database name
$dbuser = 'root'; //Database user name
$dbpass = ''; //Database password
$conn = mysqli_connect("$dbhost", "$dbuser", "$dbpass ") or die ("Connection error");
mysqli_select_db($conn,"$dbname") or die ("Cannot connect to database");
if(isset($_POST ['submit'])) {
$oldpassword = md5($_POST['oldpassword']);
$newpassword = md5($_POST['newpassword']);
$confirmpassword = md5( $_POST['confirmpassword']);
$id = $_POST['id'];
$sql = "SELECT * FROM userdata WHERE password = '$oldpassword'";
$ result = mysqli_query($conn, $sql) or die(mysqli_error($conn));
if(mysqli_num_rows($result) > 0) {
if($newpassword == $confirmpassword) { $sql = "UPDATE userdata SET password='$newpassword' WHERE id='$id'"; $result = mysqli_query($conn, $sql) or die(mysqli_error($conn)); if(mysqli_affected_rows($conn) > 0) { echo "密码修改成功"; } else { echo "密码修改失败,请重试"; } } else { echo "两次输入的新密码不一致,请重新输入"; }
} else {
echo "旧密码验证失败";
}
}
?>
Through the above steps, we can create a complete PHP password modification page to ensure data security.
The above is the detailed content of php password change page. For more information, please follow other related articles on the PHP Chinese website!
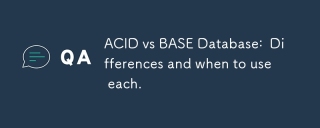
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
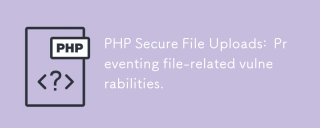
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
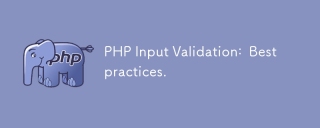
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
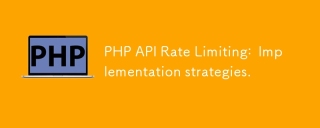
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
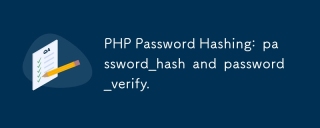
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
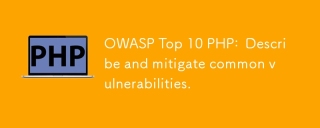
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
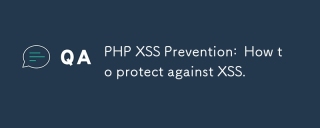
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
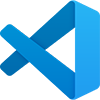
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.