In PHP development, there is often a need to query duplicate key values of array elements. For example, when processing form data submitted by users, you may need to ensure that all submitted data is unique. This article will introduce how to use PHP language to query duplicate key values in an array.
The array in PHP is a very commonly used and very powerful data structure that can store the correspondence between keys and values. The keys of the array can be integers or strings, and the values can be any type of data. In PHP, you can create an array using the following syntax:
$myArray = array('apple', 'banana', 'cherry');
The above code creates an array with three elements, each element being a string. In the array, each element has a corresponding numeric index, and the array elements can be accessed through the index:
echo $myArray[0]; // 输出 'apple' echo $myArray[1]; // 输出 'banana' echo $myArray[2]; // 输出 'cherry'
In addition to numeric indexes, PHP arrays can also use string indexes. For example:
$myArray = array('name' => '张三', 'age' => 18, 'gender' => '男'); echo $myArray['name']; // 输出 '张三' echo $myArray['age']; // 输出 18 echo $myArray['gender']; // 输出 '男'
During the development process, we often encounter the need to query duplicate key values in an array to ensure data uniqueness and avoid errors caused by data duplication. For example, we might need to query a list of users to see if they have the same username. In PHP, you can use the array_count_values() function to query the number of occurrences of array elements and count the number of repeated elements. For example:
$users = array('张三', '李四', '王五', '张三', '李四'); $result = array_count_values($users); print_r($result);
The output result is as follows:
Array ( [张三] => 2 [李四] => 2 [王五] => 1 )
In the above code, we created an array $users containing five elements, which contains two repeated elements. By calling the array_count_values() function, you can use each element in the array as a key and the number of occurrences as a value to form a new array. In the output of this new array, the key is the element in the original data array, and the value is the number of times this element appears in the original array.
If you want to query all repeated elements in the array, you can use the array_keys() function to get the key with a value of 2 (occurrence greater than 1) in the result array, as shown below:
$users = array('张三', '李四', '王五', '张三', '李四'); $result = array_count_values($users); $repeated = array_keys($result, 2); print_r($repeated);
The output is as follows:
Array ( [0] => 张三 [1] => 李四 )
In the above code, we use the array_keys() function to get the key with value 2, which is the key of all repeated elements in the array. Each element in the output of this new array is a duplicate element.
In actual development, we may need to be more flexible in handling duplicate elements, such as removing them from the array, or marking them as error data. The following is an example of using a foreach loop to traverse an array and mark duplicate elements:
$users = array('张三', '李四', '王五', '张三', '李四'); $counts = array(); foreach ($users as $user) { if (isset($counts[$user])) { $counts[$user]++; } else { $counts[$user] = 1; } } foreach ($users as &$user) { if ($counts[$user] > 1) { $user = $user . '(重复)'; } } print_r($users);
The output results are as follows:
Array ( [0] => 张三(重复) [1] => 李四(重复) [2] => 王五 [3] => 张三(重复) [4] => 李四(重复) )
In the above code, we first define an empty array $counts for Record the number of occurrences of each element in the original array. Then iterate over the original array $users. If an element already exists in the $counts array, add 1 to its occurrence count; otherwise, set its occurrence count to 1. Finally, the original array $users is traversed again, and if an element appears more than 1 in the $counts array, it is marked as a duplicate element.
In addition to the above methods, there are many other array processing functions in PHP that you can refer to. For example, the array_unique() function can be used to remove duplicate elements from an array, and the array_diff() function can be used to find non-duplicate elements, etc.
In short, querying duplicate key values in an array is a common requirement in PHP development. By using the array_count_values() function, we can quickly get the number of occurrences of each element in the array to handle duplicate elements.
The above is the detailed content of How to query duplicate key values in an array in php. For more information, please follow other related articles on the PHP Chinese website!
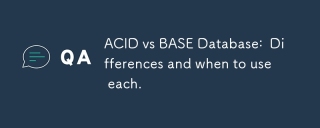
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
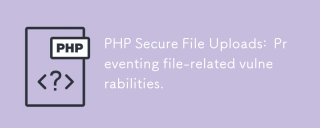
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
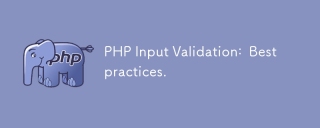
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
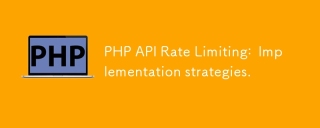
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
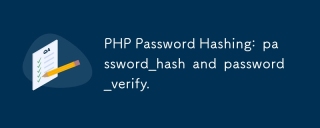
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
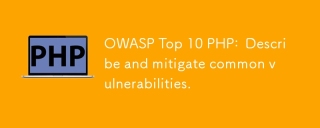
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
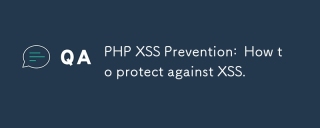
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
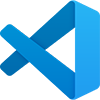
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
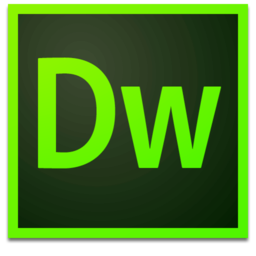
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor