PHP is a widely used programming language used to develop web applications and websites. One of the key aspects is processing data, especially JSON data. JSON (JavaScript Object Notation) is a formatted data exchange language commonly used for data exchange between web applications. In PHP, the process of converting JSON data into an array object is very common and very simple.
To convert JSON data into an array object in PHP, you can use PHP's built-in function: json_decode(). This function accepts a JSON formatted string as argument and converts it into a PHP array object.
The following is a simple example demonstrating how to convert JSON data into a PHP array object:
$json = '{"name":"Tom", "age":26, "city":"New York"}'; $array = json_decode($json, true); print_r($array);
In the above code, we define a JSON string and use json_decode() Function converts it to a PHP array object. The first parameter of the function is a JSON string, and the second parameter is a Boolean value. When set to true, an associative array will be returned instead of an object.
The output results are as follows:
Array ( [name] => Tom [age] => 26 [city] => New York )
In the above code, we can see that we successfully converted the JSON data into a PHP array object. This array contains all data information in the JSON data.
If you want to convert JSON data to a PHP object instead of an array, please set the second parameter of the json_decode() function to false or do not pass the second parameter. The following is an example:
$json = '{"name":"Tom", "age":26, "city":"New York"}'; $obj = json_decode($json); print_r($obj);
The output result is as follows:
stdClass Object ( [name] => Tom [age] => 26 [city] => New York )
In the above code, we use the json_decode() function to convert JSON data into a PHP object. This object contains all attribute information in the JSON data.
Summary
In PHP, it is very common to convert JSON data into array objects. This process can be easily accomplished using the built-in json_decode() function. By introducing JSON data, we can more conveniently use data exchange between web applications. What we need to remember is that when using the json_decode() function, the second parameter determines the type of return. If the second parameter is not passed, an object will be returned.
The above is the detailed content of How to convert json into array object in php. For more information, please follow other related articles on the PHP Chinese website!
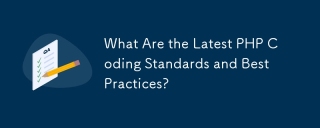
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
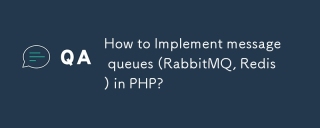
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
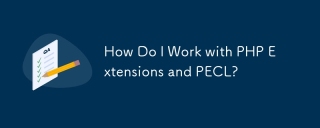
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
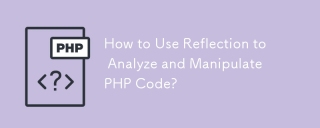
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
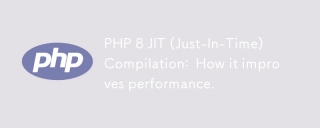
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
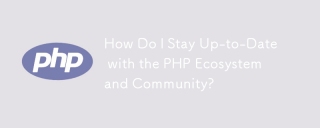
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
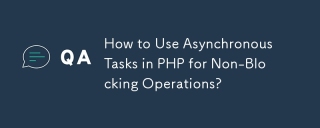
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
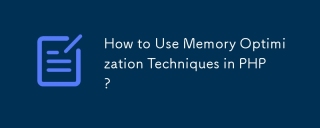
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
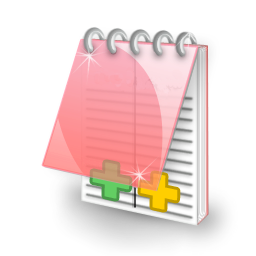
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
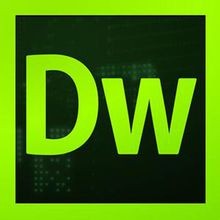
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
