In front-end development, we often need to use JavaScript to achieve some interactive effects and logical processing. Among them, modifying and saving data is also one of the common requirements. This article will introduce how to use JavaScript to modify and save data.
1. Overview
In common websites and applications, users usually need to change content or manipulate data. These changed content or data need to be saved to the back-end server or local hard drive. Therefore, we need to use JavaScript to call the corresponding API to implement the data saving function.
2. Data types
Before realizing data saving, we need to know how different types of data should be processed. Normally, we can divide data into the following two categories:
- Form data: Form data is usually form data filled in by users, such as text boxes, drop-down boxes, radio button boxes, etc. This data can be obtained through JavaScript's
document.forms
property, then processed in JSON format, and then sent to the backend server through AJAX for saving. - Local data types: Local data types include Cookie, Web Storage, IndexedDB, etc. This data can be used to save user login information, application settings, business data, etc.
3. Form data storage
Form data is one of the most common types. We can use JavaScript and AJAX to send form data to the back-end server. The following is an example:
document.getElementById("saveButton").addEventListener("click", function(){ var form = document.forms["myForm"]; var formData = new FormData(form); var xhr = new XMLHttpRequest(); xhr.open("POST", "/saveData.php"); xhr.send(formData); xhr.onreadystatechange = function() { if(xhr.readyState === 4 && xhr.status === 200) { console.log(xhr.responseText); } } });
In this example, we use the addEventListener method to add a click event. This event sends the form data to the /saveData.php file through the POST method of the XMLHttpRequest object. When the server returns a response, we print the text it returned on the console.
4. Web Storage Save
Web Storage is a type of browser local storage API. It can be accessed through localStorage and sessionStorage objects. The following is an example:
// 存储数据 localStorage.setItem("username", "Jack"); localStorage.setItem("age", "25"); // 读取数据 var username = localStorage.getItem("username"); var age = localStorage.getItem("age"); // 删除数据 localStorage.removeItem("username"); localStorage.clear(); // 删除所有数据
The above code demonstrates how to use localStorage to store and read data. This data will remain in the browser until manually cleared or until the storage limit is reached.
5. Summary
This article introduces the method of using JavaScript to modify and save data, including form data saving and Web Storage storage. These technologies can greatly improve user experience and improve application performance and efficiency. Of course, in practical applications, we need to choose the most appropriate data type and storage method according to specific scenarios to meet user needs.
The above is the detailed content of How to use JavaScript to modify and save data. For more information, please follow other related articles on the PHP Chinese website!
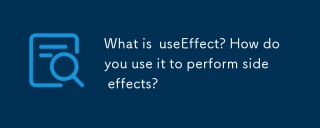
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
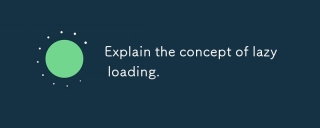
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
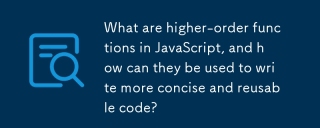
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
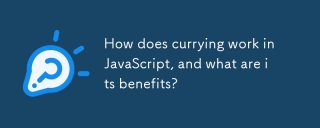
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
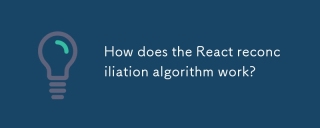
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
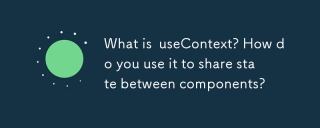
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
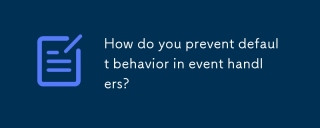
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
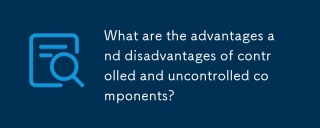
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
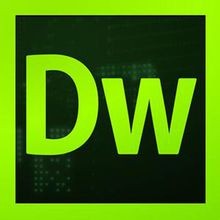
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
