What is the method for Java to automatically generate trend comparison data?
Background
Pairwise trend comparison between data is a very common application scenario in data analysis applications, as shown below:
Mock test Batch | Class | Student | Chinese | Mathematics | English |
---|---|---|---|---|---|
Class Three Years | 张小明 | 130 | 145 | 133 | |
三级一 | 王二小 | 128 | 138 | 140 | |
Class Three Three Years | Xie Chunhua | 136 | 142 | 139 | |
Three-Year Class | Zhang Xiaoming | 132 | 140 | 128 | |
Class Three Three Years | 王二小 | 125 | 146 | 142 | |
Class Three Three Years | Xie Chunhua | 138 | 143 | 140 | |
Three-Year Class | Zhang Xiaoming | 135 | 138 | 120 | |
三级一级 | 王二小 | 123 | 145 | 138 | |
Class Three Three Years | Xie Chunhua | 136 | 140 | 142 |
Class | Student | Chinese | Mathematics | English | |
---|---|---|---|---|---|
Three-Year Class | Zhang Xiaoming | -2 | 5 | 5 | |
三级一级 | 王二小 | 3 | -8 | #-2 | |
Class Three Three Years | Xie Chunhua | -2 | -1 | -1 | ##Comparison between 202301 and 202212 |
张小明 | -3 | 2 | 8 | Comparison between 202301 and 202212 | |
王二小 | 2 | 1 | 4 | 202301 and 202212 Comparison | |
Xie Chunhua | 2 | 3 | -2 |
public class TrendCompare {
/**
* 主体的字段列表(如三年一班的张小明,那么主体字段列表为 班级 + 学生姓名)
*/
private String[] subjectFields;
/**
* 在某个字段的(介词) 如三年一班张晓明在不同考试批次的成绩对比结果
*/
private String atField;
/**
* 参与趋势比较的字段集合
*/
private String[] compareFields;
/**
* 赋值映射集合:给结果数据中指定的key设置指定的值
*/
private Map<String, String> assignValMap;
public String[] subjectFields() {
return this.subjectFields;
}
public TrendCompare subjectFields(String... fields) {
this.subjectFields = fields;
return this;
}
public String atField() {
return this.atField;
}
public TrendCompare atField(String field) {
this.atField = field;
return this;
}
public String[] compareFields() {
return this.compareFields;
}
public TrendCompare compareFields(String... fields) {
this.compareFields = fields;
return this;
}
/**
* 赋值操作
*
* @param field
* @param valueEL 值表达式
* @return
*/
public TrendCompare assignVal(String field, String valueEL) {
if (assignValMap == null) {
assignValMap = new HashMap<>();
}
assignValMap.put(field, valueEL);
return this;
}
public Map<String, String> assignValMap() {
return this.assignValMap;
}
}
This class defines the following attributes:
The subject's Field list- Preposition field
- Compared field list
- For example: The progress and decline of each student
examination batcheseach subjectThe results of mapping the above requirements to the defined class are as follows :
Subject field list (- Class, student
- )
Preposition field (
Exam batch - )
Comparison field list (various subjects:
Chinese, mathematics, English - )
Trend ratio For the execution class
public static <T> List<T> compare(List<T> dataList, TrendCompare trendCompare) {
Map<String, List<T>> groupMap = group(dataList, null, trendCompare.subjectFields());
List<T> resultList = new ArrayList<>();
for (List<T> groupDataList : groupMap.values()) {
List<T> diffValueList = new ArrayList<>();
int size = groupDataList.size();
if (size > 1) {
for (int i = 0; i < size - 1; i++) {
//数据之间两两比较 diffValue = minuend - subtrahend
T minuend = groupDataList.get(i);
T subtrahend = groupDataList.get(i + 1);
T diffValue = minus(trendCompare.compareFields(), minuend, subtrahend);
//设置主体信息
if (trendCompare.subjectFields() != null) {
for (String subjectField : trendCompare.subjectFields()) {
setFieldValue(diffValue, subjectField, getFieldValue(minuend, subjectField));
}
}
//设置介词字段信息
String atField = trendCompare.atField();
if (StringUtils.isNotEmpty(atField)) {
setFieldValue(diffValue, atField, getFieldValue(minuend, atField) + "与" + getFieldValue(subtrahend, atField) + "对比增减");
}
diffValueList.add(diffValue);
}
}
if (diffValueList.size() > 0) {
T firstData = groupDataList.get(0);
Map<String, Object> valMap = new HashMap<>();
//指定的赋值集合进行赋值
if (trendCompare.assignValMap() != null) {
for (Map.Entry<String, String> stringStringEntry : trendCompare.assignValMap().entrySet()) {
String field = stringStringEntry.getKey();
if (!StringUtils.equalsAny(field, trendCompare.compareFields())) {
String valueEL = stringStringEntry.getValue();
valMap.put(field, executeSpEL(valueEL, firstData));
}
}
}
for (Map.Entry<String, Object> entry : valMap.entrySet()) {
for (T diffValue : diffValueList) {
setFieldValue(diffValue, entry.getKey(), entry.getValue());
}
}
}
resultList.addAll(diffValueList);
}
return resultList;
}
As you can see, this method requires the incoming
data collection - Trend comparison defines
- two parameters, and finally returns the result set after trend comparison.
- After grouping, within the group The data is compared pairwise and the comparison result is finally returned.
- Use case
Define a student class:
public class Student { private String batch; private String banji; private String studentNo; private String name; private String sex; private Double yuwen; private Double math; private Double english; private Double physics; //extra private String maxScoreName1; public Student(String batch, String banji, String studentNo, String name, String sex, Double yuwen, Double math, Double english, Double physics) { this.batch = batch; this.banji = banji; this.studentNo = studentNo; this.name = name; this.sex = sex; this.yuwen = yuwen; this.math = math; this.english = english; this.physics = physics; } }We write a method to return the above data:
public List<Student> getDataList() { List<Student> dataList = new ArrayList<>(); dataList.add(new Student("202302", "三年一班", "20001001", "张小明", "男", 130.0, 145.0, 133.0, 92.0)); dataList.add(new Student("202302", "三年一班", "20001002", "王二小", "男", 128.0, 138.0, 140.0, 98.0)); dataList.add(new Student("202302", "三年一班", "20001003", "谢春花", "女", 136.0, 142.0, 139.0, 95.0)); dataList.add(new Student("202302", "三年二班", "20002001", "冯世杰", "男", 129.0, 144.0, 138.0, 96.0)); dataList.add(new Student("202302", "三年二班", "20002002", "马功成", "男", 130.0, 132.0, 133.0, 98.0)); dataList.add(new Student("202302", "三年二班", "20002003", "魏翩翩", "女", 136.0, 142.0, 137.0, 92.0)); dataList.add(new Student("202301", "三年一班", "20001001", "张小明", "男", 132.0, 142.0, 134.0, 92.0)); dataList.add(new Student("202301", "三年一班", "20001002", "王二小", "男", 126.0, 136.0, 135.0, 94.0)); dataList.add(new Student("202301", "三年一班", "20001003", "谢春花", "女", 136.0, 145.0, 139.0, 95.0)); dataList.add(new Student("202301", "三年二班", "20002001", "冯世杰", "男", 124.0, 143.0, 148.0, 90.0)); dataList.add(new Student("202301", "三年二班", "20002002", "马功成", "男", 140.0, 133.0, 138.0, 90.0)); dataList.add(new Student("202301", "三年二班", "20002003", "魏翩翩", "女", 126.0, 136.0, 135.0, 92.0)); return dataList; }Trend comparison definition and execution comparison:
List<Student> dataList = getDataList(); TrendCompare trendCompare = new TrendCompare() .subjectFields("banji", "name") .atField("batch") .compareFields("yuwen", "math", "english") //.assignVal("batch", "'环比增减'") ; List<Student> resultList = DataProcessUtil.compare(dataList, trendCompare); for (Student result : resultList) { System.out.println(JSON.toJSONString(result)); }The results are as follows:
{"banji":"三年一班","batch":"202302与202301对比增减","english":-1.0,"math":3.0,"name":"张小明","yuwen":-2.0} {"banji":"三年一班","batch":"202302与202301对比增减","english":5.0,"math":2.0,"name":"王二小","yuwen":2.0} {"banji":"三年一班","batch":"202302与202301对比增减","english":0.0,"math":-3.0,"name":"谢春花","yuwen":0.0} {"banji":"三年二班","batch":"202302与202301对比增减","english":-10.0,"math":1.0,"name":"冯世杰","yuwen":5.0} {"banji":"三年二班","batch":"202302与202301对比增减","english":-5.0,"math":-1.0,"name":"马功成","yuwen":-10.0} {"banji":"三年二班","batch":"202302与202301对比增减","english":2.0,"math":6.0,"name":"魏翩翩","yuwen":10.0}
The above is the detailed content of What is the method for Java to automatically generate trend comparison data?. For more information, please follow other related articles on the PHP Chinese website!
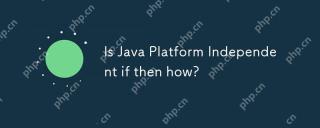
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
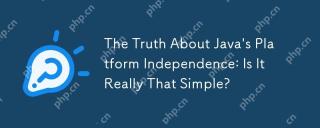
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
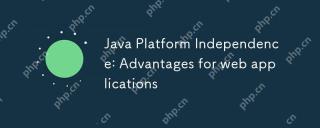
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
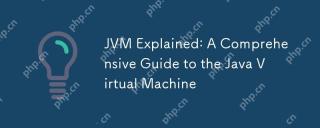
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
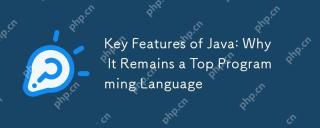
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
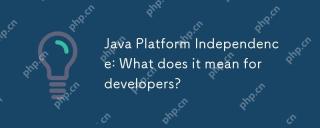
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
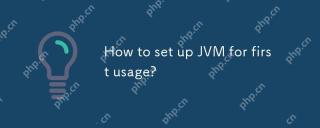
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
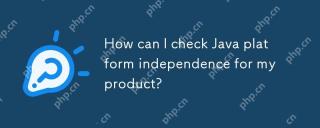
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
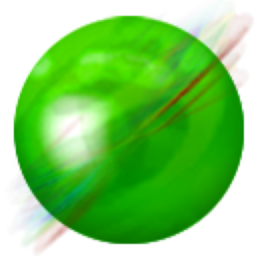
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
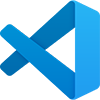
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
