PHP is a server-side scripting language that is widely used in Web server-side programming. In PHP, array is an important data structure. We can create and operate arrays in many ways. This article will introduce one of them: setting the array through key-value pairs.
1. Create an array
- Use [] or array() directly to create an empty array:
$a = []; $b = array();
- At the same time when creating To set array elements, you can use subscripts or key-value pairs:
$a = [1, 2, 3]; $b = ['name' => '张三', 'age' => 20];
- Array elements do not have to be set in order, and the subscripts can also be strings:
$a = [1, 'name' => '张三', 2, 'age' => 20];
2. Set the array elements
- Set by subscript:
$a = []; $a[0] = 1; $a[1] = 'hello'; $a[2] = ['a' => 1, 'b' => 2];
- Set by key-value pair:
$a = ['name' => '张三', 'age' => 20]; $a['address'] = '北京市朝阳区';
- Set through special key-value pairs:
$a = []; $a[] = 1; // 等价于 $a[0] = 1; $a[] = 'hello'; // 等价于 $a[1] = 'hello'; $a['name'] = '张三'; // 等价于 $a['name'] = '张三';
3. Access array elements
- Access through subscripts:
$a = [1, 'hello', ['a' => 1, 'b' => 2]]; echo $a[0]; // 输出1 echo $a[1]; // 输出hello echo $a[2]['a']; // 输出1
- Access through key-value pairs:
$a = ['name' => '张三', 'age' => 20]; echo $a['name']; // 输出张三 echo $a['age']; // 输出20
4. Traverse the array elements
- Use a for loop to traverse:
$a = [1, 2, 3]; for ($i = 0; $i <ol start="2"><li>Use a foreach loop to traverse: </li></ol><pre class="brush:php;toolbar:false">$a = ['name' => '张三', 'age' => 20]; foreach ($a as $key => $value) { echo $key, ': ', $value, PHP_EOL; // 输出:name: 张三,age: 20 }
- Use a while loop to traverse:
$a = [1, 2, 3]; while (list($key, $value) = each($a)) { echo $key, ': ', $value, PHP_EOL; // 输出:0: 1,1: 2,2: 3 }
The above is the relevant content of setting the array through key-value pairs. I hope to be helpful. PHP provides a variety of ways to create and operate arrays. Only by mastering them can you better write efficient PHP programs.
The above is the detailed content of php set array mode. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
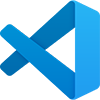
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
