Let me show you the renderings first (source code download is supported):
When we buy tickets online (such as movie tickets, train tickets, etc.), we can choose our own seats. The developer will list the seats on the page, and users can clearly see the available seats and payment at a glance. This article takes movie theater ticket purchase as an example to show you how to select seats, process seat selection data, etc.
Here, I would like to introduce to you an online seat selection plug-in based on jQuery: jQuery Seat Charts, which supports custom seat types and prices, custom styles, and settings. The selected seat also supports keyboard control of the seat.
HTML
We assume that we enter the seat selection page of the movie "Interstellar". Please see the big picture above for the page layout. The left side of the page will be in #seat-map The seat layout of the theater is displayed. The #booking-details on the right displays movie-related information as well as the selected seat information #selected-seats and ticket amount information. After selecting the seat, confirm to the payment page to complete the payment.
屏幕影片:星际穿越3D时间:11月14日 21:00座位:票数:0总计:¥0
CSS
Use CSS to convert each element on the page Element beautification, especially the seat list layout, sets different styles for seat status (sold, optional seats, selected seats, etc.). We have sorted out the CSS code. Of course, you can modify any CSS according to the style of your project page. code.
.front{width: 300px;margin: 5px 32px 45px 32px;background-color: #f0f0f0; color: #666;text-align: center;padding: 3px;border-radius: 5px;} .booking-details {float: right;position: relative;width:200px;height: 450px; } .booking-details h3 {margin: 5px 5px 0 0;font-size: 16px;} .booking-details p{line-height:26px; font-size:16px; color:#999} .booking-details p span{color:#666} p.seatCharts-cell {color: #182C4E;height: 25px;width: 25px;line-height: 25px;margin: 3px;float: left;text-align: center;outline: none;font-size: 13px;} p.seatCharts-seat {color: #fff;cursor: pointer;-webkit-border-radius:5px;-moz-border-radius:5px;border-radius: 5px;} p.seatCharts-row {height: 35px;} p.seatCharts-seat.available {background-color: #B9DEA0;} p.seatCharts-seat.focused {background-color: #76B474;border: none;} p.seatCharts-seat.selected {background-color: #E6CAC4;} p.seatCharts-seat.unavailable {background-color: #472B34;cursor: not-allowed;} p.seatCharts-container {border-right: 1px dotted #adadad;width: 400px;padding: 20px;float: left;} p.seatCharts-legend {padding-left: 0px;position: absolute;bottom: 16px;} ul.seatCharts-legendList {padding-left: 0px;} .seatCharts-legendItem{float:left; width:90px;margin-top: 10px;line-height: 2;} span.seatCharts-legendDescription {margin-left: 5px;line-height: 30px;} .checkout-button {display: block;width:80px; height:24px; line-height:20px;margin: 10px auto;border:1px solid #999;font-size: 14px; cursor:pointer} #selected-seats {max-height: 150px;overflow-y: auto;overflow-x: none;width: 200px;} #selected-seats li{float:left; width:72px; height:26px; line-height:26px; border:1px solid #d3d3d3; background:#f7f7f7; margin:6px; font-size:14px; font-weight:bold; text-align:center}
jQuery
jQuery
This example is based on jQuery, so don’t forget to load the jquery library and seat selection plug-in: jQuery Seat Charts first.
? <script type="text/javascript" src="jquery.js"></script> <script type="text/javascript" src="jquery.seat-charts.min.js"></script>
Next we define elements such as fare, seating area, number of tickets, total amount, and then call the plug-in: $('#seat-map').seatCharts() .
We first set up the seating map. The seats in a theater are fixed. In this example, the third row is the aisle, and the empty seats on the right side of the third and fourth rows are the exits. We have couple seats in the last row, so the layout of the screening room is as follows:
aaaaaaaaaa
aaaaaaaaaa
__________
aaaaaaaa__
aaaaaaaaaa
aaaaaaaaaa
aaaaaaaaaa
aaaaaaaaaa
aaaaaaaaaa
aa__aa __aa
We use the letter a to represent the seat and the symbol _ Empty means there are no seats. Of course, you can also use a, b, c, etc. to represent seats of different levels.
Then define the legend style. The key is to detect the click event click(): when the user clicks on the seat, if the seat status is available (available), then after clicking on the seat, the seat information (rows and rows) will be displayed. seat) is added to the selected seat list on the right, and the total number of votes and total amount are calculated; if the seat status is selected (selected), then after clicking the seat again, the selected seat information will be added from the seat on the right Delete it from the list and set the status to optional; if the seat status is sold (unavailable), the seat cannot be clicked. Finally, use the get() method to set the status of the sold seat number to sold.
The following is the detailed code:
var price = 80; //票价 $(document).ready(function() { var $cart = $('#selected-seats'), //座位区 $counter = $('#counter'), //票数 $total = $('#total'); //总计金额 var sc = $('#seat-map').seatCharts({ map: [ //座位图 'aaaaaaaaaa', 'aaaaaaaaaa', '__________', 'aaaaaaaa__', 'aaaaaaaaaa', 'aaaaaaaaaa', 'aaaaaaaaaa', 'aaaaaaaaaa', 'aaaaaaaaaa', 'aa__aa__aa' ], legend : { //定义图例 node : $('#legend'), items : [ [ 'a', 'available', '可选座' ], [ 'a', 'unavailable', '已售出'] ] }, click: function () { //点击事件 if (this.status() == 'available') { //可选座 $('<li>'+(this.settings.row+1)+'排'+this.settings.label+'座</li>') .attr('id', 'cart-item-'+this.settings.id) .data('seatId', this.settings.id) .appendTo($cart); $counter.text(sc.find('selected').length+1); $total.text(recalculateTotal(sc)+price); return 'selected'; } else if (this.status() == 'selected') { //已选中 //更新数量 $counter.text(sc.find('selected').length-1); //更新总计 $total.text(recalculateTotal(sc)-price); //删除已预订座位 $('#cart-item-'+this.settings.id).remove(); //可选座 return 'available'; } else if (this.status() == 'unavailable') { //已售出 return 'unavailable'; } else { return this.style(); } } }); //已售出的座位 sc.get(['1_2', '4_4','4_5','6_6','6_7','8_5','8_6','8_7','8_8', '10_1', '10_2']).status('unavailable'); }); //计算总金额 function recalculateTotal(sc) { var total = 0; sc.find('selected').each(function () { total += price; }); return total; }
The above code uses jquery to implement online seat selection and reservation for theaters. I hope you like it!
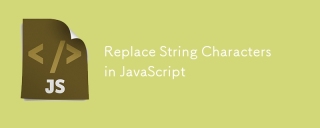
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
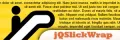
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
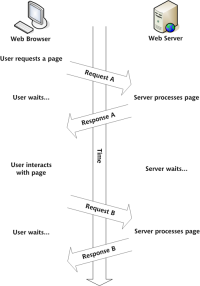
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
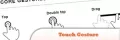
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
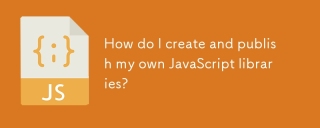
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
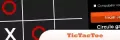
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
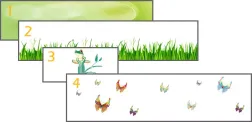
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
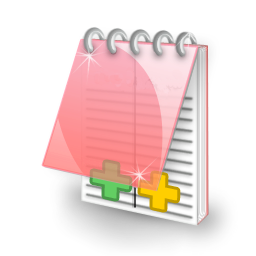
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
