JavaScript is a widely used scripting language that can be used to develop various types of applications, including web applications, desktop applications, server-side scripts, etc. In these applications, JavaScript is often used to create interactive user interfaces, process data, respond to user events, etc.
In this article, we will introduce a JavaScript library called "onion". The library provides a series of utility functions that help JavaScript developers write code more efficiently.
1. What is Onion
Onion is a lightweight JavaScript library with a functional programming paradigm at its core. It provides many practical functions and components to help developers write code more simply and efficiently.
The core idea of Onion is to allow developers to view programs as a series of nested data processing steps. Each step accepts input data and produces output data, which becomes the input for the next step. This process is like peeling off the layers of an onion, so the library is called "onion".
2. Using onions
Before using onions, we need to install the library first. It can be installed through the npm command line tool:
npm install @zhangmingkai2008/onion
After the installation is complete, we can use the onion library in JavaScript projects. Below, we will introduce some practical functions and components provided by the onion library.
- compose
The compose function can combine multiple functions into one function. These functions will be executed in order from right to left, and the output results will be used as the input of the next function.
For example, we can define three functions:
const add = x => x + 1; const double = x => x * 2; const square = x => x * x;
Then use compose to combine them:
const composed = onion.compose(square, double, add);
Executing the composed function will output the following results:
composed(2); // 返回 36
In the above code, 2 is passed as the input value to the function add, then the double function is executed, and finally the square function is executed. The final output is 36.
- #pipe
The pipe function has the same effect as the compose function, but the order of execution is from left to right. That is, the pipe function executes the first function and then passes its output to the next function.
For example, we can define three functions:
const add = x => x + 1; const double = x => x * 2; const square = x => x * x;
and then use pipe to combine them:
const piped = onion.pipe(add, double, square);
Executing the piped function will output the following results:
piped(2); // 返回 36
The execution order of the compose function is opposite. The add function is executed first, then the double function is executed, and finally the square function is executed. The final output is 36.
- curry
The curry function converts a function that accepts multiple parameters into a series of functions that accepts only one parameter. Each function returns a new function that takes the next argument as input.
For example, we can define a function that accepts three parameters:
const sum = (x, y, z) => x + y + z;
Then use the curry function to convert:
const curriedSum = onion.curry(sum);
Now, we can call curriedSum in the following way Function:
curriedSum(1)(2)(3); // 返回 6 curriedSum(1, 2)(3); // 返回 6 curriedSum(1)(2, 3); // 返回 6
curriedSum Each parameter of the function call returns a new function that accepts the next parameter. What is finally returned is the result of the last function.
- map
#The map function takes an array and a function as input, applies the function to each array element and returns a new array.
For example, we can define an array and a function:
const numbers = [1, 2, 3, 4]; const square = x => x * x;
Then use the map function to apply the function to the array elements:
const squaredNumbers = onion.map(square, numbers);
Now, the squaredNumbers array should be [1, 4, 9, 16].
- #filter
The filter function takes as input an array and a function, applies the function to each array element and returns a new array containing only Those elements for which the function returns true.
For example, we can define an array and a function:
const numbers = [1, 2, 3, 4]; const isEven = x => x % 2 === 0;
Then use the filter function to apply the function to the array elements:
const evenNumbers = onion.filter(isEven, numbers);
Now, the evenNumbers array should be [twenty four].
3. Summary
In this article, we introduced some practical functions and components of the Onion JavaScript library. These functions help JavaScript developers write code more simply and efficiently.
The core idea of Onion is to allow developers to view programs as a series of nested data processing steps. These steps will be peeled off layer by layer, and the output data will become the input of the next step. Using this approach can help developers better organize code and improve code maintainability and readability.
The above is the detailed content of How Onion uses JavaScript. For more information, please follow other related articles on the PHP Chinese website!
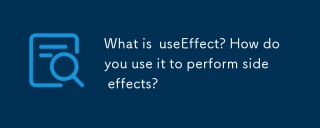
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
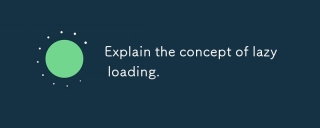
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
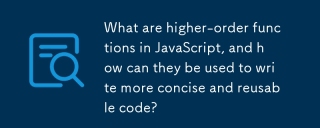
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
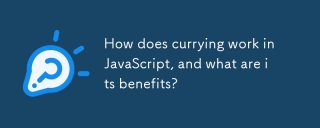
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
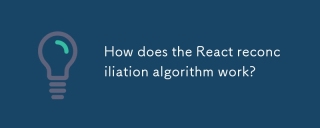
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
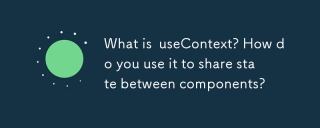
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
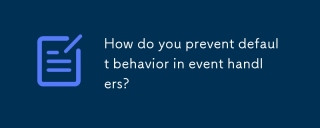
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
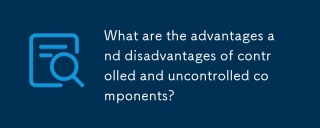
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
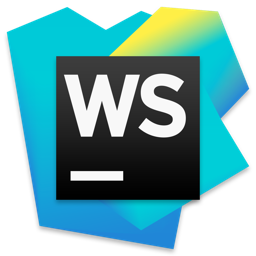
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
