What impact does the type shielding feature of generics in Java have on code?
Use generics to shield type differences
In C language, there is a very useful template function, which can write a general version with parameterized types and let the compiler automatically generate Specific versions for different types. In the Java language, there is a similar function called generics. When writing classes and methods, specific types are generally used, and generics can be used to parameterize types, so that more general code can be written.
Many people believe that the two concepts of C template (template) and Java generic (generic) are equivalent, but in fact the implementation mechanisms are completely different. C template is a set of macro instructions, and the compiler will create a copy of the template code for each type; the implementation of Java generics is based on the concept of "type erasure", which is essentially a syntactic sugar for type restrictions.
1. Generic class
Take the support class as an example to define the general support class of generics:
/** 通用支撑类 */@Getter@Setter@ToStringpublic class GenericHolder<t> { /** 通用取值 */ private T value; /** 构造函数 */ public GenericHolder() {} /** 构造函数 */ public GenericHolder(T value) { this.value = value; } }</t>
2. Generic interface
Definition Generic data provider interface:
/** 数据提供者接口 */public interface DataProvider<t> { /** 获取数据函数 */ public T getData(); }</t>
3. Generic methods
Define generic shallow copy functions:
/** 浅拷贝函数 */public static <t> T shallowCopy(Object source, Class<t> clazz) throws BeansException { // 判断源对象 if (Objects.isNull(source)) { return null; } // 新建目标对象 T target; try { target = clazz.newInstance(); } catch (Exception e) { throw new BeansException("新建类实例异常", e); } // 拷贝对象属性 BeanUtils.copyProperties(source, target); // 返回目标对象 return target; }</t></t>
4. Generic wildcards
Generic wildcards generally use "?" instead of specific type arguments. You can regard "?" as the parent class of all types. When the specific type is uncertain, you can use the generic wildcard "?"; when you do not need to use the specific functions of the type and only use the functions in the Object class, you can use the generic wildcard "?".
/** 打印取值函数 */public static void printValue(GenericHolder> holder) { System.out.println(holder.getValue()); }/** 主函数 */public static void main(String[] args) { printValue(new GenericHolder(12345)); printValue(new GenericHolder("abcde")); }
In the Java specification, it is not recommended to use the generic wildcard "?". The above function can be changed to:
/** 打印取值函数 */public static <t> void printValue(GenericHolder<t> holder) { System.out.println(holder.getValue()); }</t></t>
5. Generic upper and lower bounds
When using generics When type, we can also set upper and lower bounds for the passed in generic type actual parameters. For example, type actual parameters are only allowed to pass in a certain type of parent class or a certain type of subclass. The declaration of the upper and lower bounds of a generic type must be placed together with the declaration of the generic type.
Upper bound wildcard (extends):
The upper bound wildcard is "extends", which can accept its specified type or its subclass as a general parameter. There is also a special form that specifies that it must not only be a subclass of the specified type, but also implement certain interfaces. For example: List extends A> indicates that this is a List of a specific subclass of A, and the saved object must be A or a subclass of A. For the List extends A> list, you cannot add A or a subclass object of A, but can only get the object of A.
Lower bound wildcard (super):
The lower bound wildcard is "super", which can accept its specified type or its parent class as a general parameter. For example: List super A> indicates that this is a List of a specific parent class of A, and the saved object must be A or a superclass of A. For List super A> list, you can add A or a subclass object of A, but you can only get the object of Object.
PECS (Producer Extends Consumer Super) principle:
When providing data as a producer (reading out), it is suitable to use upper bound wildcards (extends);
As a consumer consuming data (reading in) When writing), it is appropriate to use the lower bound wildcard (super).
In daily coding, the upper bound wildcard (extends) is more commonly used, which is used to limit the parent class of a generic type. The example code is as follows:
/** 数字支撑类 */@Getter@Setter@ToStringpublic class NumberHolder{ /** 通用取值 */ private T value; /** 构造函数 */ public NumberHolder() {} /** 构造函数 */ public NumberHolder(T value) { this.value = value; } }/** 打印取值函数 */public static <t> void printValue(GenericHolder<t> holder) { System.out.println(holder.getValue()); }</t></t>
The above is the detailed content of What impact does the type shielding feature of generics in Java have on code?. For more information, please follow other related articles on the PHP Chinese website!
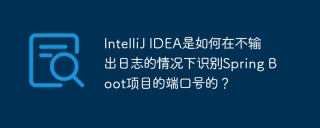
Start Spring using IntelliJIDEAUltimate version...
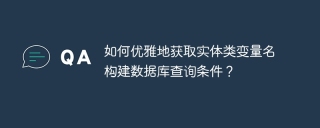
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
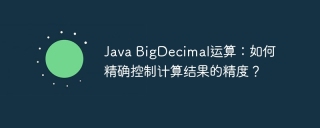
Java...
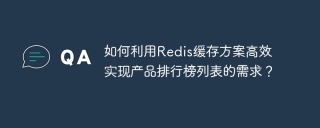
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
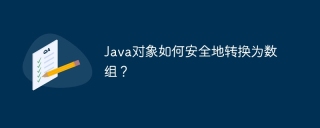
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
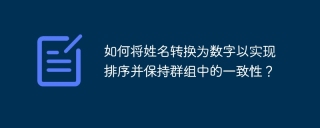
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
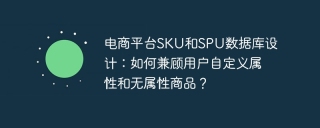
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
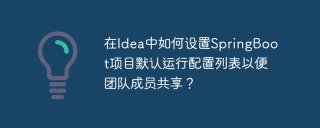
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
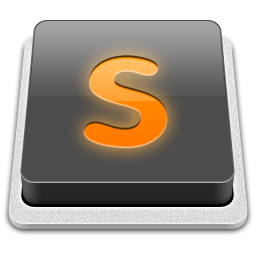
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor