1. Description of exceptions
An unexpected event occurs when a program is running, which prevents the program from executing normally as expected by the programmer. This is an exception. When an exception occurs, the program is left to fend for itself and exits immediately. In Java, that is, errors that occur during Java compilation or operation or during operation.
Java provides a better solution: exception handling mechanism.
The exception handling mechanism allows the program to handle exceptions in a targeted manner according to the preset exception handling logic of the code when an exception occurs, so that the program can return to normal and continue execution as much as possible, while maintaining the clarity of the code. .
Exceptions in Java can be caused when statements in a function are executed, or they can be thrown manually by programmers through the throw statement. As long as an exception occurs in a Java program, a The exception object of the corresponding type is used to encapsulate the exception, and the JRE will try to find an exception handler to handle the exception.
Exception refers to an abnormal situation that occurs during runtime.
Abnormal situations are described and objects encapsulated in the form of classes in java.
A class that describes abnormal situations becomes an exception class.
Separate normal code and problem handling code to improve readability.
In fact, exceptions are Java encapsulating problems into objects through object-oriented thinking, and using exception classes to describe them.
2. Exception system
Two major categories:
hrowable: exceptions that can be thrown, whether it is error Or exception, it should be thrown when a problem occurs, so that the caller can know and handle it.
The characteristic of this system is that Throwable and all its subclasses are throwable.
What exactly does throwability mean? How to reflect throwability?
is reflected by two keywords.
throws throw All classes and objects that can be operated by these two keywords are throwable.
Subcategory 1 Generally unprocessable. ————Error
Characteristics: It is a serious problem thrown by jvm. When this kind of problem occurs, it is generally not dealt with in a targeted manner, and the program is directly modified.
Subclass 2) can handle it. ——Exception, the problem is thrown to the caller, whoever throws it to whom.
Features: The suffix names of subclasses are all suffixed with the name of their parent class, which is very readable!
Example: For example, customize an exception with a negative index and encapsulate it into an object using object-oriented thinking.
Note: If a class is called an exception class, it must inherit the exception class. Because only subclasses of the exception system are throwable.
class FuShuIndex extends Exception{ //构造函数 和类名一样 FuShuIndex(){ } //定义一个带参数的构造函数 FuShuIndex(String msg){ //调用Exception中的带参数异常函数 super(msg); } } 主函数 throws FuShuIndex:{ int[] arr = new int[3]; method(arr,-7); } public static int method(int[] arr,int index) throws arrIndexexception { if (index<h3 id="Exception-classification"> 3. Exception classification: </h3><p>Exceptions detected during compilation are also Exception and its subclasses, except for the special subclass RuntimeException, which is compiled without processing fail! </p>
Once this kind of problem occurs, we hope to detect it at compile time so that this kind of problem can be dealt with accordingly, so that such problems can be dealt with in a targeted manner.
Undetected exceptions during compilation (runtime exceptions): RuntimeException and its subclasses
can be processed or not, and the compilation can pass , it will be detected at runtime!
The occurrence of this kind of problem is that the function cannot be continued and the operation cannot be run. It is mostly caused by the call or the change of the internal state. This kind of problem is generally not dealt with, and is directly compiled and passed. At runtime, the program is forced to stop by the caller, allowing the caller to correct the code.
The difference between throws and throw:
throws is used on functions ————Statement
throw is used within a function, and multiple ones can be thrown, separated by commas. ——Throws
throws throws an exception class, and multiple exceptions can be thrown.
throw throws an exception object.
4. Capture form of exception handling
This is a way to handle exceptions in a targeted manner.
Format:
try{ //需要被检测异常的代码 } catch(异常类 变量)//该变量用于接收发生的异常对象{ //处理异常代码 } finally{ //一定会被执行的代码 }
Example
class FuShuIndex extends Exception{ //构造函数 和类名一样 FuShuIndex(){ } //定义一个带参数的构造函数 FuShuIndex(String msg){ //调用Exception中的带参数异常函数 super(msg); } }
Main function: No need for throws, let’s catch the exception ourselves
{ int[] arr = new int[3]; try{ method(arr,-7); }catch(arrIndexexception a){ a.printStackTrace();//jvm默认的异常处理机制就是调用异常对象的这个方法。 System.out.println("数组的角标异常!!!");//自定义捕获后打印的信息 System.out.println(a.toString());//打印该异常对象的信息 System.out.println(a.getMessage());//获取我们自定义抛出所定义的信息 } } public static int method(int[] arr,int index) throws arrIndexexception { if (index<p>One try corresponds to multiple catches: </p>
In the case of multiple catches, the catch of the parent class should be placed at the bottom, otherwise the compilation will be empty.
5. Principles of exception handling
If an exception that needs to be detected is thrown inside a function, it must be declared in the function, or it must be caught with try catch within the function. , otherwise the compilation fails.
If the function that declares an exception is called, either try catch or throws, otherwise the compilation fails.
When to catch? When throws?
Function content can be solved by using catch.
If it cannot be solved, use throws to tell the caller, and the caller will solve it.
一个功能如果抛出了多个异常,那么调用时,必须有对应的多个catch来进行针对性处理。
内部有几个需要检测的异常,就抛几个异常,抛出几个就catch几个异常。
六、finally
通常用于关闭(释放)资源。必须要执行。除非jvm虚拟机挂了。
范例:出门玩,必须关门,所以将关门这个动作放在finally里面,必须执行。
凡是涉及到关闭连接等操作,要用finally代码块来释放资源。
try catch finally 代码块组合特点:
try catch finally:当有资源需要释放时,可以定义finally
try catch(多个):当没有资源需要释放时,可以不定义finally
try finally:异常处理不处理我不管,但是我得关闭资源,因为资源是我开的,得在内部关掉资源。
范例:
try{ //连接数据库 } //没有catch意思不处理异常,只单纯的捕获异常 finally{ //关闭连接 }
七、异常的应用
老师用电脑讲课范例:
电脑类:
public class Computer { private int state = 2; public void run() throws lanpingExcption,maoyanExcption{ if (state == 1){ throw new lanpingExcption("电脑蓝屏啦~"); }else if (state == 2){ throw new maoyanExcption("电脑冒烟啦~"); } System.out.println("电脑启动"); } public void chongqi(){ state = 0; System.out.println("重启电脑!"); } }
老师类:
public class Teacher { private String name; private Computer computer; Teacher(String name){ this.name = name; computer = new Computer(); } void teach() throws maoyanExcption{ try { computer.run(); System.out.println(this.name + "开始用电脑讲课了"); } catch (lanpingExcption l) { l.printStackTrace(); computer.chongqi(); teach();//重启后再次讲课 } catch (maoyanExcption m) { m.printStackTrace(); test(); throw m; } } public void test(){ System.out.println("大家自己练习去~"); } }
蓝屏异常类:
public class lanpingExcption extends Exception{ lanpingExcption (String msg){ super(msg); } }
冒烟异常类:
public class maoyanExcption extends Exception { maoyanExcption (String msg){ super(msg); } }
主函数:
public class Testmain { public static void main (String[] args){ Teacher teacher = new Teacher("丁老师"); try { teacher.teach(); } catch (maoyanExcption m) { //m.printStackTrace(); System.out.println("。。。。。"); } } }
八、异常的注意事项:
子类在覆盖父类方法时,父类的方法如果抛出了异常,那么子类的方法只能抛出父类的异常或者该异常的子类。
如果父类抛出多个异常,那么子类只能抛出父类异常的子集。
子类覆盖父类,只能抛出父类的异常或者子类。
如果父类的方法没有抛出异常,子类覆盖时绝对不能抛。
The above is the detailed content of How to handle exception types in Java. For more information, please follow other related articles on the PHP Chinese website!
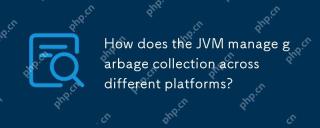
JVMmanagesgarbagecollectionacrossplatformseffectivelybyusingagenerationalapproachandadaptingtoOSandhardwaredifferences.ItemploysvariouscollectorslikeSerial,Parallel,CMS,andG1,eachsuitedfordifferentscenarios.Performancecanbetunedwithflagslike-XX:NewRa
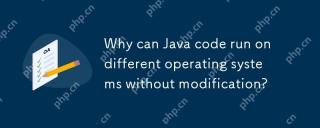
Java code can run on different operating systems without modification, because Java's "write once, run everywhere" philosophy is implemented by Java virtual machine (JVM). As the intermediary between the compiled Java bytecode and the operating system, the JVM translates the bytecode into specific machine instructions to ensure that the program can run independently on any platform with JVM installed.
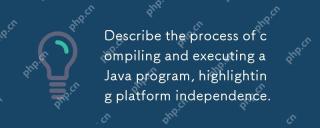
The compilation and execution of Java programs achieve platform independence through bytecode and JVM. 1) Write Java source code and compile it into bytecode. 2) Use JVM to execute bytecode on any platform to ensure the code runs across platforms.
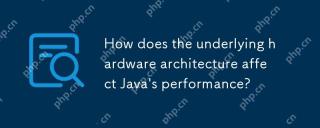
Java performance is closely related to hardware architecture, and understanding this relationship can significantly improve programming capabilities. 1) The JVM converts Java bytecode into machine instructions through JIT compilation, which is affected by the CPU architecture. 2) Memory management and garbage collection are affected by RAM and memory bus speed. 3) Cache and branch prediction optimize Java code execution. 4) Multi-threading and parallel processing improve performance on multi-core systems.
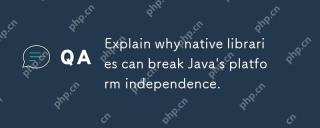
Using native libraries will destroy Java's platform independence, because these libraries need to be compiled separately for each operating system. 1) The native library interacts with Java through JNI, providing functions that cannot be directly implemented by Java. 2) Using native libraries increases project complexity and requires managing library files for different platforms. 3) Although native libraries can improve performance, they should be used with caution and conducted cross-platform testing.
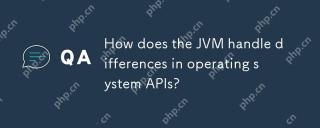
JVM handles operating system API differences through JavaNativeInterface (JNI) and Java standard library: 1. JNI allows Java code to call local code and directly interact with the operating system API. 2. The Java standard library provides a unified API, which is internally mapped to different operating system APIs to ensure that the code runs across platforms.
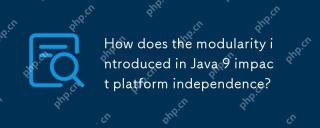
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
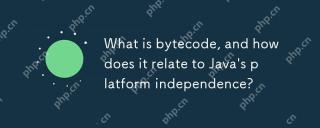
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
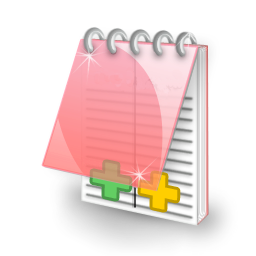
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
