PHP is an object-oriented server-side scripting language, which is very suitable for developing server-side Web applications. Arrays are a very important data type in PHP that allow multiple values to be grouped together and referenced and manipulated as a single variable. In the process of using arrays, we often encounter situations where we need to query elements in the same array. This article will introduce how to use PHP to query elements in the same array.
1. Use loop traversal to query
PHP provides a variety of loop statements that can be used to traverse the elements in the array and perform query operations. Here we introduce two commonly used loop statements:
1.1 for loop
The for loop is a commonly used loop statement that can be used to traverse elements in an array. The following is a sample code that uses a for loop to query elements in the same array:
<?php $array = array(1, 2, 3, 4, 1, 3); $len = count($array); for ($i = 0; $i < $len; $i++) { for ($j = $i + 1; $j < $len; $j++) { if ($array[$i] == $array[$j]) { echo "相同的元素为:".$array[$i]."<br />"; } } } ?>
In the above code, we first define an array containing repeated elements $array
, and then use a for loop Iterate through all the elements in the array and for each element we again use a for loop to find the element that is the same as it. If the same element is found, the element is output.
1.2 foreach loop
The foreach loop is also a commonly used loop statement, which can be used to traverse the elements in the array and perform query operations. The following is a sample code that uses a foreach loop to query elements in the same array:
<?php $array = array(1, 2, 3, 4, 1, 3); $count = array_count_values($array); foreach ($count as $key => $value) { if ($value > 1) { echo "相同的元素为:".$key."<br>"; } } ?>
In the above code, we first define an array containing repeated elements $array
, and then use the PHP built-in Function array_count_values
counts the number of occurrences of each element in the array, and stores the statistical results in the associative array $count
. Finally, we use a foreach loop to traverse the $count
array and determine whether the number of occurrences is greater than 1. If it is greater than 1, the element will be output.
2. Use PHP built-in function query
In PHP, there are multiple built-in functions that can be used to query elements in the same array. Below we introduce two commonly used functions:
2.1 array_count_values
array_count_values function can be used to count the number of occurrences of each element in an array and store the statistical results in an associative array. The following is a sample code that uses the array_count_values function to query elements in the same array:
<?php $array = array(1, 2, 3, 4, 1, 3); $count = array_count_values($array); foreach ($count as $key => $value) { if ($value > 1) { echo "相同的元素为:".$key."<br>"; } } ?>
In the above code, we first define an array containing repeated elements $array
, and then use the PHP built-in Function array_count_values
counts the number of occurrences of each element in the array, and stores the statistical results in the associative array $count
. Finally, we use a foreach loop to traverse the $count
array and determine whether the number of occurrences is greater than 1. If it is greater than 1, the element will be output.
2.2 array_unique, array_diff_assoc and array_diff_key
The array_unique function can be used to remove duplicate elements from an array and return the array after removing duplicate elements. The array_diff_assoc and array_diff_key functions can be used to compare the difference between two arrays and return different elements. By using these functions, we can also query elements in the same array. The following is a sample code:
<?php $array = array(1, 2, 3, 4, 1, 3); $unique = array_unique($array); $diff = array_diff_assoc($array, $unique); foreach ($diff as $key => $value) { echo "相同的元素为:".$value."<br>"; } ?>
In the above code, we first define an array containing repeated elements$array
, then use the array_unique function to remove duplicate elements in the array, and store the result in the $unique
variable. Then we use the array_diff_assoc function to compare the difference between the two arrays and store the different elements into the $diff
variable. Finally we use foreach to loop through the $diff
array and output the same elements.
Summary
The above is how to query elements in the same array in PHP. We can easily implement this function by using loop statements, built-in functions and other methods. In actual development, you can choose a method that suits you for query operations. At the same time, it should be noted that repeated elements may have an impact on the performance of the program, so in actual development, you should try to avoid repeated elements in the array.
The above is the detailed content of How to query elements in the same array using PHP. For more information, please follow other related articles on the PHP Chinese website!
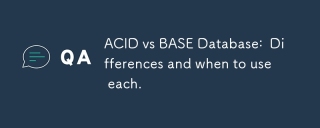
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
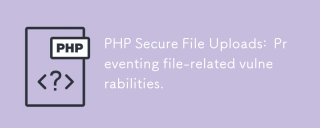
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
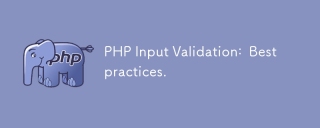
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
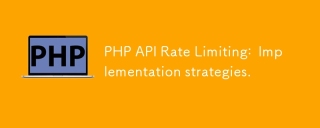
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
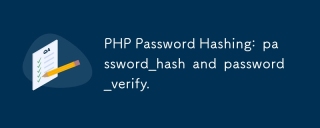
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
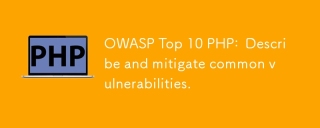
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
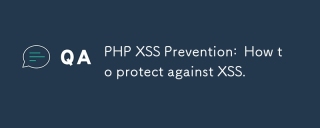
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
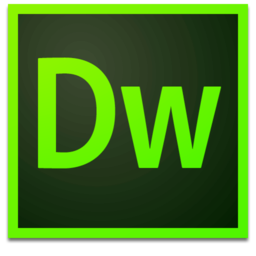
Dreamweaver Mac version
Visual web development tools
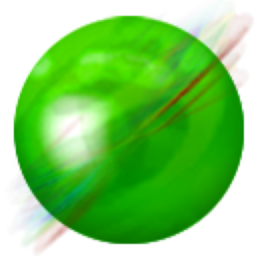
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software