Golang is an efficient, concise and fast programming language. It is gradually favored by major enterprises, and more and more developers are beginning to use it for development. In Golang, we can use static methods to simplify the code and improve the readability and maintainability of the program. This article will introduce how to use static methods in Golang and how to write static methods elegantly.
1. What is a static method
In Golang, a static method refers to a function defined on a type. These functions are independent of type instances and we can call them directly using the type name. Compared with static methods in classes, static methods in Golang are more elegant and concise.
2. How to define a static method
In Golang, to define a static method, you need to add a specific keyword - "func" before the function, and add the type name before the function name. For example:
type User struct { Name string } func (u User) SayHello() { fmt.Printf("Hello, my name is %s", u.Name) } func SayBye() { fmt.Println("Bye") }
In the above code, we define a type User and define a method SayHello() on it. At this point, each User instance will have this method. In addition to this, we also define a static method SayBye() in the global scope. This method has nothing to do with the User type, we can call it directly using the function name.
3. How to call static methods
There is a certain difference between calling static methods and calling instance methods. In the instance method, we need to first create an instance, and then call the method through the instance name:
user := User{"Alice"} user.SayHello()
And in the static method, we directly use the type name to call the method:
SayBye()
Four , How to write static methods elegantly
The writing of static methods needs to be as elegant and concise as possible, so that we can fully reflect the beauty and simplicity of Golang in the code. Here are some noteworthy tips for writing static methods.
- Consider the type of receiving object
When writing a static method, we should consider the type of receiving object. If a method only takes its own state and does not need its own behavior, you can consider defining it as a static method. In this case, it should not access instance variables and need not be associated with any instance of the struct.
type User struct { Name string Age int } func NewUser(name string) *User { return &User{ Name: name, } }
In the above code, we define a static method NewUser(), which receives a parameter name and returns a newly created User instance. This method is only relevant to the User type and does not depend on any specific User instance.
- Avoid too many magic variables
When writing static methods, we should try to avoid using too many magic variables, which may increase the complexity of the code and readability. For example:
func Random(min, max int) int { return rand.Intn(max-min) + min }
In the above code, we define a static method Random(), which receives two parameters: the minimum value and the maximum value. This method uses the built-in rand package to generate a random number and return it. This method only contains one magic variable - rand, because we can regenerate this random number at any time. If we use more magic variables, the code will become more difficult to understand, so we should try to avoid this situation.
- Use default parameter values
In Golang, we can use default parameter values to simplify the writing of static methods. For example:
func LoadConfig(path string, retries int) { if retries == 0 { retries = 5 } }
In the above code, we define a static method LoadConfig(), which receives two parameters - the configuration file path path and the number of retries retries. If no retries parameter is passed when calling this method, it will default to 5. Using this approach avoids writing too many branch statements and improves code readability.
Summary
Static method is an excellent programming technology in Golang, which can help us simplify the code and improve the readability and maintainability of the program. When writing static methods, you need to pay attention to avoid using too many magic variables, consider the type of the receiving object, and use default parameter values. I hope this article can provide you with some reference and inspiration for writing excellent static methods in Golang.
The above is the detailed content of How to use static methods in Golang. For more information, please follow other related articles on the PHP Chinese website!
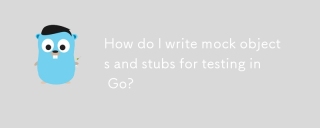
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
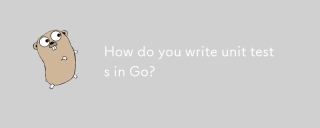
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
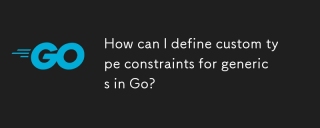
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
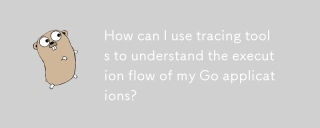
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
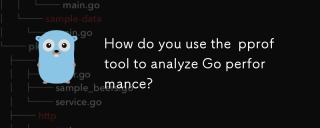
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
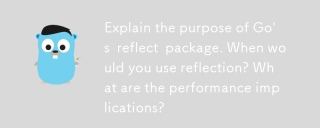
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
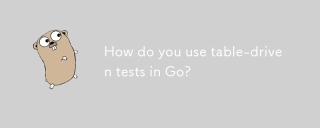
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
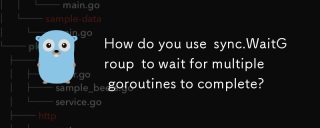
The article explains how to use sync.WaitGroup in Go to manage concurrent operations, detailing initialization, usage, common pitfalls, and best practices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
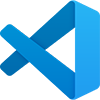
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
