In PHP development, sometimes we need to convert a string into an array for further operations and processing. In this article, we will introduce how to convert a PHP string to an array.
PHP provides a variety of methods to convert strings into arrays. The choice of specific methods depends on the format and content of the string. Below we discuss common methods.
- explode function
The explode function is the most common and commonly used method in PHP to convert a string into an array. Its syntax is as follows:
array explode ( string $delimiter , string $string [, int $limit = PHP_INT_MAX ] )
Among them, $delimiter represents the delimiter, $string represents the string that needs to be separated, and $limit represents the upper limit of the number of array elements returned.
For example, if we convert the comma-separated string "1,2,3,4,5" into an array, we can use the following code:
$str = "1,2,3,4,5"; $arr = explode(",", $str); print_r($arr);
The output result is as follows:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
- str_split function
The str_split function can split a string into multiple substrings according to the specified length and return an array of these substrings. The syntax of this function is as follows:
array str_split ( string $string [, int $split_length = 1 ] )
Among them, $string represents the string that needs to be split, and $split_length represents the length of each substring.
For example, if we split the string "abcdefg" into an array according to each letter, we can use the following code:
$str = "abcdefg"; $arr = str_split($str); print_r($arr);
The output result is as follows:
Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g )
- preg_split function
If you need to split a string according to a regular expression, you can use the preg_split function. The syntax of this function is as follows:
array preg_split ( string $pattern , string $subject [, int $limit = -1 [, int $flags = 0 ]] )
Among them, $pattern represents the matching pattern of the regular expression, $subject represents the string that needs to be split, $limit represents the upper limit of the number of returned array elements, and $flags represents matching. flag bit.
For example, if we divide the string "1,2,3,a,b,c" into an array according to numbers and letters, we can use the following code:
$str = "1,2,3,a,b,c"; $arr = preg_split("/[, ]/", $str); print_r($arr);
The output result is as follows :
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => a [4] => b [5] => c )
- unserialize function
If the string is serialized data, you can use the unserialize function to convert it into an array. The syntax of this function is as follows:
mixed unserialize ( string $str )
Among them, $str represents the string that needs to be deserialized.
For example, we will serialize the data "a:3:{i:0;s:1:"1";i:1;s:1:"2";i:2;s :1:"3";}" to convert to an array, you can use the following code:
$str = 'a:3:{i:0;s:1:"1";i:1;s:1:"2";i:2;s:1:"3";}'; $arr = unserialize($str); print_r($arr);
The output result is as follows:
Array ( [0] => 1 [1] => 2 [2] => 3 )
- json_decode function
If the string is data in JSON format, you can use the json_decode function to convert it to an array. The syntax of this function is as follows:
mixed json_decode ( string $json [, bool $assoc = false [, int $depth = 512 [, int $options = 0 ]]] )
Among them, $json represents the JSON string that needs to be decoded, $assoc represents whether to return an associative array, $depth represents the depth of recursion, and $options represents the decoding options.
For example, if we convert the data in JSON format "{"name":"Jack","age":20,"gender":"male"}" into an array, we can use the following code:
$str = '{"name":"Jack","age":20,"gender":"male"}'; $arr = json_decode($str, true); print_r($arr);
The output results are as follows:
Array ( [name] => Jack [age] => 20 [gender] => male )
Summary
There are many ways to convert strings to arrays in PHP, including the explode function, str_split function, preg_split function, unserialize function and json_decode function. Developers can choose appropriate methods for data processing based on actual needs to improve development efficiency and code quality.
The above is the detailed content of How to convert PHP string to array. For more information, please follow other related articles on the PHP Chinese website!
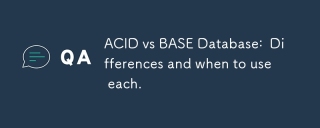
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
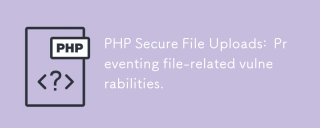
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
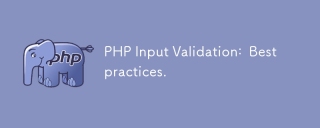
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
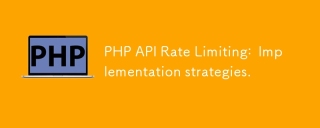
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
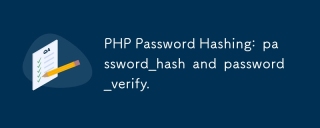
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
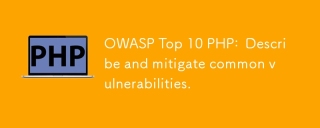
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
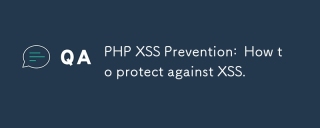
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
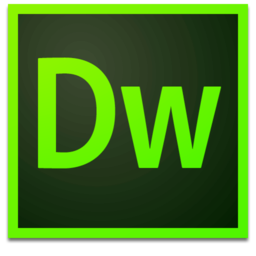
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
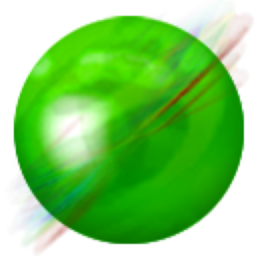
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!