Illustration
The kmp algorithm has a certain similarity with the idea of the bm algorithm mentioned before. As mentioned before, there is the concept of a good suffix in the bm algorithm, and there is a concept of a good prefix in kmp. What is a good prefix? Let's first look at the following example.
Observe the above example, the already matched abcde is called a good prefix, a does not match the following bcde, so there is no need to compare again, slide directly after e That’s it.
What if there are matching characters in the good prefix?
Observe the above example, if we slide directly after the good prefix at this time, we will slide too much and miss the matching substring. So how do we perform reasonable sliding based on good prefixes?
In fact, it is to check whether the prefix and suffix of the current good prefix match, find the longest matching length, and slide directly. In view of finding the longest matching length more than once, we can initialize an array first and save the longest matching length under the current good prefix. At this time, our next array will come out.
We define a next array, which represents the length of the longest matching substring of the prefix and suffix of the good prefix under the current good prefix. This longest matching length means that this substring has been matched before, not It is necessary to match again, starting directly from the next character of the substring.
Do we need to match every character every time we calculate next[i]? Can we deduce based on next[i - 1] to reduce unnecessary comparisons? .
With this idea, let’s take a look at the following steps:
Assume next[i - 1] = k - 1;
If modelStr[k] = modelStr[ i] then next[i]=k
int[] next ; /** * 初始化next数组 * @param modelStr */ public void init(char[] modelStr) { //首先计算next数组 //遍历modelStr,遍历到的字符与之前字符组成一个串 next = new int[modelStr.length]; int start = 0; while (start < modelStr.length) { next[start] = this.recursion(start, modelStr); ++ start; } } /** * * @param i 当前遍历到的字符 * @return */ private int recursion(int i, char[] modelStr) { //next记录的是个数,不是下标 if (0 == i) { return 0; } int last = next[i -1]; //没有匹配的,直接判断第一个是否匹配 if (0 == last) { if (modelStr[last] == modelStr[i]) { return 1; } return 0; } //如果last不为0,有值,可以作为最长匹配的前缀 if (modelStr[last] == modelStr[i]) { return next[i - 1] + 1; } //当next[i-1]对应的子串的下一个值与modelStr不匹配时,需要找到当前要找的最长匹配子串的次长子串 //依据就是次长子串对应的子串的下一个字符==modelStr[i]; int tempIndex = i; while (tempIndex > 0) { last = next[tempIndex - 1]; //找到第一个下一个字符是当前字符的匹配子串 if (modelStr[last] == modelStr[i]) { return last + 1; } -- tempIndex; } return 0; }and then start using the next array Match, start matching from the first character, and find the first unmatched character. At this time, all the previous ones are matched. Next, first judge whether it is a complete match. If it is a direct return, if not, judge whether the first character is matched. If one does not match, it will match directly to the next one. If there is a good prefix, the next array is used at this time. Through the next array, we know where the current matching can start, and there is no need to match the previous ones.
public int kmp(char[] mainStr, char[] modelStr) { //开始进行匹配 int i = 0, j = 0; while (i + modelStr.length <= mainStr.length) { while (j < modelStr.length) { //找到第一个不匹配的位置 if (modelStr[j] != mainStr[i]) { break; } ++ i; ++ j; } if (j == modelStr.length) { //证明完全匹配 return i - j; } //走到这里找到的是第一个不匹配的位置 if (j == 0) { ++ i; continue; } //从好前缀后一个匹配 j = next[j - 1]; } return -1; }
The above is the detailed content of What is the implementation method of KMP algorithm in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
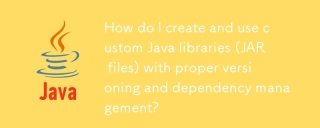
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
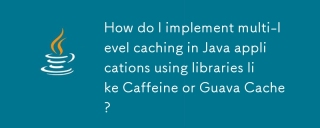
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
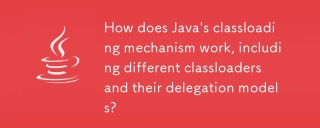
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
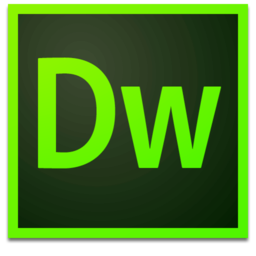
Dreamweaver Mac version
Visual web development tools
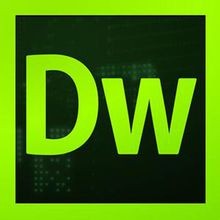
Dreamweaver CS6
Visual web development tools