In PHP, array is a very commonly used data type, which allows us to store a set of data and access and operate these data by specifying subscripts. Sometimes, when operating on an array, we may need to replace a string in the array. In this article, we will explore how to use PHP arrays to replace a certain string.
- Use array_walk() function
array_walk() function is a very powerful array function in PHP. It can traverse each element in the array and Perform the specified operation. When performing string replacement, we can define a callback function, which will be called by the array_walk() function and used to replace elements. The following is a basic sample code:
<?php $myArray = array("apple", "banana", "cherry"); function replaceStr(&$value, $key, $replacement) { $value = str_replace("a", $replacement, $value); } array_walk($myArray, 'replaceStr', 'x'); print_r($myArray); ?>
In the above sample code, we first define an array $myArray containing three elements. Next, a replacement function replaceStr() is defined, which accepts three parameters: $value represents the value of the array element currently traversed, $key represents the subscript of the current element, and $replacement represents the replaced string. In the function, we use PHP's built-in str_replace() function to replace all "a" characters in the currently traversed element with the value specified by the $replacement parameter. Note that the & symbol is added in front of this function, which means that the $value parameter passed in is a reference type, that is to say, modifications to it will directly affect the elements in the array.
Finally, we use the array_walk() function to traverse the entire array $myArray, and call the replaceStr() function to perform string replacement on each element. Finally, when we print out the modified array, we can see that the "a" character in each element has been replaced by the "x" character.
- Use the array_map() function
Similar to the array_walk() function, the array_map() function can also traverse each element in the array and specify it operate. The difference is that the array_map() function will return a new array, and the original array will not be modified. When performing string replacement operations, we can create a new function to replace the original element and return the modified value. The following is a sample code:
<?php $myArray = array("apple", "banana", "cherry"); function replaceStr($value, $replacement) { return str_replace("a", $replacement, $value); } $newArray = array_map('replaceStr', $myArray, 'x'); print_r($myArray); print_r($newArray); ?>
In the above sample code, we also first define an array $myArray containing three elements. Next, a replacement function replaceStr() is defined, which accepts two parameters: $value represents the value of the array element currently traversed, and $replacement represents the replaced string. In the function, we also use PHP's built-in str_replace() function to replace all "a" characters in the currently traversed element with the value specified by the $replacement parameter. Finally, we return the modified value as a new element, which is used to construct a new array.
Finally, we print out both the original array $myArray and the new array $newArray. We can see that the original array has not been modified, and each element in the new array has been modified to the replaced one. String.
Summary
Both of the above two methods can easily replace strings in PHP arrays. The array_walk() function is suitable for scenarios where the original array is directly modified, while the array_map() function is suitable for scenarios where a new array is returned. In practical applications, we can choose the appropriate method to operate according to our needs.
The above is the detailed content of How to replace a string in a php array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
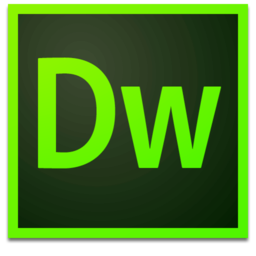
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor
