In PHP, we often need to operate on arrays. One common operation is to remove strings from an array. This article will introduce how to use functions and methods in PHP to achieve this operation.
1. Use the function array_filter()
The function array_filter() is a built-in function in PHP that can remove some values from the array. Its usage is as follows:
array_filter(array $input, callable $callback = "?"): array
Among them, the parameter $input is the array that needs to be processed, and the parameter $callback is an optional callback function used to determine which array elements are retained.
The following is an example:
$my_array = [1, 2, 'hello', 'world']; $filtered_array = array_filter($my_array, function($element) { return (!is_string($element)); //返回true表示要保留该元素,返回false表示要去掉该元素 }); print_r($filtered_array); //输出:Array ( [0] => 1 [1] => 2 )
In the above example, $filtered_array only contains integer elements 1 and 2.
2. Use the function array_map()
The function array_map() is also a PHP built-in function. It can convert an array into another array and at the same time automatically perform operations on each element. Define operations. You can use array_map() to remove strings from an array. The specific method is as follows:
array_map(callable $callback , array $array , array ...$arrays): array
Among them, the parameter $callback is a callback function used to operate each element in the array. The parameter $array is the array to be processed, and the parameter $arrays is an optional other array.
The following is an example:
$my_array = [1, 2, 'hello', 'world']; $filtered_array = array_map(function($element) { if(is_string($element)) { //如果是字符串,则返回null,表示要去掉该元素 return null; } else { //否则返回元素本身 return $element; } }, $my_array); print_r($filtered_array); //输出:Array ( [0] => 1 [1] => 2 [2] => [3] => )
In the above example, $filtered_array only contains integer elements 1 and 2.
3. Use array_intersect()
The function array_intersect() is used to compare two or more arrays and return an array containing elements that exist in all arrays. We can use this function to remove strings from an array.
The following is an example:
$my_array = [1, 2, 'hello', 'world']; $filtered_array = array_intersect($my_array, array_filter($my_array, 'is_int')); print_r($filtered_array); //输出:Array ( [0] => 1 [1] => 2 )
In the above example, $filtered_array contains integer elements 1 and 2 that do not contain any strings.
Summary
In PHP, we can use a variety of methods to remove strings from arrays. This article introduces some commonly used methods, including the function array_filter(), the function array_map() and the function array_intersect(). Using these functions can help us perform array operations more conveniently.
The above is the detailed content of How to remove strings from array in php. For more information, please follow other related articles on the PHP Chinese website!
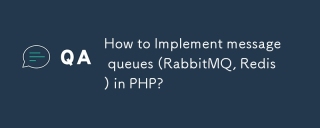
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
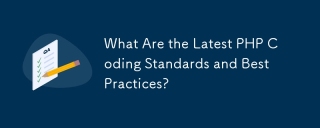
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
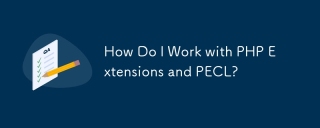
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
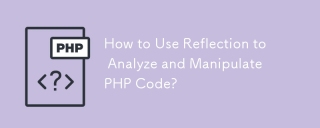
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
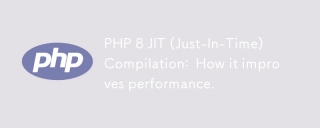
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
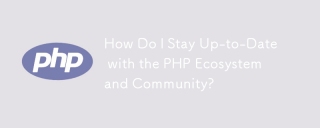
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
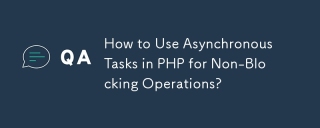
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
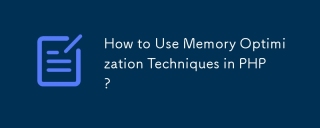
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
