Node.js is a JavaScript running environment based on the Chrome V8 engine, which can run JavaScript code on the server side. Node.js is widely used in both front-end and back-end development. However, in some applications, database access is required, which makes Node.js database query operations extremely important.
In this article, we will learn how to use Node.js to connect to the database, perform database query operations, and how to handle asynchronous operations through callback functions.
1. Connect to the database
In Node.js, we can use multiple libraries to connect and operate different types of databases. We first need to import the required libraries by installing dependencies in order to connect to the required database.
The following is a sample code to connect to the MySQL database:
const mysql = require('mysql'); const connection = mysql.createConnection({ host : 'localhost', user : 'root', password : 'password', database : 'database_name' }); connection.connect(function(err) { if (err) throw err; console.log('Connected to MySQL database!'); });
In the above code, we use the mysql library to connect to the MySQL database. The parameters of the createConnection method include the user name, password, database name and host name to connect to the database. After the connection is successful, we will get a successful log message.
We can also use databases such as MongoDB, PostgreSQL and SQLite by simply changing the library's dependencies and related connection information.
2. Perform database query operations
Once we successfully connect to the database, we can start querying data. We can query one piece of data in the database or multiple pieces of data. Below, we can see how to perform basic queries:
- Query a single piece of data
We can query a single piece of data through parameters such as ID. The following is a basic query code example:
const mysql = require('mysql'); const connection = mysql.createConnection({ host : 'localhost', user : 'root', password : '', database : 'mydatabase' }); connection.connect(); connection.query('SELECT * FROM customers WHERE id = 1', function (err, result, fields) { if (err) throw err; console.log(result); }); connection.end();
In the above code, we use the SELECT statement to query the customer information with id 1, and print the results in the callback function.
- Query multiple pieces of data
Sometimes we need to query all the data in the database or the data that meets some specific conditions. We can use the SELECT statement to query multiple pieces of data. The following is a sample code:
const mysql = require('mysql'); const connection = mysql.createConnection({ host : 'localhost', user : 'root', password : '', database : 'mydatabase' }); connection.connect(); connection.query('SELECT * FROM customers', function (err, result, fields) { if (err) throw err; console.log(result); }); connection.end();
In the above code, we query and print all customer information in the customers table.
3. Node.js asynchronous operations
In Node.js, all I/O operations are performed asynchronously. This means that when we perform a query operation, we cannot know exactly when the query will complete. To do this, we need to use a callback function to listen to the asynchronous operation and process the data in the callback function.
In the above sample code, we use a callback function to process the results of the query operation. When the query is successful, we use the result parameter passed in the callback function to iterate over the results of the query and print it to the console.
4. Conclusion
In this article, we learned how to use Node.js to connect, query and operate the database. Specifically, we learned how to use different databases such as MySQL, MongoDB, and PostgreSQL, among others. We also learned how to perform basic queries and handle asynchronous operations through callback functions.
Using Node.js to operate the database can provide faster query results and more efficient operation methods. It can also help us complete tasks better in front-end and back-end development. I hope this article will be helpful for you to learn Node.js database operations.
The above is the detailed content of How to connect to a database using Node.js. For more information, please follow other related articles on the PHP Chinese website!

No,youshouldn'tusemultipleIDsinthesameDOM.1)IDsmustbeuniqueperHTMLspecification,andusingduplicatescancauseinconsistentbrowserbehavior.2)Useclassesforstylingmultipleelements,attributeselectorsfortargetingbyattributes,anddescendantselectorsforstructure

HTML5aimstoenhancewebcapabilities,makingitmoredynamic,interactive,andaccessible.1)Itsupportsmultimediaelementslikeand,eliminatingtheneedforplugins.2)Semanticelementsimproveaccessibilityandcodereadability.3)Featureslikeenablepowerful,responsivewebappl

HTML5aimstoenhancewebdevelopmentanduserexperiencethroughsemanticstructure,multimediaintegration,andperformanceimprovements.1)Semanticelementslike,,,andimprovereadabilityandaccessibility.2)andtagsallowseamlessmultimediaembeddingwithoutplugins.3)Featur

HTML5isnotinherentlyinsecure,butitsfeaturescanleadtosecurityrisksifmisusedorimproperlyimplemented.1)Usethesandboxattributeiniframestocontrolembeddedcontentandpreventvulnerabilitieslikeclickjacking.2)AvoidstoringsensitivedatainWebStorageduetoitsaccess

HTML5aimedtoenhancewebdevelopmentbyintroducingsemanticelements,nativemultimediasupport,improvedformelements,andofflinecapabilities,contrastingwiththelimitationsofHTML4andXHTML.1)Itintroducedsemantictagslike,,,improvingstructureandSEO.2)Nativeaudioand

Using ID selectors is not inherently bad in CSS, but should be used with caution. 1) ID selector is suitable for unique elements or JavaScript hooks. 2) For general styles, class selectors should be used as they are more flexible and maintainable. By balancing the use of ID and class, a more robust and efficient CSS architecture can be implemented.

HTML5'sgoalsin2024focusonrefinementandoptimization,notnewfeatures.1)Enhanceperformanceandefficiencythroughoptimizedrendering.2)Improveaccessibilitywithrefinedattributesandelements.3)Addresssecurityconcerns,particularlyXSS,withwiderCSPadoption.4)Ensur

HTML5aimedtoimprovewebdevelopmentinfourkeyareas:1)Multimediasupport,2)Semanticstructure,3)Formcapabilities,and4)Offlineandstorageoptions.1)HTML5introducedandelements,simplifyingmediaembeddingandenhancinguserexperience.2)Newsemanticelementslikeandimpr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
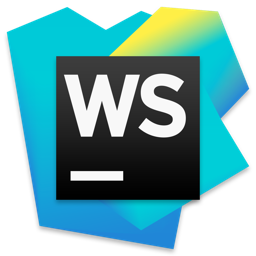
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
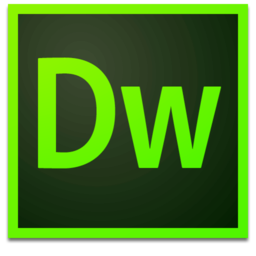
Dreamweaver Mac version
Visual web development tools
