PHP is a widely used programming language that supports processing various types of data, including strings and arrays. In PHP, string array is a common data type that can be used to store multiple string values and perform processing and operations. This article will explain how to handle string arrays in PHP.
- Create a string array
In PHP, you can use the following methods to create a string array:
// 方式一:使用数组初始化器 $array1 = array('string1', 'string2', 'string3'); // 方式二:使用数组初始化器的简写 $array2 = ['string1', 'string2', 'string3']; // 方式三:使用 array() 函数 $array3 = array('string1', 'string2', 'string3');
- Common uses of string arrays Operation
2.1. Access array elements
You can use subscripts to access array elements. Array subscripts in PHP start at 0, so the first element has an index of 0, the second element has an index of 1, and so on. For example:
$array = ['string1', 'string2', 'string3']; echo $array[0]; // 输出 string1 echo $array[1]; // 输出 string2 echo $array[2]; // 输出 string3
2.2. Add elements to the array
You can use the array_push()
function to add elements to the end of the array, or you can directly assign them to the next free one Key:
$array = ['string1', 'string2', 'string3']; // 使用 array_push() 函数 array_push($array, 'string4'); echo $array[3]; // 输出 string4 // 直接赋值 $array[4] = 'string5'; echo $array[4]; // 输出 string5
2.3. Delete elements
You can use the unset()
function to delete any element in the array:
$array = ['string1', 'string2', 'string3']; unset($array[1]); print_r($array); // 输出 Array ( [0] => string1 [2] => string3 )
2.4. Array splicing
You can use the .
operator to join two arrays together:
$array1 = ['string1', 'string2']; $array2 = ['string3', 'string4']; $array3 = $array1 . $array2; print_r($array3); // 输出 Array ( [0] => string1 [1] => string2 [2] => string3 [3] => string4 )
2.5. Array traversal
You can use foreach()
Loop through the elements in the array:
$array = ['string1', 'string2', 'string3']; foreach ($array as $item) { echo $item . "\n"; } // 输出: // string1 // string2 // string3
- Advanced processing of string arrays
3.1. Array sorting
You can use sort ()
Function sorts the array in alphabetical order:
$array = ['string2', 'string1', 'string3']; sort($array); print_r($array); // 输出 Array ( [0] => string1 [1] => string2 [2] => string3 )
3.2. Array filtering
You can use the array_filter()
function to filter the elements in the array. array_filter()
The function iterates through each element in the array and passes it to a callback function for verification. The callback function should return a boolean value indicating whether to retain the element. If the callback function returns true
, the element will be included in the filtered array. For example:
$array = ['string1', '', 'string2', null, 'string3', false]; $array = array_filter($array, function($item) { return !empty($item); }); print_r($array); // 输出 Array ( [0] => string1 [2] => string2 [4] => string3 )
3.3. Array mapping
You can use the array_map()
function to map each element in the array. array_map()
The function will iterate through each element in the array and pass it to a callback function for operation. The callback function should return a new value and then add that value to the new array. For example:
$array = ['string1', 'string2', 'string3']; $array = array_map(function($item) { return strtoupper($item); }, $array); print_r($array); // 输出 Array ( [0] => STRING1 [1] => STRING2 [2] => STRING3 )
- Summary
PHP is a powerful programming language that provides many functions and operations for working with string arrays. In this article, we covered some common string array operations, including creating, accessing, adding, deleting, concatenating, traversing, sorting, filtering, and mapping. Using these operations, we can easily process and manage string arrays in PHP to achieve various functional needs.
The above is the detailed content of How to process string array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
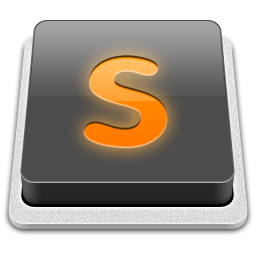
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
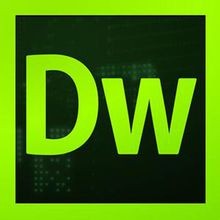
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
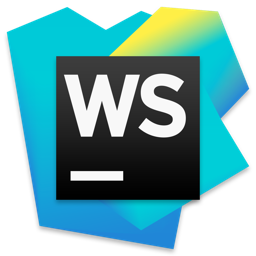
WebStorm Mac version
Useful JavaScript development tools
