Vue is a very popular JavaScript framework that provides a powerful platform for building web applications. Components are one of the most important and powerful features of Vue. It allows us to split complex applications into front-end reusable parts, making development more efficient and simpler.
So how to build a Vue component? This article will show you the basic knowledge, establishment methods and best practices of Vue components.
Basic knowledge of Vue components
First we need to understand some basic knowledge of Vue component development.
Component definition
There are two ways to define components in Vue:
One is global registration (Global registration), which defines the component in the global Vue instance:
Vue.component('component-name', { // 组件的选项 })
We can use this component in any Vue instance, including other components.
The second is local registration, which defines the component in the options of the current component:
new Vue({ el: '#app', components: { 'component-name': { // 组件的选项 } } })
This component can only be used in the current instance.
Component Template
The way to define component templates is more flexible. You can use HTML template strings or directly use the Render function.
Vue.component('component-name', { template: '<div>组件模板</div>' })
Vue.component('component-name', { render: function(h) { return h('div', '组件模板') } })
It is recommended to use HTML template strings as it is more intuitive and easier to read.
Component properties
Components can have input and output properties. Input properties (props) are the data flowing from the parent component to the child component, and the output properties are the child components communicating data to the parent component.
Vue.component('component-name', { props: ['prop-name'], template: '<div>{{ prop-name }}</div>' })
When using a component in a parent component, input attributes can be passed through HTML attributes (note that the attribute name uses "kebab-case").
<component-name></component-name>
In the child component, access this property through this.propName
.
Component life cycle
The Vue component life cycle refers to the entire process from creation to destruction of the component instance, including the stages of creation, mounting, updating and destruction.
The life cycle of components can be registered with the following hooks:
Vue.component('component-name', { created: function() { // 组件创建时调用,可以在这里初始化数据 }, mounted: function() { // 将组件挂载到DOM后调用,可以在这里访问DOM节点 }, updated: function() { // 组件更新时调用,可以在这里修改数据 }, destroyed: function() { // 组件销毁时调用,可以在这里清理一些无用的数据和资源 } })
How to build Vue components
After understanding the basic knowledge, we will explain how to build Vue components.
1. Determine the component name
First, we need to determine the name of the component to be developed. This name is not only the name of the component in the Vue instance, but also the name when we package it and publish it into the public component library.
Component names in Vue can use "kebab-case" format or "camelCase" format. It is recommended to use the "kebab-case" format as it is easier to read.
2. Create component files
Create component files and define components.
<template> <div> 组件模板 </div> </template> <script> export default { name: 'component-name', props: { propName: { type: String, default: '' } }, data() { return { // 组件的数据 } }, methods: { // 组件的方法 }, mounted() { // 组件挂载到DOM后执行的方法 } } </script> <style> /* 组件的样式 */ </style>
Use Vue single file component form to define component templates, scripts and styles in the same file for easy management.
3. Export the component
After the component is defined, export it for use elsewhere.
import MyComponent from './MyComponent.vue' export default MyComponent
Best Practices
Finally, let’s share some best practices for Vue component development.
- Component naming convention: Naming should be as descriptive as possible, describing the purpose and characteristics of the component, while avoiding conflicts or duplicate names.
- Provide documentation for components: Components should have clear and concise documentation, including how to use the component and API documentation, so that others can use and understand it.
- Single function principle: Each component should have only one clear and clear function, and this function should be as independent and reusable as possible.
- Component style encapsulation: The style of a component should be encapsulated inside the component as much as possible, using scoped styles to reduce repeated definitions.
- Use self-closing tags: Try to use self-closing tags to reduce errors.
- Use the default v-bind directive: Use the default directive to avoid destroying the component's input attributes, such as
$props
and$attrs
. - Loading on demand: Some components may not be used many times. We can dynamically load them when needed to improve the loading speed of the first screen.
Conclusion
This article introduces the basic knowledge, construction methods and best practices of Vue components. Vue components are a very powerful feature of the Vue framework, which can realize code reuse and component development. Through the study and practice of this article, I believe you can better utilize Vue components to build reusable, scalable and easy-to-maintain web applications.
The above is the detailed content of How to build vue components. For more information, please follow other related articles on the PHP Chinese website!

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
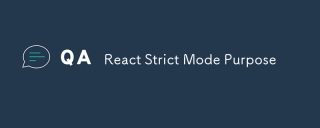
React Strict Mode is a development tool that highlights potential issues in React applications by activating additional checks and warnings. It helps identify legacy code, unsafe lifecycles, and side effects, encouraging modern React practices.
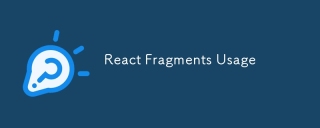
React Fragments allow grouping children without extra DOM nodes, enhancing structure, performance, and accessibility. They support keys for efficient list rendering.
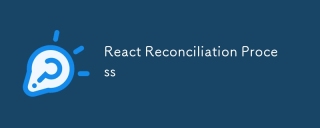
The article discusses React's reconciliation process, detailing how it efficiently updates the DOM. Key steps include triggering reconciliation, creating a Virtual DOM, using a diffing algorithm, and applying minimal DOM updates. It also covers perfo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
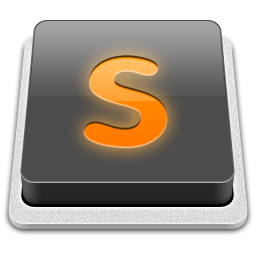
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
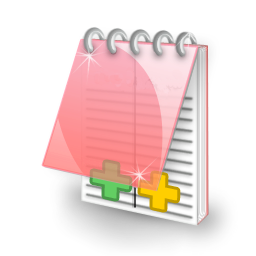
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function