With the development of the Internet, registration has become a task that many people must complete. It has become very common to register on websites and applications, and these registration processes require users to fill in a lot of personal information, such as username, password, email address, etc. In order to ensure the reliability and security of this information, we need to verify the registration information. In web development, JavaScript validation is widely used. In this article, we will discuss how to use JavaScript to verify registration information and add some special features.
Generally speaking, the front-end form validation process is triggered when it gains focus and loses focus. By using JavaScript, we can pop up a prompt message when the focus is gained, and perform corresponding verification when the focus is lost.
First, in the HTML markup, we need to create a form that contains the form element. The form element should contain an input field and a submit button. In this example, we will use a simple registration form as an example.
We can see some new attributes in the form element, such as "required" and "onsubmit". Among them, "required" means that the input box must be filled in before the form can be submitted, and "onsubmit" means the JavaScript function to be executed before submitting the form.
Next, we need to implement a JavaScript function to validate the form data. In this example, we will implement a function called validateForm(). This function will take the data entered in the form and do some validation.
function validateForm() { // Get the input data var username = document.forms["registerForm"]["userName"].value; var password = document.forms["registerForm"]["password"].value; var repassword = document.forms["registerForm"]["rePassword"].value; var email = document.forms["registerForm"]["email"].value; // Check username if (username == "") { alert("用户名不能为空!"); document.forms["registerForm"]["userName"].focus(); return false; } // Check password if (password == "") { alert("密码不能为空!"); document.forms["registerForm"]["password"].focus(); return false; } // Check confirm password if (repassword == "") { alert("确认密码不能为空!"); document.forms["registerForm"]["rePassword"].focus(); return false; } else { if (repassword != password) { alert("两次密码不匹配!"); document.forms["registerForm"]["password"].value = ""; document.forms["registerForm"]["rePassword"].value = ""; document.forms["registerForm"]["password"].focus(); return false; } } // Check email var emailReg = /^([\w-]+\.)*?[\w-]+@[\w-]+\.([\w-]+\.)*?[\w]+$/; if (email == "") { alert("电子邮件不能为空!"); document.forms["registerForm"]["email"].focus(); return false; } else if (!emailReg.test(email)) { alert("电子邮件格式不正确!"); document.forms["registerForm"]["email"].value = ""; document.forms["registerForm"]["email"].focus(); return false; } // If all the data is correct, return true return true; }
In this function, we use some simple validation conditions to check the correctness of the form data. For example, check if the username is empty, check if the password and confirm password are the same, check if the email is in the correct format, etc. If the input data does not meet the requirements, a warning message will pop up and false will be returned to prevent form submission. Returns true if all data meets the requirements.
Next, we need to add a JS function to perform corresponding operations on the input box gaining focus and losing focus.
// Add onfocus event document.getElementsByName("userName")[0].onfocus = function() { document.getElementsByName("userName")[0].nextElementSibling.innerHTML = "请输入用户名"; }; document.getElementsByName("password")[0].onfocus = function() { document.getElementsByName("password")[0].nextElementSibling.innerHTML = "请输入密码"; }; document.getElementsByName("rePassword")[0].onfocus = function() { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = "请再次输入密码"; }; document.getElementsByName("email")[0].onfocus = function() { document.getElementsByName("email")[0].nextElementSibling.innerHTML = "请输入电子邮件地址"; }; // Add onblur event document.getElementsByName("userName")[0].onblur = function() { if (document.getElementsByName("userName")[0].value == "") { document.getElementsByName("userName")[0].nextElementSibling.innerHTML = "用户名不能为空!"; } else { document.getElementsByName("userName")[0].nextElementSibling.innerHTML = ""; } }; document.getElementsByName("password")[0].onblur = function() { if (document.getElementsByName("password")[0].value == "") { document.getElementsByName("password")[0].nextElementSibling.innerHTML = "密码不能为空!"; } else { document.getElementsByName("password")[0].nextElementSibling.innerHTML = ""; } }; document.getElementsByName("rePassword")[0].onblur = function() { if (document.getElementsByName("rePassword")[0].value == "") { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = "确认密码不能为空!"; } else if (document.getElementsByName("rePassword")[0].value != document.getElementsByName("password")[0].value) { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = "两次密码不匹配!"; } else { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = ""; } }; document.getElementsByName("email")[0].onblur = function() { var email = document.getElementsByName("email")[0].value; var emailReg = /^([\w-]+\.)*?[\w-]+@[\w-]+\.([\w-]+\.)*?[\w]+$/; if (email == "") { document.getElementsByName("email")[0].nextElementSibling.innerHTML = "电子邮件不能为空!"; } else if (!emailReg.test(email)) { document.getElementsByName("email")[0].nextElementSibling.innerHTML = "电子邮件格式不正确!"; } else { document.getElementsByName("email")[0].nextElementSibling.innerHTML = ""; } };
In these codes, we have added event handlers for each input field. When the focus is obtained, a prompt message is displayed; after the focus is lost, the verification result is displayed. For example, when entering a user name, "Please enter user name" will be displayed next to the text box, and a specific error message will be displayed when the input is incorrect.
Finally, we can put these codes together to form a complete example:
<script> // Add onfocus event document.getElementsByName("userName")[0].onfocus = function() { document.getElementsByName("userName")[0].nextElementSibling.innerHTML = "请输入用户名"; }; document.getElementsByName("password")[0].onfocus = function() { document.getElementsByName("password")[0].nextElementSibling.innerHTML = "请输入密码"; }; document.getElementsByName("rePassword")[0].onfocus = function() { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = "请再次输入密码"; }; document.getElementsByName("email")[0].onfocus = function() { document.getElementsByName("email")[0].nextElementSibling.innerHTML = "请输入电子邮件地址"; }; // Add onblur event document.getElementsByName("userName")[0].onblur = function() { if (document.getElementsByName("userName")[0].value == "") { document.getElementsByName("userName")[0].nextElementSibling.innerHTML = "用户名不能为空!"; } else { document.getElementsByName("userName")[0].nextElementSibling.innerHTML = ""; } }; document.getElementsByName("password")[0].onblur = function() { if (document.getElementsByName("password")[0].value == "") { document.getElementsByName("password")[0].nextElementSibling.innerHTML = "密码不能为空!"; } else { document.getElementsByName("password")[0].nextElementSibling.innerHTML = ""; } }; document.getElementsByName("rePassword")[0].onblur = function() { if (document.getElementsByName("rePassword")[0].value == "") { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = "确认密码不能为空!"; } else if (document.getElementsByName("rePassword")[0].value != document.getElementsByName("password")[0].value) { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = "两次密码不匹配!"; } else { document.getElementsByName("rePassword")[0].nextElementSibling.innerHTML = ""; } }; document.getElementsByName("email")[0].onblur = function() { var email = document.getElementsByName("email")[0].value; var emailReg = /^([\w-]+\.)*?[\w-]+@[\w-]+\.([\w-]+\.)*?[\w]+$/; if (email == "") { document.getElementsByName("email")[0].nextElementSibling.innerHTML = "电子邮件不能为空!"; } else if (!emailReg.test(email)) { document.getElementsByName("email")[0].nextElementSibling.innerHTML = "电子邮件格式不正确!"; } else { document.getElementsByName("email")[0].nextElementSibling.innerHTML = ""; } }; function validateForm() { // Get the input data var username = document.forms["registerForm"]["userName"].value; var password = document.forms["registerForm"]["password"].value; var repassword = document.forms["registerForm"]["rePassword"].value; var email = document.forms["registerForm"]["email"].value; // Check username if (username == "") { alert("用户名不能为空!"); document.forms["registerForm"]["userName"].focus(); return false; } // Check password if (password == "") { alert("密码不能为空!"); document.forms["registerForm"]["password"].focus(); return false; } // Check confirm password if (repassword == "") { alert("确认密码不能为空!"); document.forms["registerForm"]["rePassword"].focus(); return false; } else { if (repassword != password) { alert("两次密码不匹配!"); document.forms["registerForm"]["password"].value = ""; document.forms["registerForm"]["rePassword"].value = ""; document.forms["registerForm"]["password"].focus(); return false; } } // Check email var emailReg = /^([\w-]+\.)*?[\w-]+@[\w-]+\.([\w-]+\.)*?[\w]+$/; if (email == "") { alert("电子邮件不能为空!"); document.forms["registerForm"]["email"].focus(); return false; } else if (!emailReg.test(email)) { alert("电子邮件格式不正确!"); document.forms["registerForm"]["email"].value = ""; document.forms["registerForm"]["email"].focus(); return false; } // If all the data is correct, return true return true; } </script>The above is the process of verifying registration information using JavaScript. Through this example, we can see that front-end verification can greatly improve user experience and data security. Therefore, in web development, front-end form verification and back-end data verification go hand in hand to achieve a complete verification system.
The above is the detailed content of Discuss how to use JavaScript to verify registration information. For more information, please follow other related articles on the PHP Chinese website!

To integrate React into HTML, follow these steps: 1. Introduce React and ReactDOM in HTML files. 2. Define a React component. 3. Render the component into HTML elements using ReactDOM. Through these steps, static HTML pages can be transformed into dynamic, interactive experiences.

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
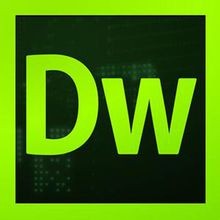
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
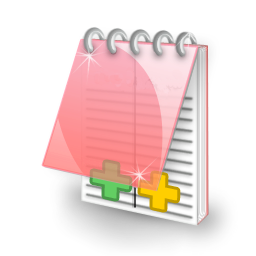
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.