In Web development, data interaction between the backend and the frontend is a very important topic. Due to the popularity of JavaScript, more and more front-end developers choose to use axios to send asynchronous requests to obtain background data. So how to receive the array from axios in the background?
First, let us understand what axios is. axios is an open source JavaScript library for sending asynchronous requests. It can be installed in a Node.js environment or used in the browser. Using axios, you can easily handle various types of HTTP requests, such as GET, POST, etc., and it also supports sending data, including JSON, form data, etc. In the front-end, we usually use axios to send requests and display the received data, but in the background, we need to receive and process the data sent by axios.
Next, we will introduce how to receive the array from axios in the php background.
Step 1: Use axios to send data
First, in the front end, we need to use axios to send an array to the background. The code is as follows:
axios.post('/backend.php', { data: [1, 2, 3, 4, 5] }) .then(function (response) { console.log(response); }) .catch(function (error) { console.log(error); });
Through the above code, we send an array to the background at the address /backend.php, and the value of the array is [1, 2, 3, 4, 5]. The amount of data passed by this array is relatively small, but it can be used to demonstrate how to receive the array from axios in the background.
Step 2: Receive data in the php background
In the php background, we can use the $_POST array to receive the array data from axios. The code is as follows:
$data = $_POST['data']; if (isset($data)) { // 对数据进行处理 }
Through the above code, we get the data with the key "data" from the $_POST array. If the data is obtained, we can process the data.
Step 3: Parse the array data
After receiving the array data from axios, we can use the json_decode function to parse the array data. The code is as follows:
$data = $_POST['data']; if (isset($data)) { $dataArray = json_decode($data); }
The above code assigns the parsed array data to the $dataArray variable.
Step 4: Traverse the array data
Next, we can traverse and operate the array data. For example, we can use the foreach statement to traverse the array. The code is as follows:
$data = $_POST['data']; if (isset($data)) { $dataArray = json_decode($data); foreach ($dataArray as $value) { // 对数组元素进行操作 } }
Through the above code, we traverse the passed array elements and can perform various operations, such as accumulating and calculating the values of the array. average and so on.
Step 5: Return the processed data to the front end
After processing the array data, we need to return the result to the front end. You can use the echo statement to return the processed data to the front end. The data is returned to the front end. For example, we can perform the json_encode operation on the processed array data. The code is as follows:
$data = $_POST['data']; if (isset($data)) { $dataArray = json_decode($data); $result = array_sum($dataArray); // 对数组元素进行求和 echo json_encode(array('result' => $result)); // 返回对数组求和的结果 }
Through the above code, we return the result of the array sum to the front end and wrap it in a json object. In the front end, we can get the results returned after background processing through response.data.result.
Summary
Receiving the array from axios in the PHP background is essentially to obtain the data through the $_POST array, use the json_decode function to parse the data, and then traverse and summarize the array elements. operate. Finally, we need to return the processed data to the front end. In actual development, we may need to perform more complex processing on the array, such as filtering and sorting the data in the array. Fortunately, PHP provides powerful array processing functions. We can use these functions to easily perform various operations on arrays to quickly complete complex data processing tasks.
The above is the detailed content of How to receive array from axios in php background. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
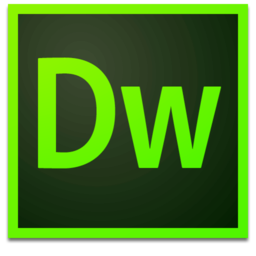
Dreamweaver Mac version
Visual web development tools
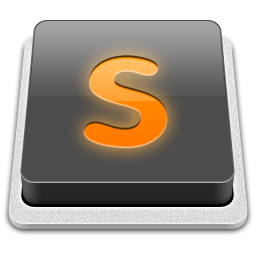
SublimeText3 Mac version
God-level code editing software (SublimeText3)
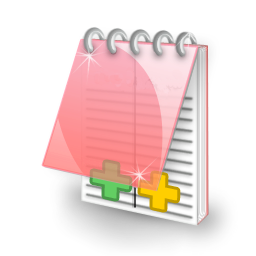
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
