Decoding method
A message containing the letters A-Z is encoded with the following mapping:
- ##'A' -> "1"
- 'B' -> "2"
- ...
- 'Z' -> " 26"
- Example 1:
Input: s = "12"Output: 2Explanation: It can be decoded as "AB" (1 2) or "L" (12).
- Example 2:
Input: s = "226"Output: 3Explanation: It can be decoded as "BZ" (2 26), "VF" (22 6), or "BBF" (2 2 6).
- Example 3:
Input: s = "0"Output: 0Explanation: No character maps to a number starting with 0. Valid mappings containing 0 are 'J' -> "10" and 'T'-> "20" . Since there are no characters, there is no efficient way to decode this, as all numbers need to be mapped. Tip:
1s contain only digits and may contain leading zeros. Method 1: Dynamic Programming (Java) For a given string s, let its length be n, and the characters in it from left to right are s[1],s [2],...,s[n]. We can use dynamic programming to calculate the number of decoding methods for a string. Specifically, let fi represent the number of decoding methods of the first i characters s[1..i] of the string s. When performing state transfer, we can consider which characters in s were used for the last decoding
class Solution { public int numDecodings(String s) { int n = s.length(); int[] f = new int[n + 1]; f[0] = 1; for (int i = 1; i <= n; ++i) { if (s.charAt(i - 1) != '0') { f[i] += f[i - 1]; } if (i > 1 && s.charAt(i - 2) != '0' && ((s.charAt(i - 2) - '0') * 10 + (s.charAt(i - 1) - '0') <= 26)) { f[i] += f[i - 2]; } } return f[n]; } }Time complexity: o(n)Space complexity: o(n)Method Two: Dynamic Programming - Optimization (go) Please see the above description for specific method ideas. This method optimizes the space complexity. By using temporary variables, Reduce the space complexity from o(n) to o(1)
func numDecodings(s string) int { n := len(s) // a = f[i-2], b = f[i-1], c = f[i] a, b, c := 0, 1, 0 for i := 1; i <= n; i++ { c = 0 if s[i-1] != '0' { c += b } if i > 1 && s[i-2] != '0' && ((s[i-2]-'0')*10+(s[i-1]-'0') <= 26) { c += a } a, b = b, c } return c }Time complexity: o(n)Space complexity: o(1)
The above is the detailed content of Go Java algorithm decoding method example code analysis. For more information, please follow other related articles on the PHP Chinese website!
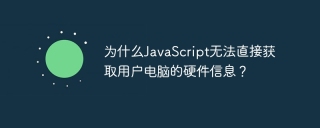
Discussion on the reasons why JavaScript cannot obtain user computer hardware information In daily programming, many developers will be curious about why JavaScript cannot be directly obtained...
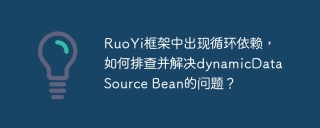
RuoYi framework circular dependency problem troubleshooting and solving the problem of circular dependency when using RuoYi framework for development, we often encounter circular dependency problems, which often leads to the program...

About SpringCloudAlibaba microservices modular development using SpringCloud...
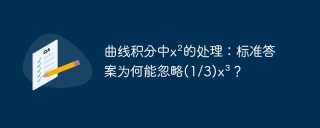
Questions about a curve integral This article will answer a curve integral question. The questioner had a question about the standard answer to a sample question...
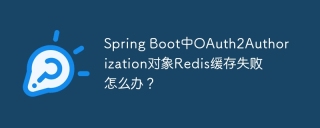
In SpringBoot, use Redis to cache OAuth2Authorization object. In SpringBoot application, use SpringSecurityOAuth2AuthorizationServer...
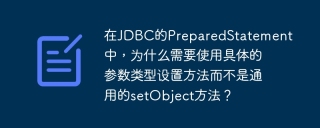
JDBC...
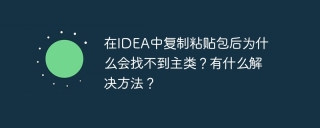
Why can't the main class be found after copying and pasting the package in IDEA? Using IntelliJIDEA...
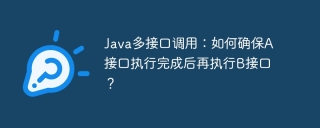
State synchronization between Java multi-interface calls: How to ensure that interface A is called after it is executed? In Java development, you often encounter multiple calls...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
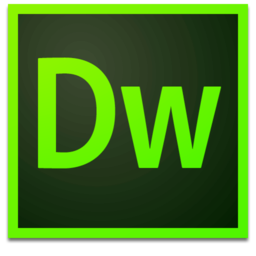
Dreamweaver Mac version
Visual web development tools
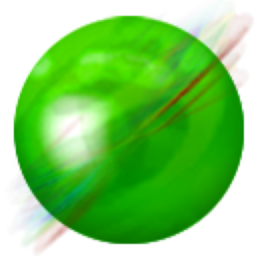
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
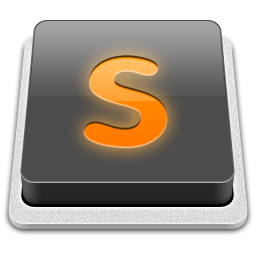
SublimeText3 Mac version
God-level code editing software (SublimeText3)