In PHP, object arrays and index arrays are two different data types. An object array is an array of objects, while an index array is an array of values, and each value has an associated index.
In some cases, we may need to convert the object array to an index array to make it easier to process and manipulate the data. Fortunately, PHP provides some built-in functions and tricks to easily convert an array of objects into an indexed array. Below we will describe in detail how to implement this conversion process.
Method 1: Use the array_column function
The array_column function in PHP can be used to extract the value of a key from an array and return an array of these values. When converting an array of objects into an indexed array, we can use this function to extract the object's property values and form them into a new array. The following is a sample code snippet:
// 定义一个对象数组 $users = [ new User('john', 'doe', 28), new User('jane', 'smith', 32), new User('bob', 'jones', 45), ]; // 使用 array_column 函数提取对象的属性值 $ages = array_column($users, 'age'); // 打印结果 print_r($ages);
In this example, we first define an object array containing three user objects. We then use the array_column function to extract the age property of each object and form them into a new array. Finally, we print this new array to verify that the conversion was successful.
Method 2: Use a foreach loop
Another way to convert an object array into an index array is to use a foreach loop. This approach is a bit more low-level, but it allows for more flexibility in handling object properties. The following is a sample code snippet:
// 定义一个对象数组 $users = [ new User('john', 'doe', 28), new User('jane', 'smith', 32), new User('bob', 'jones', 45), ]; // 创建一个空的索引数组 $ages = []; // 遍历对象数组并将属性值存入新数组 foreach ($users as $user) { $ages[] = $user->age; } // 打印结果 print_r($ages);
In this example, we first define an object array containing three user objects. We then create an empty indexed array to store the attribute values we extracted. Next, we use a foreach loop to loop through the object array and store the age attribute value of each object into a new array. Finally, we print this new array to verify that the conversion was successful.
Method 3: Use the array_map function
The array_map function in PHP can be used to apply each element of an array to a callback function and return a new array. When converting an array of objects to an indexed array, we can use this function to map each object to a property value and form them into a new array. The following is a sample code snippet:
// 定义一个对象数组 $users = [ new User('john', 'doe', 28), new User('jane', 'smith', 32), new User('bob', 'jones', 45), ]; // 定义一个回调函数,用于从对象中提取属性值 $extractAge = function ($user) { return $user->age; }; // 使用 array_map 函数将对象映射为属性值 $ages = array_map($extractAge, $users); // 打印结果 print_r($ages);
In this example, we first define an object array containing three user objects. We then define a callback function that extracts the age property value from each object. Next, we use the array_map function to map each object to an attribute value and form them into a new array. Finally, we print this new array to verify that the conversion was successful.
Conclusion
The above three methods can convert an object array into an index array, but different methods may be suitable for different scenarios and needs. For example, if you only need to extract an object property, using the array_column function is probably the easiest way to do it. If you need more flexibility with object properties and want to apply some logic during the conversion, then using a foreach loop or the array_map function may be a better choice. Whichever method you choose, you should consider data size and performance to avoid unnecessary overhead and latency.
The above is the detailed content of How to convert php object array into index array. For more information, please follow other related articles on the PHP Chinese website!
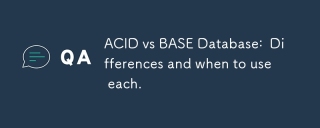
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
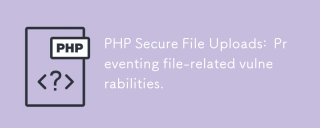
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
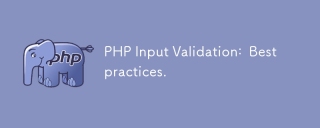
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
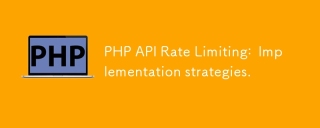
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
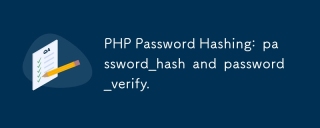
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
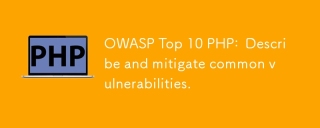
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
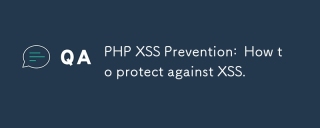
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
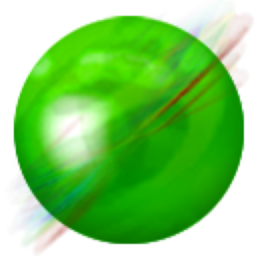
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use