Arrays in PHP are a very powerful data type. They allow us to store and process various types of data, including strings, integers, floating point numbers, Boolean values, etc. In PHP, arrays can be regular arrays or arrays like objects. This kind of array is like an object, each element has its own properties and methods, that is, accessors and mutators. In this article, we will learn how to use PHP array objects for data processing.
- What is an array object?
First, let us understand the concept of array objects. Array objects refer to arrays that can store multiple data in PHP, and each data can be an object. An array object is similar to an ordinary array, but each element can have its own properties and methods. In PHP, array objects are often used as a collection of related objects.
- Creating an array object
Creating an array object is very simple, just use the array() function. For example:
$students_array = array( array('name' => '小明', 'age' => 18, 'score' => array(80,90,95)), array('name' => '小红', 'age' => 19, 'score' => array(85,86,90)), array('name' => '小王', 'age' => 20, 'score' => array(75,70,80)) );
The above code creates an array object containing three student information. Each student information contains name, age and score, and the score attribute is an array containing three elements.
- Accessing array objects
The way to access array objects is similar to accessing ordinary arrays. You can use array subscripts to read and write elements. For example:
echo $students_array[0]['name']; //输出 小明 echo $students_array[1]['score'][1]; //输出 86
Here you access the properties of the array object elements by specifying the array subscript. In the first example, the name of the first student is accessed, and in the second example, the second subject score of the second student is accessed.
- Modify the array object
Contrary to access, to modify the elements of the array object, we need to assign the new value to the original variable. For example:
$students_array[2]['score'][0] = 80;
Here, the third student’s first subject score is modified to 80 points.
- Methods in array objects
Methods in array objects are similar to methods in objects, and we can use arrow symbols to call them. For example:
class student{ private $name;//私有 private $age;//私有 private $score;//私有 function __construct($name, $age, $score){ $this->name = $name; $this->age = $age; $this->score = $score; } //可访问器方法 function getName(){ return $this->name; } //可变器方法 function setName($name){ $this->name = $name; } } $student1 = new student("小明",18,array(80,90,95));//实例化student对象 echo $student1->getName();//使用可访问器方法获取姓名 小明 $student1->setName("小红");//使用可变器方法修改姓名 echo $student1->getName();//输出 小红
The above code defines a student class student, which contains three private properties: name, age and score, as well as an accessor method and a mutator method. Then an instantiated object student1 was created, the accessor method was used to obtain the name, the variable method was used to modify the name, and the name was obtained again.
- Traverse array objects
Loops can be used to traverse array objects, including loop structures such as for, foreach, and while. For example:
//for循环方式 for($i=0;$i<count></count>'; } //foreach循环方式 foreach($students_array as $student){ echo $student['name'].': '; foreach($student['score'] as $value){ echo $value.' '; } echo '<br>'; }
The above code uses for and foreach loops to traverse the array object and output the name and score of each student.
- Conclusion
In PHP, the array object is a very powerful data type that can be used to handle various types of data. Array objects can store the properties and methods of corresponding objects, and maintain and modify data through accessors and mutators. In actual development, we can make full use of array objects to simplify data processing logic and improve code readability and maintainability.
The above is the detailed content of How to deal with php array objects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
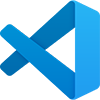
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
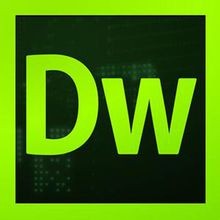
Dreamweaver CS6
Visual web development tools
