In PHP development, we often encounter the need to convert JSON data into a string array. This article details how to implement this feature.
First, let’s take a look at the basic format of JSON. JSON is a data exchange format that consists of key-value pairs. Keys and values are separated by colons (:). Each key-value pair is separated by commas (,). All values must be characters. One of string, number, Boolean, array, object, and null.
For example, the following is a simple JSON format:
{
"name": "Tom", "age": 18, "gender": "male", "hobbies": ["Swimming", "Reading", "Traveling"]
}
Next, we will introduce how to convert the above JSON into characters string array. The steps to implement this function are as follows:
Step 1: Obtain JSON data
Usually, we will obtain JSON data from external data sources, such as from API interfaces. In PHP, we can get JSON data through the following code:
$json_data = file_get_contents("data.json");
The "data.json" here is a JSON file name, we can use the file_get_contents function to read the file and store it in the variable $json_data.
Step 2: Parse JSON data
Next, we need to parse the JSON data string into a PHP array or object. In PHP, we can use the json_decode() function to decode JSON data. The syntax of this function is as follows:
mixed json_decode(string $json, bool $associative = false, int $depth = 512, int $options = 0)
Among them, the $json parameter is Decoded JSON data string, the $associative parameter is used to specify whether the returned result is an associative array (default is an object), the $depth parameter is used to specify the depth of recursive decoding, and the $options parameter is used to specify decoding options.
For example, the following code converts a JSON string into an associative array:
$json_array = json_decode($json_data, true);
After decoding is complete, the $json_array variable Will contain the decoded associative array.
Step 3: Extract the required data
Now, we have parsed the JSON data into a PHP array or object. Next, we need to extract the required data from this array. Taking the above JSON as an example, we need to extract all elements in the "hobbies" array.
In PHP, we can use a foreach loop to iterate through an array and use the array_push function to add each element to a new array. The following code implements this step:
$hobbies_array = array();
foreach ($json_array['hobbies'] as $hobby) {
array_push($hobbies_array, $hobby);
}
In the above code, we use the array_push function to add each element to the $hobbies_array array.
Now, the $hobbies_array variable will contain all "hobbies" elements and the array can be returned as a string array.
Step 4: Output the string array
Finally, we need to output the $hobbies_array array as a string array. In PHP, we can use the implode function to concatenate array elements into a string. The following code converts the $hobbies_array array to a string array:
$hobbies_string = implode(",", $hobbies_array);
$hobbies_list = "[" . $hobbies_string . "]";
In the above code, we used the implode function to concatenate the $hobbies_array elements into a string and added commas between each element of the string. Finally, we stored the results in the $hobbies_list variable and added square brackets to form the complete string array.
So far, we have successfully converted JSON data into a string array. The complete code is as follows:
$json_data = file_get_contents("data.json");
$json_array = json_decode($json_data, true);
$hobbies_array = array();
foreach ($json_array['hobbies'] as $hobby) {
array_push($hobbies_array, $hobby);
}
$hobbies_string = implode(",", $hobbies_array);
$hobbies_list = " [" . $hobbies_string . "]";
echo $hobbies_list;
The above is a detailed introduction on how to convert JSON data into a string array. I hope this article can help PHP developers realize their project needs.
The above is the detailed content of json to string array php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
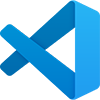
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
