1.API
1.1API Overview
API (Application Programming Interface): Application Programming Interface
in java refers to the one provided in the JDK Java classes with various functions encapsulate the underlying implementation. We don't need to care about how these classes are implemented. We only need to learn how to use these classes. We can learn how to use these APIs through the help documentation.
1.2 Specific use of API help documentation

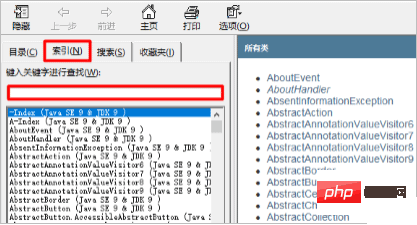
- ##Enter Random
# in the input box
##Look at which package the class is under
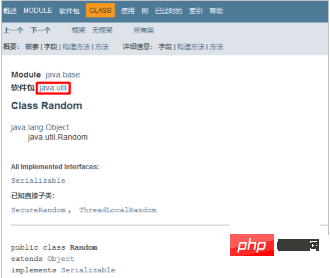
Look at the description of the class
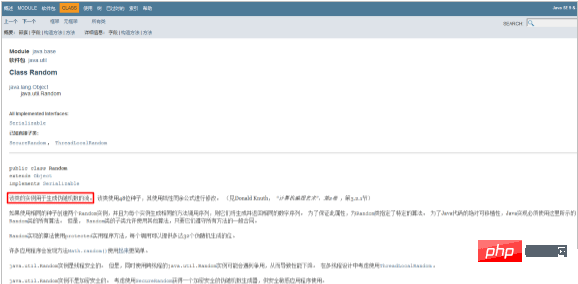
##See the construction method
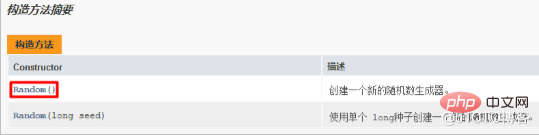
Look at member methods
2.String class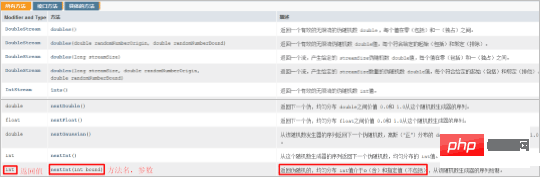
2.1String class overview
String The class represents a string, and all string literals in a Java program (such as "abc") are implemented as instances of this class. That is, all double-quoted strings in Java programs are objects of the String class. The String class is under the java.lang package, so there is no need to import the package when using it!
2.2 Characteristics of the String class
Strings are immutable and their values cannot be changed after creation
-
Although String values are immutable, but they can be shared
-
A string is effectively equivalent to a character array (char[]), but the underlying principle is a byte array (byte[] )
-
2.3Construction method of String class
Commonly used construction methods

Sample code
-
public class StringDemo01 {
public static void main(String[] args) {
//public String(): Create a blank string object without any content
String s1 = new String();
System.out.println("s1:" s1);
// public String(char[] chs): Create a string object based on the contents of the character array
char[] chs = {'a', 'b', 'c'};
String s2 = new String (chs);
System.out.println("s2:" s2);
//public String(byte[] bys): Create a string object based on the contents of the byte array
byte[] bys = {97, 98, 99};
String s3 = new String(bys);
System.out.println("s3:" s3);
/ /String s = "abc"; Create a string object by direct assignment, the content is abc
String s4 = "abc";
System.out.println("s4:" s4);
}
}
The specific execution results are as follows:
2.4 The difference between the two ways of creating string objects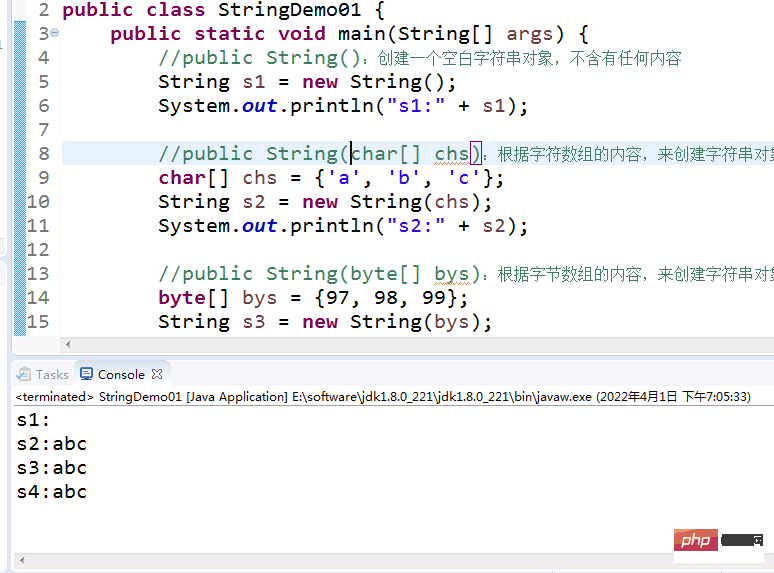
Create through the construction method
-
String objects created through new, each time new will apply for a memory space, although the content is the same, the address value is different
Create by direct assignment
-
A string given in "" mode, as long as the character sequence is the same (order and case), no matter how many times it appears in the program code , the JVM will only create a String object and maintain it in the string pool
2.5 Comparison of strings
2.5.1 The role of ==
Compare basic data types: Compare specific values
-
Compare reference data types: Compare object address values
-
2.5.2 The role of the equals method
Method introduction
-
public boolean equals(String s) Compares whether the contents of two strings are the same and distinguishes the size
Sample code
public class StringDemo02 {
public static void main(String[] args) {
//Constructor method to get the object
char[] chs = {'a' , 'b', 'c'};
String s1 = new String(chs);
String s2 = new String(chs);
//Get the object by direct assignment
String s3 = "abc";
String s4 = "abc";
//Compare whether the string object addresses are the same
System.out.println(s1 == s2);
System.out.println(s1 == s3);
System.out.println(s3 == s4);
System.out.println("--------");
//Compare whether the string contents are the same
System.out.println(s1.equals(s2));
System.out.println(s1.equals(s3));
System.out.println(s3.equals(s4));
}
}
The specific execution results are as follows:
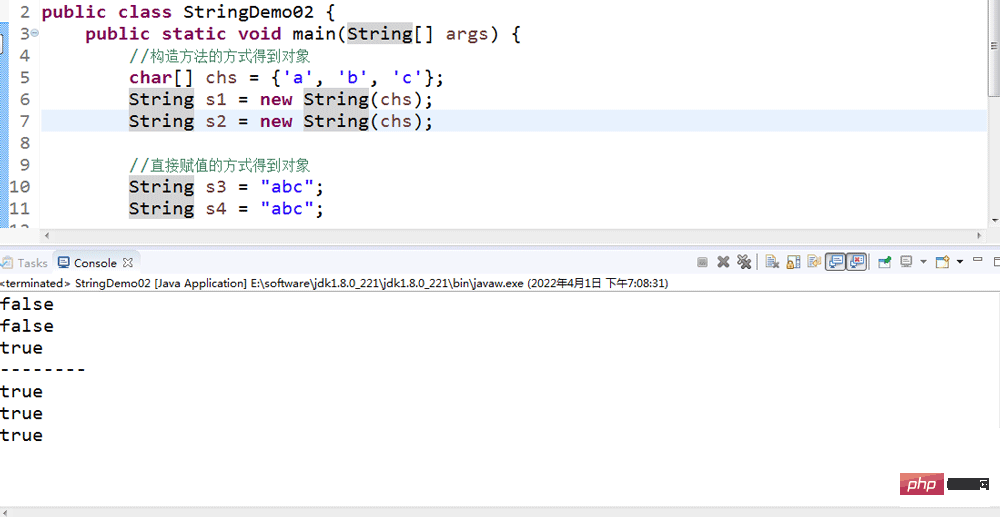
##2.6 User Login Case
2.6.1 Case Requirements
If the user name and password are known, please use a program to simulate user login. A total of three opportunities will be given. After logging in, corresponding prompts will be given
2.6.2 Code implementation
<br>
/*
Ideas:
1: Known username and password, definition Two strings can be represented
2: Enter the user name and password to log in with the keyboard, and use Scanner to implement
3: Compare the user name and password entered with the keyboard with the known user name and password, and give Corresponding tips. To compare the contents of strings, use the equals() method to implement
4: Use a loop to achieve multiple opportunities. The number of times here is clear. Use a for loop to implement it, and when the login is successful, use break to end the loop
*/
public class StringTest01 {
public static void main(String[] args) {
//If the username and password are known, just define two string representations
String username = "itheima";
String password = "czbk";
//Use a loop to achieve multiple opportunities. The number of times here is clear. Use a for loop to implement it. When the login is successful, use break to end the loop
for (int i=0; i
//Enter the username and password to log in with the keyboard, use Scanner to implement
Scanner sc = new Scanner(System.in);
System.out.println("Please enter the user name:");
String name = sc.nextLine();
System.out.println("Please enter the password:");
String pwd = sc.nextLine();
//Compare the user name and password entered by the keyboard with the known user name and password, and give corresponding prompts. String content comparison is implemented using the equals() method
if (name.equals(username) && pwd.equals(password)) {
System.out.println("Login successful");
break;
} else {
if(2-i == 0) {
System.out.println("Your account is locked, please contact the administrator");
} else {
//2,1,0
//i,0,1,2
System.out.println("Login failed, you still have" (2 - i) "second chances") ;
}
}
}
}
}
The specific execution results are as follows:
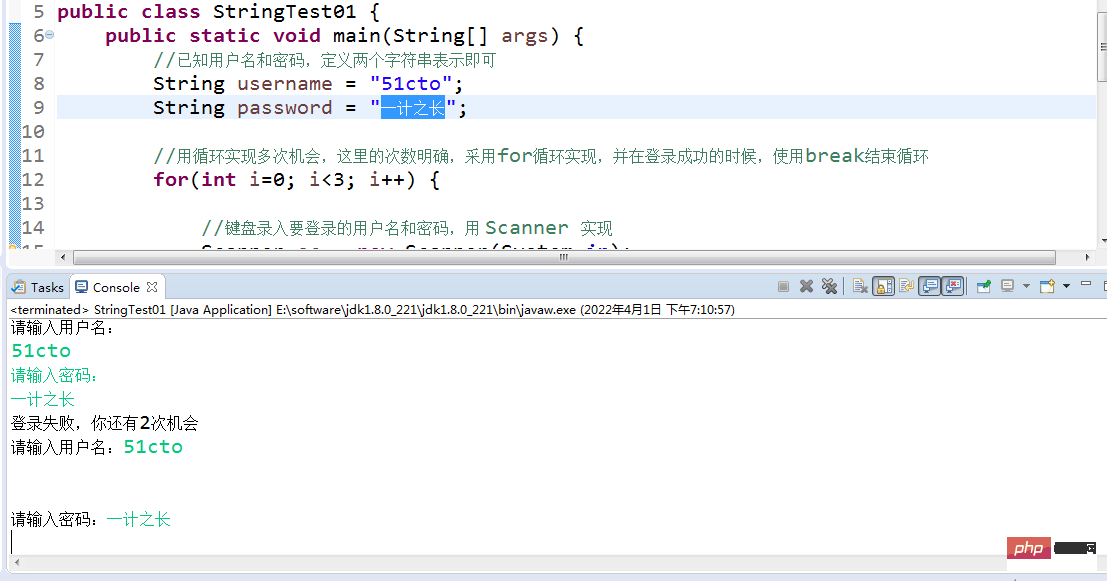
2.8 Help Document View String common methods
Method name
|
Description
|
public boolean equals(Object anObject)
|
Compare the contents of strings, strictly case-sensitive (username and password)
|
public char charAt(int index)
|
Returns the char value at the specified index
|
public int length()
|
Return the length of this string
|
##3.StringBuilder class
3.1 Overview of StringBuilder class
StringBuilder is a variable string class. We can think of it as a container. The variable here means that the content in the StringBuilder object is variable
3.2 The difference between the StringBuilder class and the String class
String class: the content is immutable StringBuilder class: the content is mutable Change 3.3Construction method of StringBuilder class
Commonly used construction methods
Method name | Description |

public class StringBuilderDemo01 {
public static void main(String[] args) {
//public StringBuilder(): Create a blank variable string object without any content
StringBuilder sb = new StringBuilder();
System.out.println("sb:" sb);
System.out.println("sb.length():" sb.length());
//public StringBuilder(String str): Create variable characters based on the content of the string String object
StringBuilder sb2 = new StringBuilder("hello");
System.out.println("sb2:" sb2);
System.out.println("sb2.length():" sb2 .length());
}
}
The specific execution results are as follows:
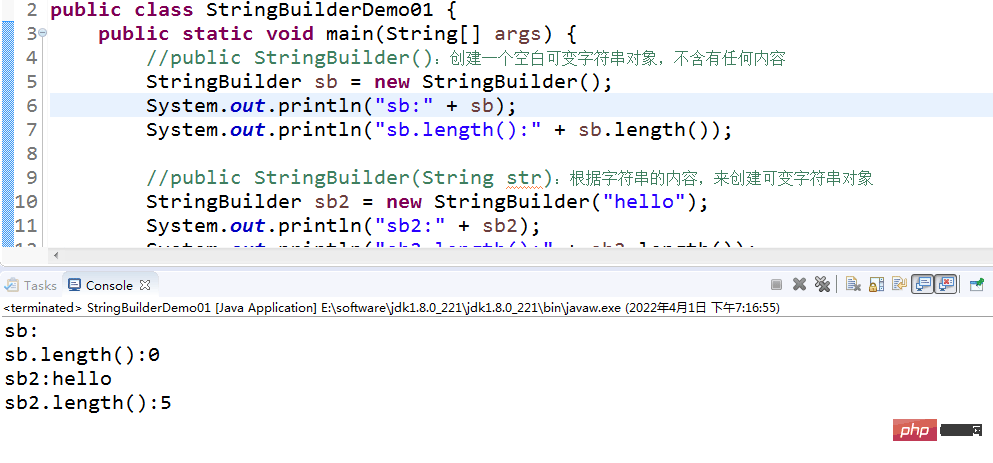
3.4 StringBuilder class addition and reverse method

public class StringBuilderDemo01 {
public static void main(String[] args) {
//Create object
StringBuilder sb = new StringBuilder();
//Chain programming
sb.append("hello").append("world").append("java").append(100);
System.out.println(" sb:" sb);
//public StringBuilder reverse(): Returns the reverse character sequence
sb.reverse();
System.out.println("sb:" sb);
}
}
The specific execution results are as follows:
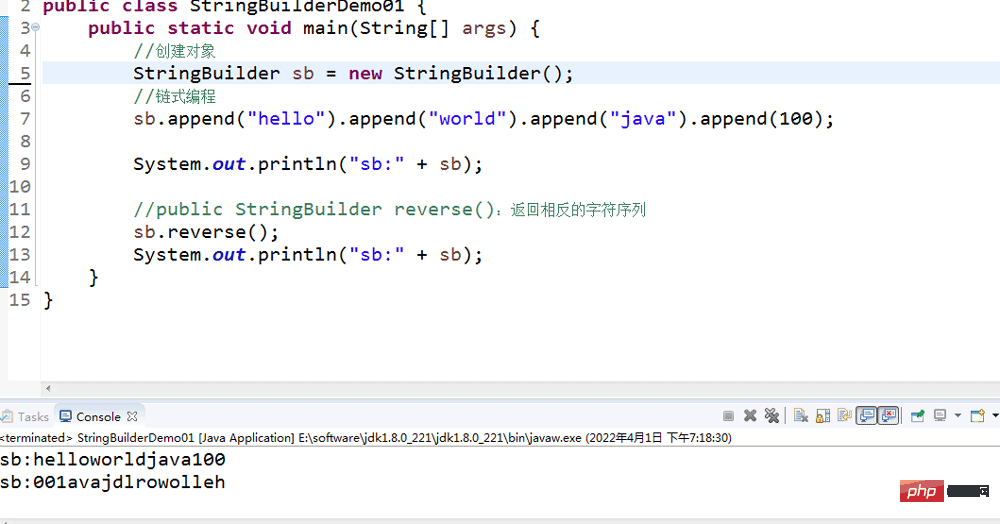
3.5 StringBuilder and String conversion
public String toString(): Convert StringBuilder to String
public StringBuilder(String s): Converting String to StringBuilder can be achieved through the constructor
public class StringBuilderDemo02 {
public static void main(String[] args) {
String s = sb.toString();
System.out.println(s);
String s = "hello";
StringBuilder sb = new StringBuilder(s);
System.out.println(sb);
}
}
Specific execution The results are as follows:
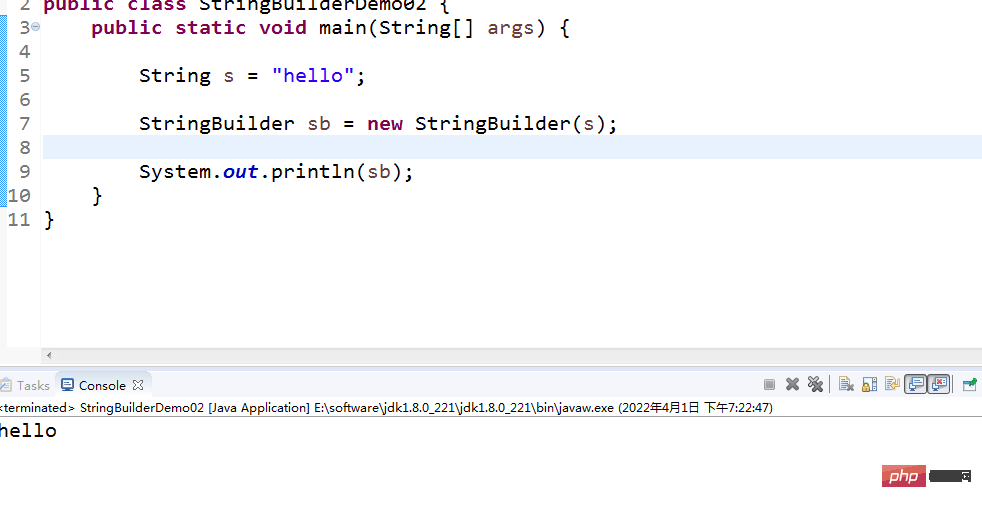
3.6 String splicing upgraded version case
3.6.1 Case requirements
Define a method to put int array The data is spliced into a string according to the specified format and returned, the method is called, and the result is output on the console. For example, the array is int[] arr = {1,2,3};, and the output result after executing the method is: [1, 2, 3]
3.6.2 Code Implementation
<br>
/*
Idea:
1: Define an array of type int, and use static initialization to complete the initialization of the array elements
2: Define a method for splicing the data in the int array into a format according to the specified format A string is returned.
Return value type String, parameter list int[] arr
3: Use StringBuilder in the method to splice as required, and convert the result into String return
4: Call the method and use a variable to receive the result
5: Output results
*/
public class StringBuilderTest01 {
public static void main(String[] args) {
//Define an array of int type and use static initialization to complete the array elements Initialization
int[] arr = {1, 2, 3};
//Call the method and use a variable to receive the result
String s = arrayToString(arr);
//Output results
System.out.println("s:" s);
}
//Define a method to convert the data in the int array as specified The format is spliced into a string and returns
/*
two clear: Return value type: String
Parameters: int[] arr
*/
public static String arrayToString(int[] arr ) {
//Use StringBuilder in the method to splice as required, and convert the result into String and return
StringBuilder sb = new StringBuilder();
sb.append("[");
for(int i=0; iif(i == arr.length-1) {
sb.append(arr[i]);
} else {
sb.append(arr[i]).append(", ");
}
}
sb.append("]");
String s = sb.toString();
return s;
}
}
The specific execution results are as follows:
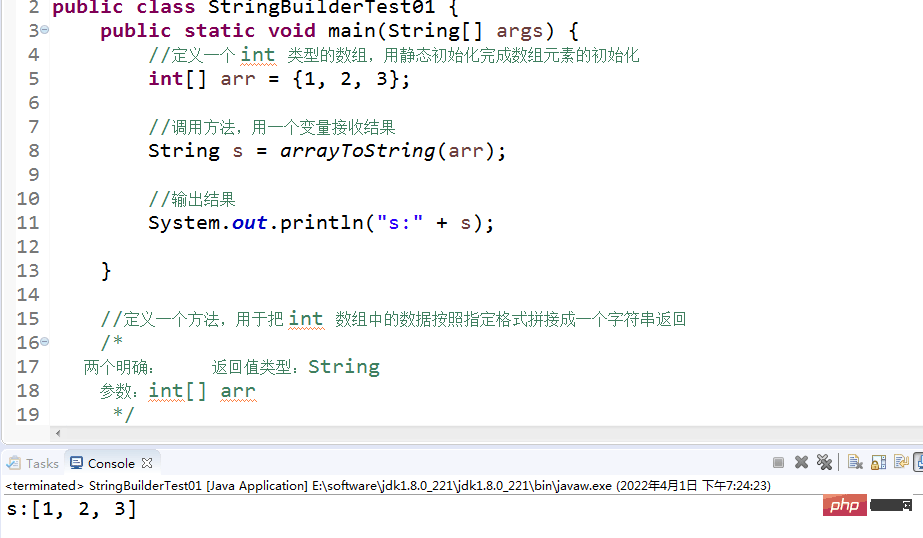
3.7 View the help documentation for common methods of StringBuilder
##Method name
|
Description
|
public StringBuilder append (any type) |
Add data and return the object itself |
public StringBuilder reverse() |
Returns the reverse character sequence |
public int length() |
Returns the length, the actual stored value |
public String toString() |
You can convert StringBuilder into String through toString() |
The above is the detailed content of How to use Java API?. For more information, please follow other related articles on the PHP Chinese website!