In PHP, it is often necessary to determine whether a variable is an array, which can be achieved through built-in functions. This article will introduce three PHP functions to determine whether a variable is an array.
- is_array function: The is_array function is the most basic function provided by PHP to determine whether a variable is an array. This function accepts one parameter, which is the variable to check, and returns true if the variable is an array, otherwise it returns false. The code example is as follows:
$arr = array(1, 2, 3); if (is_array($arr)) { echo '$arr是数组'; } else { echo '$arr不是数组'; }
- gettype function: The gettype function is a function provided by PHP to obtain the variable type. This function accepts one parameter, the variable to be checked, and returns the type of the variable. If the variable is an array, returns 'array'. The code example is as follows:
$arr = array(1, 2, 3); if (gettype($arr) == 'array') { echo '$arr是数组'; } else { echo '$arr不是数组'; }
- is_iterable function in PHP 7: The is_iterable function is a new function introduced in PHP 7 for checking whether a variable is iterable. This function accepts one parameter, which is the variable to be checked, and returns true if the variable is an array or an object that implements the Traversable interface, otherwise it returns false. The sample code for using the is_iterable function to determine whether a variable is an array is as follows:
$arr = array(1, 2, 3); if (is_iterable($arr)) { echo '$arr是数组'; } else { echo '$arr不是数组'; }
No matter which method is used, it is very simple to determine whether a variable is an array in PHP. Through these functions, we can easily determine whether a variable is an array, thereby ensuring that our code executes correctly.
The above is the detailed content of What is the function in php to determine whether it is an array?. For more information, please follow other related articles on the PHP Chinese website!
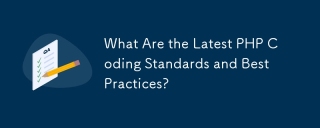
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
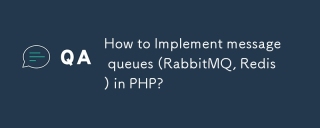
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
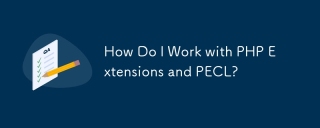
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
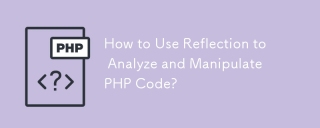
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
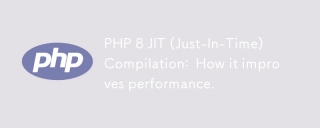
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
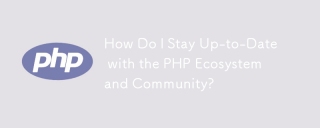
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
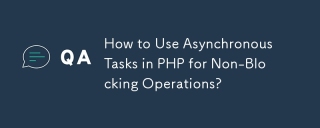
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
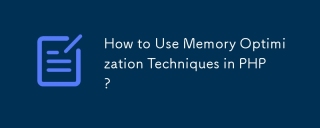
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
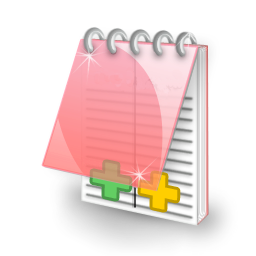
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
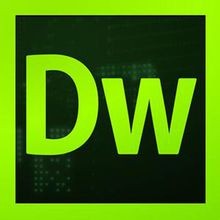
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
