Java is a widely used programming language that can be used to perform various computing and data processing tasks by writing code. In Java we can convert Word document to HTML using different API implementations. In this article, we will focus on converting Word documents to HTML using Apache POI (Java API for reading and writing Microsoft Office files).
Introduction
When working with Word documents, converting them to HTML is a common need. This can make it easier to display and share documents on the web. There are many libraries in Java that help us achieve this task. One way is to use the Apache POI API.
Apache POI is an open source Java API that can be used to read and write Microsoft Office files. We can convert Word documents to HTML using its XWPF (Word Document Processor) class library.
Implementation
First, we need to add the following dependencies to the project:
<dependency> <groupid>org.apache.poi</groupid> <artifactid>poi-ooxml</artifactid> <version>4.1.2</version> </dependency> <dependency> <groupid>org.apache.poi</groupid> <artifactid>poi-ooxml-schemas</artifactid> <version>4.1.2</version> </dependency> <dependency> <groupid>org.apache.xmlbeans</groupid> <artifactid>xmlbeans</artifactid> <version>3.1.0</version> </dependency>
Then, we will create a class named WordToHtmlConverter
, which The class will have a convertToHtml
method whose parameter is the path to the Word document. This method will use the POI API implementation to convert the Word document to HTML.
import java.io.*; import org.apache.poi.xwpf.converter.core.*; import org.apache.poi.xwpf.converter.xhtml.*; import org.apache.poi.xwpf.usermodel.*; public class WordToHtmlConverter { public void convertToHtml(String wordFilePath) { try { InputStream inputStream = new FileInputStream(new File(wordFilePath)); IXWPFConverter<htmlsettings> converter = XWPFConverter.getInstance(); HTMLSettings htmlSettings = new HTMLSettings(); OutputStream outputStream = new FileOutputStream(new File("output.html")); converter.convert(new XWPFDocument(inputStream), outputStream, htmlSettings); } catch (Exception ex) { ex.printStackTrace(); } } }</htmlsettings>
In this example, we first open the input stream of the Word document and then instantiate the IXWPPFonverter object. We also created the HTMLSettings class to serve as the configuration file for the transformation. Finally, we save the results to a file called "output.html".
When using this method, you simply pass the string of the full path to the Word document to the convertToHtml
method, as shown below:
WordToHtmlConverter converter = new WordToHtmlConverter(); converter.convertToHtml("/path/to/my/document.docx");
Conclusion
In this article, we have demonstrated how to convert a Word document to HTML using Apache POI. Java provides several ways to convert Word documents, but using Apache POI is a very convenient and practical method. Consider using this method if you need to display and share your Word document on the web.
The above is the detailed content of How to convert Word document to HTML in java. For more information, please follow other related articles on the PHP Chinese website!

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.

React is a JavaScript library for building user interfaces. Its core idea is to build UI through componentization. 1. Components are the basic unit of React, encapsulating UI logic and styles. 2. Virtual DOM and state management are the key to component work, and state is updated through setState. 3. The life cycle includes three stages: mount, update and uninstall. The performance can be optimized using reasonably. 4. Use useState and ContextAPI to manage state, improve component reusability and global state management. 5. Common errors include improper status updates and performance issues, which can be debugged through ReactDevTools. 6. Performance optimization suggestions include using memo, avoiding unnecessary re-rendering, and using us

Using HTML to render components and data in React can be achieved through the following steps: Using JSX syntax: React uses JSX syntax to embed HTML structures into JavaScript code, and operates the DOM after compilation. Components are combined with HTML: React components pass data through props and dynamically generate HTML content, such as. Data flow management: React's data flow is one-way, passed from the parent component to the child component, ensuring that the data flow is controllable, such as App components passing name to Greeting. Basic usage example: Use map function to render a list, you need to add a key attribute, such as rendering a fruit list. Advanced usage example: Use the useState hook to manage state and implement dynamics

React is the preferred tool for building single-page applications (SPAs) because it provides efficient and flexible ways to build user interfaces. 1) Component development: Split complex UI into independent and reusable parts to improve maintainability and reusability. 2) Virtual DOM: Optimize rendering performance by comparing the differences between virtual DOM and actual DOM. 3) State management: manage data flow through state and attributes to ensure data consistency and predictability.

React is a JavaScript library developed by Meta for building user interfaces, with its core being component development and virtual DOM technology. 1. Component and state management: React manages state through components (functions or classes) and Hooks (such as useState), improving code reusability and maintenance. 2. Virtual DOM and performance optimization: Through virtual DOM, React efficiently updates the real DOM to improve performance. 3. Life cycle and Hooks: Hooks (such as useEffect) allow function components to manage life cycles and perform side-effect operations. 4. Usage example: From basic HelloWorld components to advanced global state management (useContext and

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.

React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through ReactDevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintaining dependability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
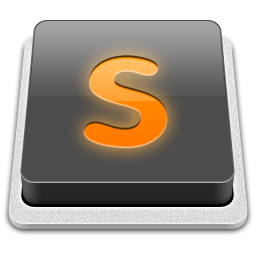
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.