What does comma mean in JavaScript
There are many operators in JavaScript, and comma is one of them. Commas in JavaScript behave a little differently than commas in other languages, and have some unique uses and syntax rules.
Basic usage of JavaScript commas
When comma is used as an operator, its function is to connect two or more operands and return the result value of the last operand. When a comma is used in an expression, its result value is the result of the last expression on the right.
For example, the comma operator is used in the following code snippet:
let a = 1, b = 2, c = 3; let d = (a + b, c * 2); console.log(d); // 6
In this example, the first line of code uses commas to declare three variables on the same line. The second line of code uses a comma to concatenate the addition and multiplication expressions and then assigns the result to the variable d. Finally, the console.log() function outputs the value of variable d as 6.
In actual development, the comma operator is often used to simplify code and reduce variable declarations. The following code shows how to use commas to connect multiple expressions to achieve the purpose of multiple operations:
for (let i = 0, j = 10; i <p>In this example, use comma separators to separate the initialization, loop conditions and self of i and j. Concatenate increment/decrement expressions. In this way, multiple operations can be implemented simultaneously in one line of code, helping us achieve more concise and efficient code. </p><p>Application of commas in function parameters and arrays</p><p>In JavaScript, the comma operator can also be used in function parameters and arrays. </p><p>In function parameters, commas can be used to connect multiple parameters. Although JavaScript functions have no limit on the number of parameters, in order to improve the readability of the code, it is generally recommended not to use too many commas in function parameters. The following example shows how to use commas to link multiple parameters: </p><pre class="brush:php;toolbar:false">function multiply(a, b, c) { return a * b * c; } let result = multiply(2, 3, 4); console.log(result); // 24
In this example, commas are used to link three parameters so that the function multiply() returns the product of the three parameters.
In an array, a comma represents a separator for the array. Comma separator is used to separate the elements of an array, it separates each element in the array and places them in an array object. The following code shows the use of comma separator in an array:
let myArray = [1, 2, 3, 4, 5]; console.log(myArray); // [1, 2, 3, 4, 5]
In this example, the comma operator is used to separate each element in the array so that they are placed in an array object.
Summary
Comma, as an operator in JavaScript, can be used to link multiple operations and return the result of the last operation. It can also be used as a delimiter in function parameters and arrays. Although commas have many uses in JavaScript, you need to avoid overuse when using them to avoid adversely affecting the readability and maintainability of your code. Proper use of commas can help us achieve more concise and efficient code.
The above is the detailed content of What does a javascript comma mean?. For more information, please follow other related articles on the PHP Chinese website!

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
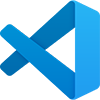
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software