In PHP, arrays are a very important data type, they allow a group of related data items to be stored in the same variable. Using arrays can greatly improve the readability and maintainability of your code. However, in actual programming, it is often necessary to operate on arrays, such as finding the difference between two arrays. This article will explore how to compare two different arrays in PHP.
First, let's create two different arrays to better demonstrate how to compare them:
$array1 = array("apple", "banana", "orange", "pear"); $array2 = array("banana", "orange", "grapefruit");
Let's look at the differences between these two arrays. We can use the PHP built-in function array_diff() to find the differences between two arrays. The syntax of this function is as follows:
array_diff(array1, array2, array3...)
Among them, array1, array2, array3, etc. are the arrays to be compared, and there can be multiple arrays.
Now, let us use the array_diff() function to find the difference between $array1 and $array2. The code is as follows:
$diff = array_diff($array1, $array2); print_r($diff);
The output result is as follows:
Array ( [0] => apple [3] => pear )
We can see that the output result is the difference between the two arrays. "apple" and "pear" in $array1 are in Does not exist in $array2.
What if we want to find the difference between $array2 and $array1? We only need to exchange the order of the arrays in the above code. The code is as follows:
$diff = array_diff($array2, $array1); print_r($diff);
The output result is as follows:
Array ( [2] => grapefruit )
We can see that the output result is the difference between the two arrays. "grapefruit" in $array2 is not in $array1 exist.
In actual programming, you may encounter the situation of comparing the differences between multiple arrays. At this point, we can use a variant of PHP's built-in function array_diff(): array_diff_key() to compare key-value pairs between multiple arrays.
The syntax is as follows:
array_diff_key(array1, array2, array3...)
Now, let’s look at an example. We create three arrays as follows:
$array1 = array("name" => "Tom", "age" => 20, "gender" => "male"); $array2 = array("name" => "Jack", "age" => 22, "gender" => "male", "hobby" => "swimming"); $array3 = array("name" => "Alice", "age" => 24, "gender" => "female", "hobby" => "reading");
We can use the array_diff_key() function to find the differences between the above three arrays. The code is as follows:
$diff = array_diff_key($array1, $array2, $array3); print_r($diff);
The output result is as follows:
Array ( [name] => Tom [age] => 20 [gender] => male )
We can see that the output result is the difference between the three arrays. The key-value pairs in $array1 are in $array2 and $ Does not exist in array3.
Finally, let’s talk about what to do if you need to find the same elements in two arrays at the same time. At this time, we can use the PHP built-in function array_intersect(). The syntax of this function is as follows:
array_intersect(array1, array2, array3...)
Among them, array1, array2, array3, etc. are the arrays to be compared, and there can be multiple arrays.
Now, let’s look at an example. We create two arrays as follows:
$array1 = array("apple", "banana", "orange", "pear"); $array2 = array("banana", "orange", "grapefruit");
We can use the array_intersect() function to find the same elements between the above two arrays. The code is as follows:
$intersect = array_intersect($array1, $array2); print_r($intersect);
The output result is as follows:
Array ( [1] => banana [2] => orange )
We can see that the output result is the common element between the two arrays, namely "banana" and "orange".
The above is how to compare different PHP arrays. In actual programming, we need to choose which method to use for array calculations based on specific needs.
The above is the detailed content of How to compare two different arrays in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
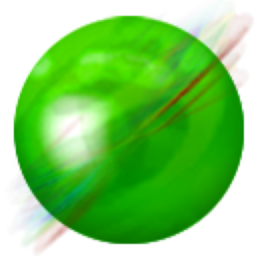
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
