linspace function in python numpy
numpy provides the linspace function (sometimes also called np.linspace) which is a tool for creating numerical sequences in python. Similar to the Numpy arange function, generates a uniformly distributed sequence of values with a structure similar to a Numpy array. Although there are some differences between the two, most people prefer to use the linspace function. It is easy to understand, but we need to learn how to use it.
In this article we learn the linspace function and other syntax, and explain the specific parameters through examples. Finally, I would like to mention the difference between np.linspace and np.arange.
1. Quickly understand
Create a numerical sequence by defining uniform intervals. In fact, you need to specify the starting point and ending end of the interval, and specify the total number of separated values (including the starting point and ending point); the final function returns a numerical sequence of uniform distribution of the interval class. Please see the example:
np.linspace(start = 0, stop = 100, num = 5)
The code generates a NumPy array (ndarray object), the result is as follows: array([ 0., 25., 50., 75., 100.])
As shown in the picture:
#Let us explain that the Numpy linspace function generates uniformly distributed values according to the defined interval. We specify the interval using the start and stop parameters, here we set them to 0 and 100, and specify that 5 observations are produced within the range, so the function generates 5 evenly distributed elements. The first one is 0, the last one is 100, and the other three are distributed between 0 and 100.
Let’s take a closer look at the parameters of the linspace function so that you can understand its mechanism more clearly.
2. Linspace function syntax
The syntax of linspace is very simple and direct. As shown in the figure below, the first is the function name, and the corresponding code is np.linspace (assuming you have imported NumPy as np).
Figure 2
The above figure has three parameters, which are the three most frequently used parameters. There are other optional parameters, whose parameters are discussed below.
In order to understand the parameters, let's look at the diagram again:
start
start The starting point of the parameter value range. If set to 0, the first number of the result is 0. This parameter must be provided.
stop
stop The end point of the parameter value range. Usually it is the last value of the result, but if endpoint = False is modified, the value will not be included in the result (as will be explained in the following examples).
num (Optional) The
num parameter controls how many elements there are in the result. If num=5, the number of output arrays is 5. This parameter is optional, and the default is 50.
endpoint (optional) The
endpoint parameter determines the end value (stop parameter) is included in the result array. If endpoint = True, the end value is included in the result, otherwise it is not. The default is True.
dtype (Optional)
Like other NumPy, the dtype parameter in np.linspace determines the data type of the output array. If not specified, Python infers the data type based on other parameter values. It can be specified explicitly if needed, and the parameter value can be any data type supported by NumPy and Python.
We do not need to use all parameters every time, if the default values can meet our needs. Generally, start, stop, and num are more commonly used than endpoint and dtype.
Positional parameters vs named parameters
There is no need to display the specified parameter name when actually calling, you can directly match it through the parameter position:
np.linspace(0, 100, 5)
The above code and the previous The function of the example is the same: np.linspace(start = 0, stop = 100, num = 5)
.
The former uses position matching, and the latter uses name matching. Position matching makes the code simpler, and name matching makes the code more readable. In practical applications, we encourage using name matching to call functions.
3. Example
Let’s learn the meaning of each parameter through examples.
3.1 A numerical sequence from 0 to 1 with an interval of 0.1
np.linspace(start = 0, stop = 1, num = 11)
The output result is:
array([ 0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1. ])
Useful when you need percentage scenarios.
3.2 A numerical sequence from 0 to 100 with an interval of 10
np.linspace(start = 0, stop = 100, num = 11)
The output result is:
array([ 0., 10., 20., 30., 40., 50., 60., 70., 80., 90., 100.])
This example is the same as before, but it is commonly used in practical applications.
3.3 Using the endpoint parameter
As mentioned earlier, the endpoint parameter determines whether the end value is included in the result array. The default is True, which means it is included in the result, otherwise it is not included. Please see the example:
np.linspace(start = 1, stop = 5, num = 4, endpoint = False)
Because endpoint = False, 5 is not included in the result. The result is 1 to 4.
array([ 1., 2., 3., 4.])
Personally, I think this parameter is not direct enough and is generally not used.
3.4 手动指定数据类型
默认linspace根据其他参数类型推断数据类型,很多时候,输出结果为float类型。如果需要指定数据类型,可以通过dtype设置。该参数很直接,除了linspace其他函数也一样,如:np.array,np.arange等。示例:
np.linspace(start = 0, stop = 100, num = 5, dtype = int)
这里dtype为int,结果为int类型,而不是float类型。
The above is the detailed content of How to use the linspace function in python numpy. For more information, please follow other related articles on the PHP Chinese website!
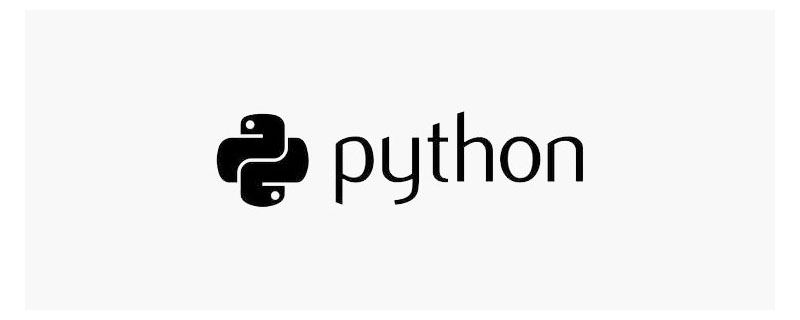
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
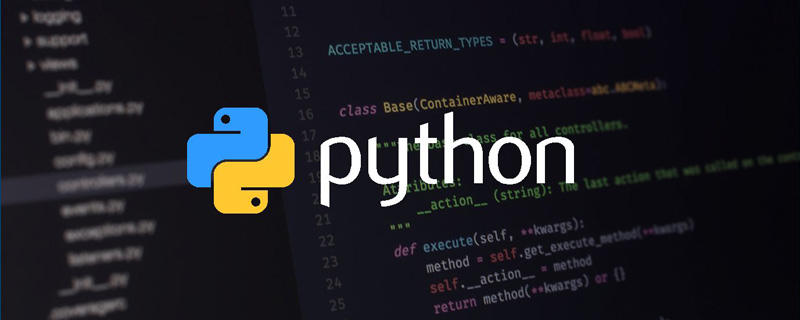
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
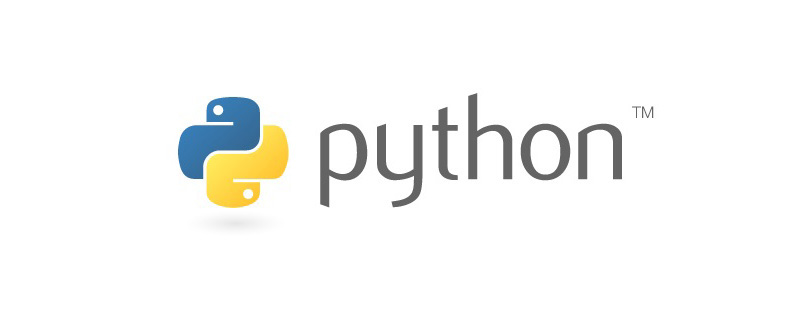
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
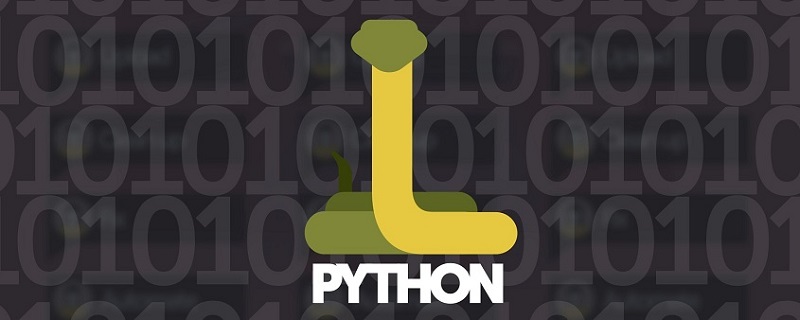
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
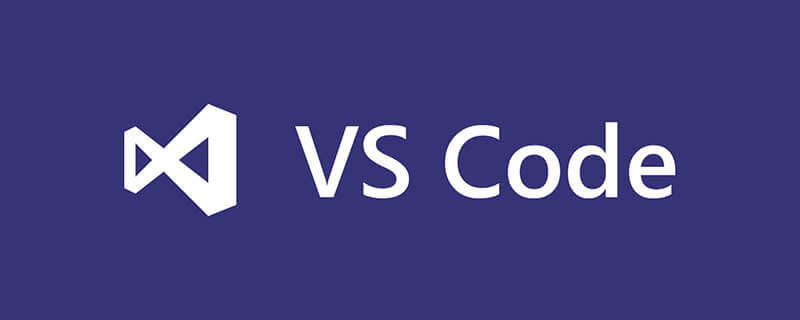
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
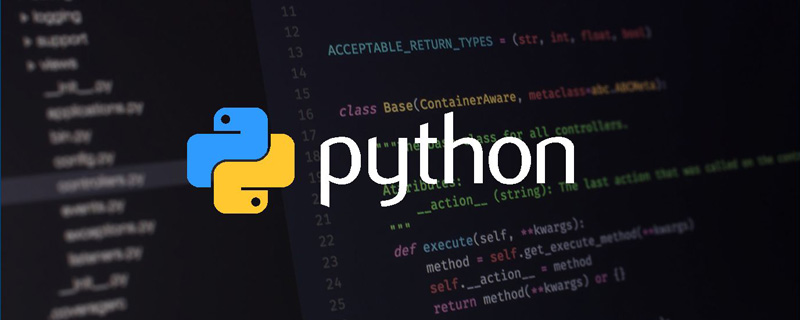
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
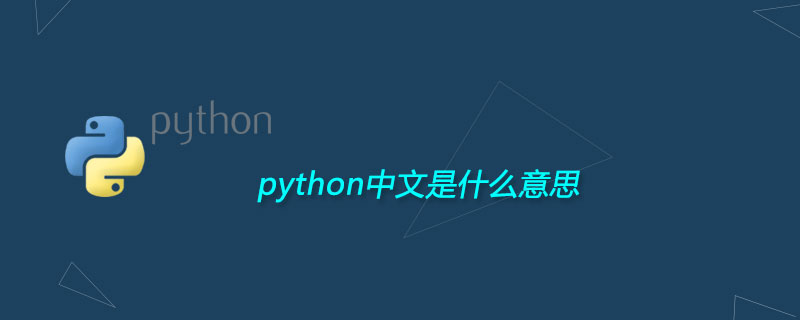
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
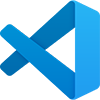
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.