Golang is an efficient and reliable programming language. Its built-in concurrency support and excellent performance have attracted many developers to join it. TCP protocol is one of the most important communication protocols in computer networks, and its implementation requires a lot of skills and experience. This article will introduce how to use Golang to implement TCP clients and servers, including how to establish connections, send and receive data, and error handling.
1. Basic knowledge of TCP
TCP protocol (Transmission Control Protocol) is a reliable, connection-oriented, byte stream-based transmission protocol. It is characterized by orderly data transmission, no loss, no duplication, no errors, and supports full-duplex communication. Both parties to the data transmission can send and receive data at any time. When developing applications, we usually use the TCP protocol to transmit data, such as connecting to databases, sending emails, browsing websites, etc.
In the TCP protocol, the two ends of the communication are called "client" and "server" respectively. The client actively initiates a connection request to the server, and the server responds to the connection after receiving the request. There is a connection between the two ends. A TCP connection is established. After the connection is established, the client can send data to the server through the connection, and the server can also send data to the client through the connection. After completing the data transfer, the connection needs to be closed explicitly.
2. TCP client implementation
It is very simple to implement TCP client in Golang. The specific steps are as follows:
- Create TCP connection
Create a TCP connection using the "Dial" function in Golang's "net" package.
conn, err := net.Dial("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("Failed to connect to server:", err) return } defer conn.Close()
The first parameter of the "Dial" function specifies the protocol type to be connected (tcp or udp), and the second parameter specifies the IP address and port number of the server. If the connection is successful, a connection object of type "net.Conn" will be returned, otherwise an error will be returned.
- Send data
After creating the connection, we can send data to the server. Data can be sent using the "Write" method of the "Conn" object. The parameter of this method is a byte array.
data := []byte("Hello, server!") _, err := conn.Write(data) if err != nil { fmt.Println("Failed to send data to server:", err) return }
The return value of the "Write" method is the number of bytes written, which we can use to determine whether the data is sent successfully.
- Receive data
After sending data to the server, we need to wait for the server's response. Data can be received using the "Read" method of the "Conn" object. The parameter of this method is a byte array, and the return value is the number of bytes received.
buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { fmt.Println("Failed to receive data from server:", err) return } fmt.Println("Received from server:", string(buffer[:n]))
The received data is stored in a byte array and we can convert it to a string through the "string" function.
- Close the connection
When we complete the data transfer, we need to explicitly close the connection to release resources.
conn.Close()
It should be noted that the "defer" statement should be used to close the connection at the end of the function to ensure that the connection is automatically closed after the function is executed.
3. TCP server implementation
Implementing a TCP server in Golang is also very simple. The specific steps are as follows:
- Create a listener
Use the "Listen" function in the "net" package to create a TCP server listener.
listener, err := net.Listen("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("Failed to start server:", err) return } defer listener.Close()
The first parameter of the "Listen" function specifies the protocol type to be listened to (tcp or udp), and the second parameter specifies the IP address and port number of the server. If the startup is successful, a listening object of type "net.Listener" will be returned, otherwise an error will be returned.
- Establishing a connection
When a client requests a connection, you can use the "Accept" method of the "Listener" object to establish a connection.
conn, err := listener.Accept() if err != nil { fmt.Println("Failed to accept connection:", err) break } defer conn.Close()
The "Accept" method will block program execution until a connection request is received. When the connection is successfully established, a "net.Conn" type connection object will be returned, otherwise an error will be returned.
- Receive data
When the connection is successfully established, the server can receive the data sent by the client. Data can be received using the "Read" method of the "Conn" object.
buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { fmt.Println("Failed to receive data from client:", err) break } fmt.Println("Received from client:", string(buffer[:n]))
The received data is stored in a byte array and we can convert it to a string through the "string" function.
- Send data
After the server receives the data, it can use the "Write" method of the "Conn" object to send data to the client.
data := []byte("Hello, client!") _, err = conn.Write(data) if err != nil { fmt.Println("Failed to send data to client:", err) break }
The return value of the "Write" method is the number of bytes written, which we can use to determine whether the data is sent successfully.
- Close the connection
When the data exchange with the client is completed, the connection needs to be explicitly closed to release resources.
conn.Close()
It should be noted that if we use a "for" loop, then the "defer" statement should be placed outside the "for" loop to ensure that resources are released uniformly after all connections are closed.
4. Error handling
Error handling is particularly important when using the TCP protocol for data communication. In Golang, the "error" type can be used to represent possible error conditions in function return values.
In the implementation of TCP client and server, we need to perform error handling for operations such as connection, data sending and receiving. Normally, we can use the "if err != nil" statement to determine whether the function returns an error, and handle it accordingly when an error occurs.
For example, in the TCP client implementation, we can use the following code to determine whether the connection is successfully established:
conn, err := net.Dial("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("Failed to connect to server:", err) return } defer conn.Close()
如果连接建立失败,将打印错误信息并退出程序。
在TCP服务器实现中,如果在接收数据时发生了错误,我们应该关闭连接并退出循环。
n, err := conn.Read(buffer) if err != nil { fmt.Println("Failed to receive data from client:", err) break }
以上只是TCP客户端和服务器的基本实现方法,开发者可以根据实际需求进行更加复杂的实现,比如多连接、并发、SSL加密等。Golang的高并发和轻量级特性使得它适合于TCP服务器等高并发场景的开发。
The above is the detailed content of How to implement tcp in golang. For more information, please follow other related articles on the PHP Chinese website!
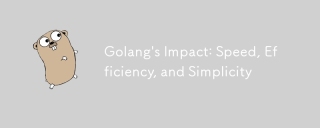
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
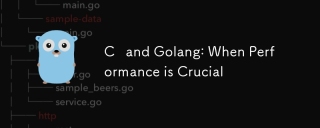
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
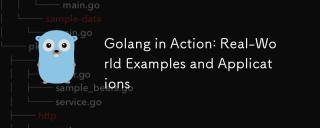
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
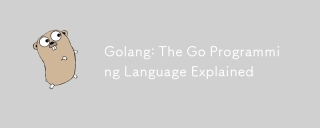
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
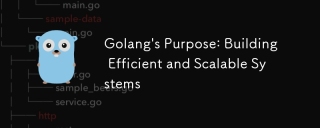
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
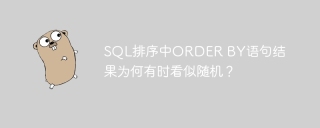
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
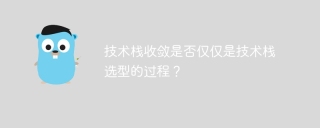
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
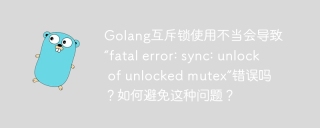
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
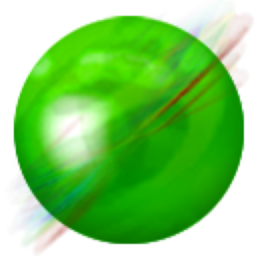
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
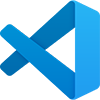
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
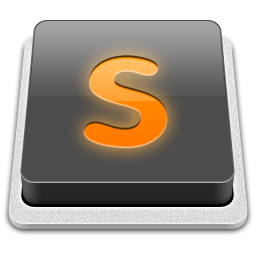
SublimeText3 Mac version
God-level code editing software (SublimeText3)
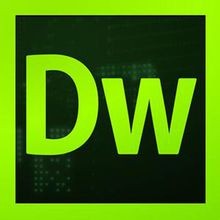
Dreamweaver CS6
Visual web development tools