What——What is a weak reference?
The weak reference in Java specifically refers to the java.lang.ref.WeakReference
The existence of a weak reference object will not prevent the object it points to from being recycled by the garbage collector. The most common use of weak references is to implement canonicalizing mappings (such as hash tables).
Assume that the garbage collector determines at a certain point in time that an object is weakly reachable (that is, all current points pointing to it are weak references), then the garbage collector will Clear all weak references to the object, and then mark the weakly reachable object as finalizable so that it will be recycled later. At the same time or later, the garbage collector will put the weak references that have just been cleared into the reference queue (Reference Queue) specified when creating the weak reference object.
Actually, there are four types of references in Java, which from strong to weak are: strong references, soft references, weak references, and virtual references. Below we briefly introduce the other three types of references except weak references:
Strong Reference: Usually when we create a new object through new, the reference returned is a strong reference. Reference, if an object is reachable through a series of strong references, it is strongly reachable, then it will not be recycled
Soft Reference: Soft Reference The difference between a reference and a weak reference is that if an object is reachable by a weak reference, it will be recycled regardless of whether the current memory is sufficient, while an object that is reachable by a soft reference will be recycled when the memory is insufficient, so a soft reference is better than a weak reference. References are "stronger"
Phantom Reference: Phantom Reference is the weakest reference in Java, so how weak is it? It is so fragile that we cannot even get the referenced object through a virtual reference. The only purpose of a virtual reference is that when the object it points to is recycled, the virtual reference itself will be added to the reference queue to record it. The pointed object has been recycled.
Why——Why use weak references?
Consider the following scenario: There is now a Product class representing a product. This class is designed to be non-extensible, and at this time we want to add a number to each product. One solution is to use HashMap
So in this case, if we want to truly recycle a Product object, it is not enough to just assign its strong reference to null, but also to remove the corresponding entry from the HashMap. Obviously we don't want to complete the work of "removing no longer needed entries from HashMap" ourselves. We want to tell the garbage collector that when only the keys in the HashMap refer to the Product object, the corresponding Product object can be recycled. Obviously, according to the previous definition of weak reference, using weak references can help us achieve this goal. We only need to use a weak reference object pointing to the Product object as the key in the HashMap.
How——How to use weak references?
Take the scenario introduced above as an example. We use a weak reference object pointing to the Product object as the key of HashMap. We only need to define this weak reference object like this:
Product productA = new Product(...);
WeakReferenceweakProductA = new WeakReference(productA);
Now, if the object weakProductA is referenced It points to the Product object productA. So how do we get the Product object productA it points to through weakProduct? It's very simple. You only need the following code:
Product product = weakProductA.get();
In fact, for this case, the Java class The library provides us with the WeakHashMap class. When using this class, its key is naturally a weak reference object, and we do not need to manually wrap the original object. In this way, when productA becomes null (indicating that the Product it refers to no longer needs to exist in memory), then the weak reference object weakProductA points to the Product object, so obviously the corresponding Product object is weak at this time. Reachable, so the weak reference pointing to it will be cleared, the Product object will be recycled immediately, and the weak reference object pointing to it will enter the reference queue.
Reference Queue
Let’s briefly introduce the concept of reference queue. In fact, the WeakReference class has two constructors:
//创建一个指向给定对象的弱引用 WeakReference(T referent) //创建一个指向给定对象并且登记到给定引用队列的弱引用 WeakReference(T referent, ReferenceQueue super T> q)
We can see that the second constructor provides a parameter of type ReferenceQueue. By providing this parameter, we register the created weak reference object. to a reference queue, so that when it is cleared by the garbage collector, it will be sent to the reference queue, and we can uniformly manage these cleared weak reference objects.
The above is the detailed content of Learning and understanding of Java weak references. For more information, please follow other related articles on the PHP Chinese website!
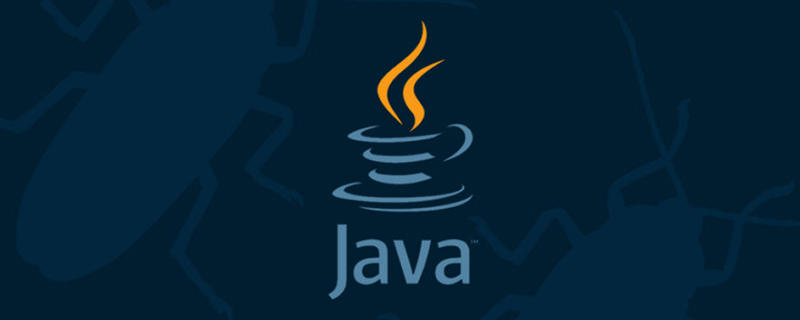
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
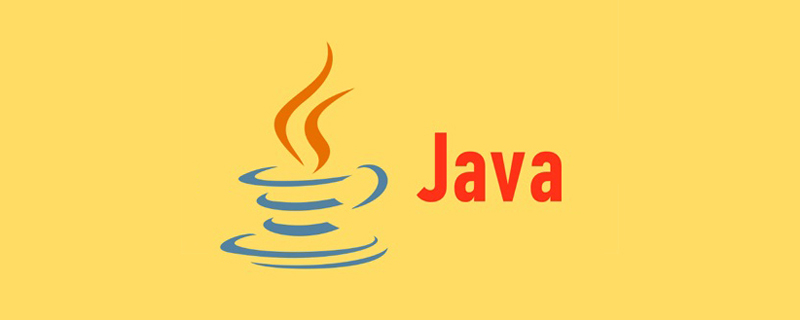
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
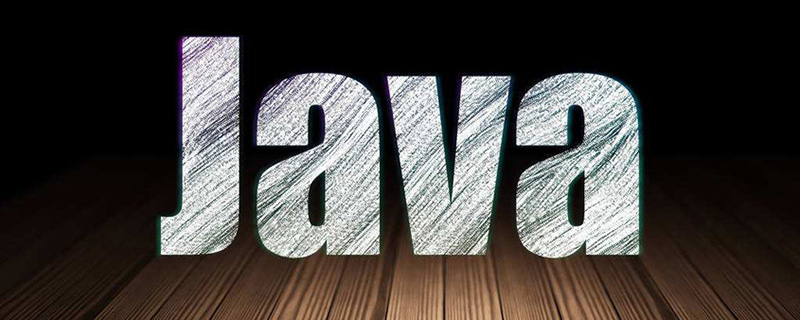
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
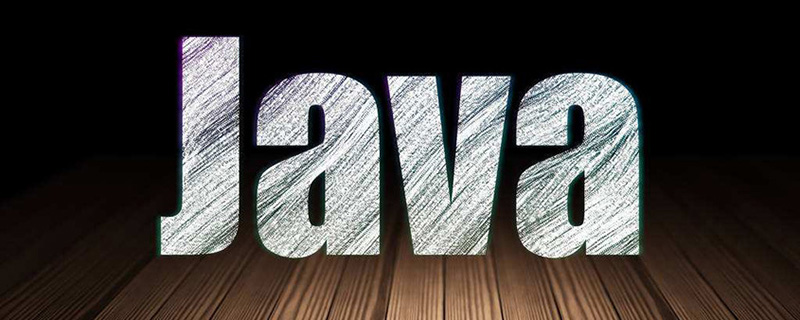
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
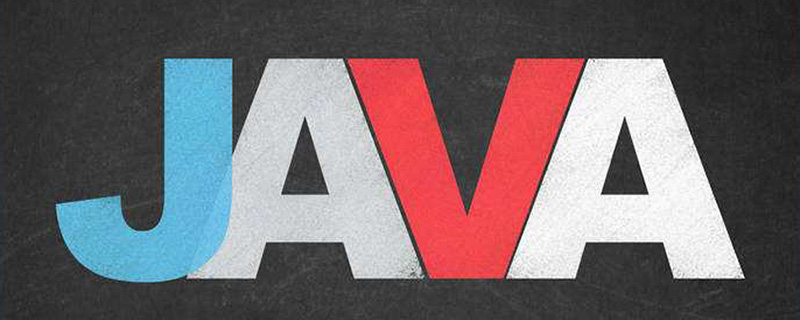
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
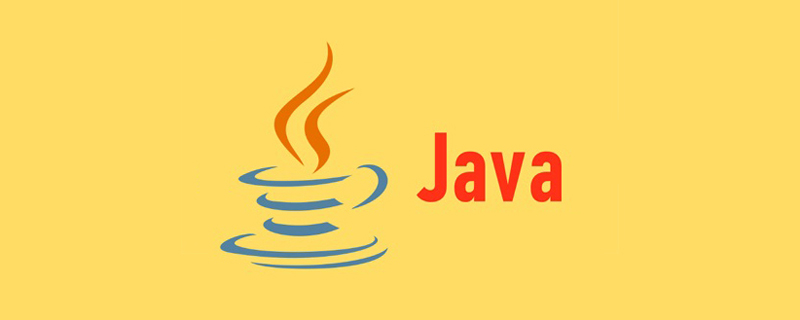
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
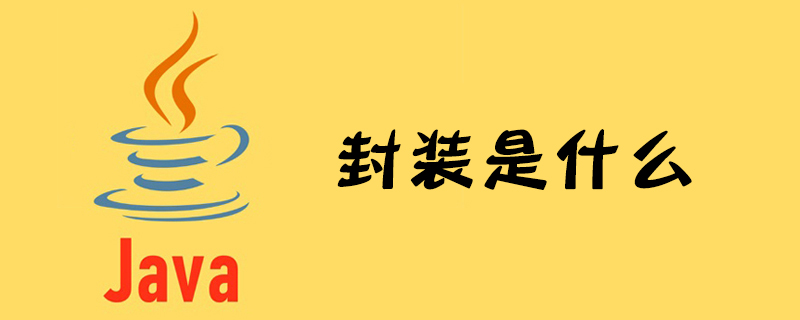
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
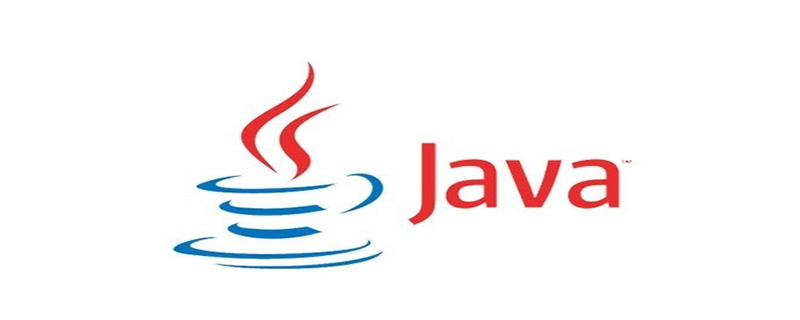
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
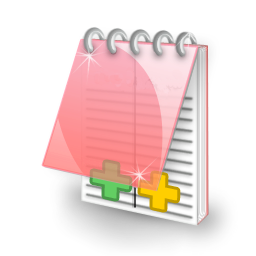
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
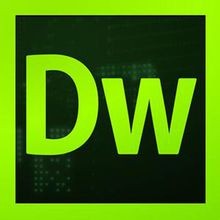
Dreamweaver CS6
Visual web development tools
