1. Arithmetic operators
1. Introduction
In Java, the arithmetic operators , -, *, /, and % represent addition, subtraction, multiplication, division, and modulo respectively.
2. Application
There are three types in java: 1. Normal operation. 2. Use as positive and negative. 3. As a connector (when data of any data type is connected to a string, that number is the connector)
Example:
package com; public class liu { public static void main(String[] args) { //实例一:作为正常运算 int c = 1; int d = 2; int e = c + d; System.out.println(e) //最后结果为3; //实例二:作为连接符 //已知有两个变量a和b,值分别为1和2,在控制台上输出a + b = 3, 1 + 2 = 3 int a = 1; int b = 2; System.out.println(a + " + " + b + " = " + (a + b)); //控制台显示结果为:a + b = 3。 //注意此时的"+","="作为字符串,而(a + b)则进行运算。 } }
-
in java There are two types: 1. Normal operation. 2. Use as positive and negative.
Example:
package com; public class liu { public static void main(String[] args) { int a = 1; int b = 3; int c = b-a; System.out.println(c); //运算结果:2。 } }
*
Example:
package com; public class liu { public static void main(String[] args) { int i = 4; int j = 2; int a = i*j; System.out.println(a);//8 } }
/
When the two numbers involved in/operating are both integers , the result is an integer, otherwise it is a floating point number.
Example:
package com; public class liu { public static void main(String[] args) { //实例1:作为整数运算 int i = 4; int j = 2; int a = i / j; System.out.println(a);//2 //实例2:作为浮点运算 int i = 5; double j = 2; double a = i / j; System.out.println(a);//2.5 } }
%
The remainder operation of an integer (sometimes called modulo) is represented by %.
package com; public class liu { public static void main(String[] args) { int i = 2; int j = 3; System.out.println(i % j); //0 int a = 4; int b = 2; System.out.println(a % b); //2 } }
2. Auto-increment and auto-decrement operators
Used alone: No matter before or after, the result is the same. See Example 1 for details.
Participate in the operation: First: first add 1 to itself, and then participate in the operation, see Example 2 for details; Behind: first participate in the operation, and then add 1 to itself, see Example 3 for details.
Example:
package com; public class liu { public static void main(String[] args) { //实例1:单独运算。 int i = 1; i++; // i = i + 1 ++i; System.out.println(i);//结果都等于2。 //实例2:++在前。 int i = 1; int j = ++i; System.out.println(j);//2 System.out.println(i);//2 //实例3:++在后。 int i = 1; int j = i++; System.out.println(j);//1 System.out.println(i);//2 } }
–
Used alone: No matter whether – is in front or behind, the result is the same. See Example 1 for details.
Participate in the operation: – In the front: first decrement itself by 1, and then participate in the operation, see Example 2 for details; – In the back: first participate in the operation, and then decrement itself by 1, see Example 3 for details.
package com; public class liu { public static void main(String[] args) { //实例1:单独运算。 int i = 2; i--; // i = i - 1 --i; System.out.println(i);//结果都等于1。 //实例2:--在前。 int i = 1; int j = --i; System.out.println(j);//1 System.out.println(i);//1 //实例3:--在后。 int i = 1; int j = i--; System.out.println(j);//2 System.out.println(i);//1 } }
3. Assignment operator
You can use binary operators in assignment, and the form is very simple: x = 4 is equivalent to x = x 4.
Common assignment operators are: =, =, -=, *=, /=, %=.
The following takes = as an example:
package com; public class liu { public static void main(String[] args) { int i = 1; i += 2; // i = i + 2 System.out.println(i); //输出结果3 byte b = 1; b += 2; // b = (byte)(b + 2) System.out.println(b); } }
4. Relational operators
The result of the relational operator must be boolean type data, which means that it is either true , or false
Common relational operators: >, =,
Example:
package com; public class liu { public static void main(String[] args) { int i = 3; int j = 2; System.out.println(i > j);//true System.out.println(i < j);//false System.out.println(i >= j);//true System.out.println(i <= j);//false System.out.println(i != j);//true System.out.println(i == j);//false // ==是比较两边的大小,=是赋值。 } }
5. Logical operators
The data types of the data on the left and right sides are all boolean type, and the results are all boolean type.
&(Single AND)
As long as one of the two sides is false, the result will be false
Example:
package com; public class Demo12 { public static void main(String[] args) { System.out.println(true & true); System.out.println(true & false); //false System.out.println(false & false); System.out.println(false & true); //false } }
| (Single Or)
As long as one of the two sides is true, the result is true.
Example:
package com; public class Demo12 { public static void main(String[] args) { System.out.println(true | true); System.out.println(true | false); System.out.println(false | false); //false System.out.println(false | true); } }
^(XOR)
If both sides are the same, it will be false, and if both sides are different, it will be true.
Example:
package com; public class Demo12 { public static void main(String[] args) { System.out.println(true ^ true); //false System.out.println(true ^ false); System.out.println(false ^ false); //false System.out.println(false ^ true); } }
! (Non)
is directly the opposite.
Example:
package com; public class Demo12 { public static void main(String[] args) { System.out.println(!true); //false System.out.println(!false); //true System.out.println(!!true); //true System.out.println(!!!true); //false } }
&&(Double AND)
As long as one of the two sides is false, the result will be false.
Example:
package com; public class Demo12 { public static void main(String[] args) { System.out.println(true && true); System.out.println(true && false); //false System.out.println(false && false); //false System.out.println(false && true); //false } }
What is the difference between & and &&?
&&: If the left side is false, the right side will no longer be executed, and the result will be false.
&: If the left side is false, the right side will still be executed, and the result will be false.
|| (Double OR)
As long as one of the two sides is true, the result is true.
Example:
package com; public class Demo12 { public static void main(String[] args) { System.out.println(true || true); //true System.out.println(true || false); //true System.out.println(false || false); //false System.out.println(false || true); //true } }
What is the difference between | and ||?
||: If the left side is true, the right side will no longer be executed, and the result will be true.
|: If the left side is true, the right side will still be executed, and the result will be true.
6. Bitwise operators
The data on both sides are numbers, and the result is also a number.
Common bitwise operators: & (AND bit operation), | (OR bit operation), ^ (XOR bit operation), ~ (bitwise negation), >> (right shift operation), >> (unsigned right shift).
Example:
package com; public class Demo01 { public static void main(String[] args) { System.out.println(3 & 2); //2 System.out.println(3 | 2); //3 System.out.println(3 ^ 2); //1 System.out.println(~3);//-4 System.out.println(3 >> 2);//0 System.out.println(3 << 2);//12 System.out.println(3 >>> 2);//0 System.out.println(-3 >> 2);//-1 System.out.println(-3 >>> 2); } }
>> What is the difference between >>>?
>>: If the data is a negative number, the leftmost sign bit is 1. After the right shift, 1 must be added to the left.
If the data is a positive number, the leftmost sign bit is 0. After the right shift, 0 must be added to the left.
>>>: Regardless of whether the data is positive or negative, after the right shift, 0 is added to the left.
7. Ternary operator
Format: Conditional expression? Expression 1 : Expression 2; Equivalent to x > y ? x : y
Conditional expression The result of the expression must be of type boolean
Execution process:
If the conditional expression is true, expression 1 will be executed, and expression 2 will not be executed
If the condition If the expression is false, expression 2 will be executed and expression 1 will not be executed
Example:
package com; public class Demo02 { public static void main(String[] args) { //获取两个数的较大值 int i = 2; int j = 3; //第一种: int max = i > j ? i : j; System.out.println(max); //3 //第二种: System.out.println(i > j ? i : j); //表达式1和表达式2既然会得到一个结果,如果传递给一个变量去接收,该变量的数据类型应该和表达式1和表达式2的结果的数据类型匹配。 //浮点型: double d = 3 > 2 ? 1 : 2; System.out.println(d); //输出:1.0 //字符型: char c1 = 3 > 2 ? 97:98; System.out.println(c1); //输出:a } }
The above is the detailed content of Analysis of application examples of various operators in Java. For more information, please follow other related articles on the PHP Chinese website!
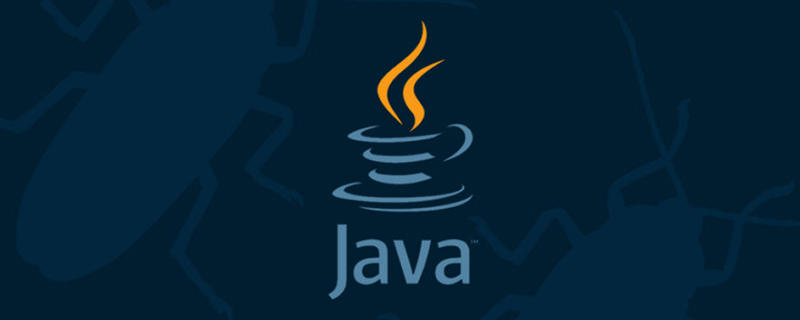
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
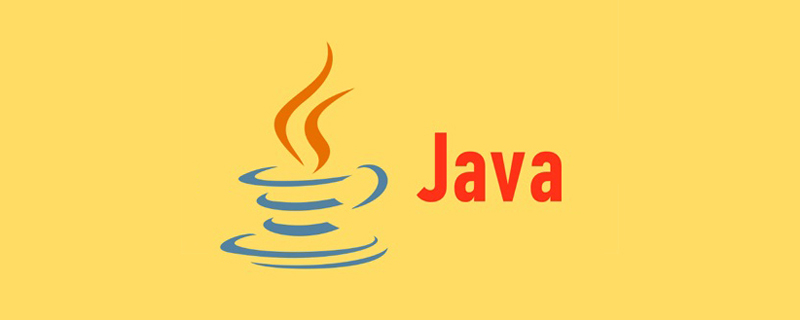
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
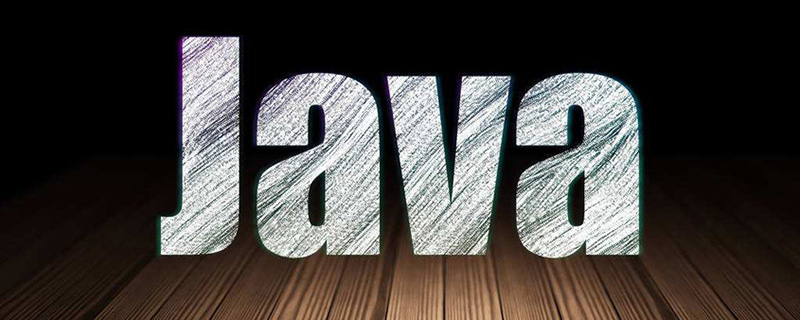
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
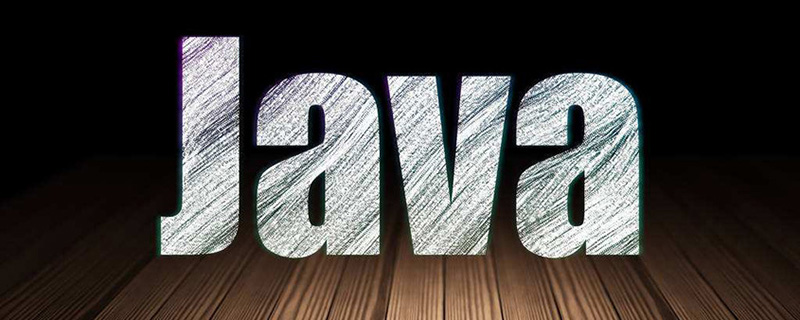
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
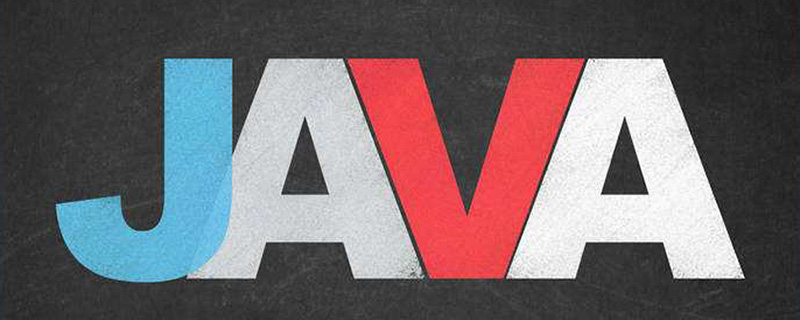
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
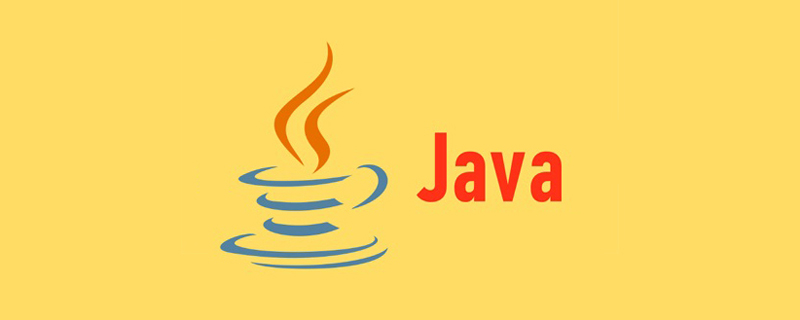
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
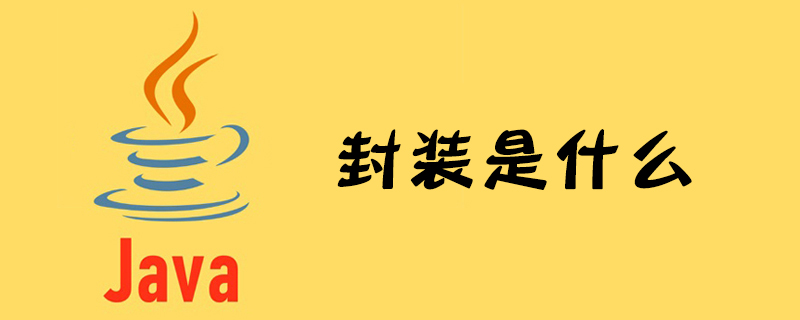
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
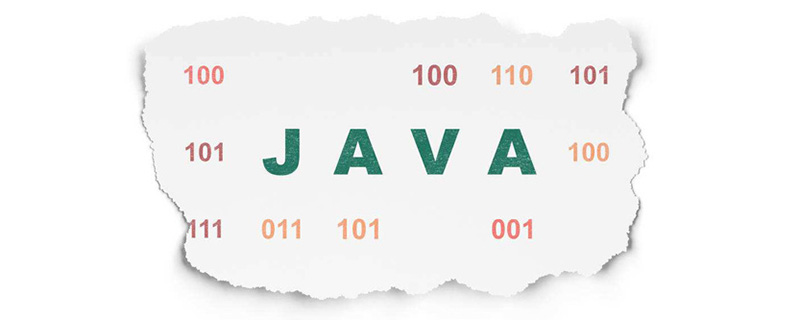
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
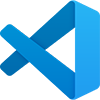
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
