PHP is a popular programming language that is widely used in website and application development. In PHP, array is a very useful data type that allows us to organize and store multiple values. Sometimes, we need to split a large array into multiple small arrays to process the data more conveniently. In this article, we will discuss how to split a large array into multiple small arrays in PHP.
Why do we need to divide the array into multiple arrays?
In many cases, the arrays we need to process may be very large and contain a large amount of data. If we process the entire array at once, we may run out of memory or take too long to run. At this time, we can split the large array into multiple small arrays to improve the efficiency of the program. For example, if we need to sort an array containing 1 million elements according to certain rules, then we can divide the large array into multiple small arrays, sort them separately, and finally merge them into an ordered array, so It can significantly improve the running efficiency of the program.
How to divide an array into multiple arrays?
Below we will introduce some common methods and techniques to split a large array into multiple small arrays.
- array_chunk() function
PHP provides a very convenient function array_chunk(), which can divide an array into multiple arrays according to the specified size. The following is a sample code for splitting an array using the array_chunk() function:
$big_array = range(1,100); // 生成一个包含100个元素的数组 $small_arrays = array_chunk($big_array, 10); // 将大数组分成10个元素一组的小数组 print_r($small_arrays); // 打印输出结果
The output results are as follows:
Array ( [0] => Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 [6] => 7 [7] => 8 [8] => 9 [9] => 10 ) [1] => Array ( [0] => 11 [1] => 12 [2] => 13 [3] => 14 [4] => 15 [5] => 16 [6] => 17 [7] => 18 [8] => 19 [9] => 20 ) [2] => Array ( [0] => 21 [1] => 22 [2] => 23 [3] => 24 [4] => 25 [5] => 26 [6] => 27 [7] => 28 [8] => 29 [9] => 30 ) [3] => Array ( [0] => 31 [1] => 32 [2] => 33 [3] => 34 [4] => 35 [5] => 36 [6] => 37 [7] => 38 [8] => 39 [9] => 40 ) [4] => Array ( [0] => 41 [1] => 42 [2] => 43 [3] => 44 [4] => 45 [5] => 46 [6] => 47 [7] => 48 [8] => 49 [9] => 50 ) [5] => Array ( [0] => 51 [1] => 52 [2] => 53 [3] => 54 [4] => 55 [5] => 56 [6] => 57 [7] => 58 [8] => 59 [9] => 60 ) [6] => Array ( [0] => 61 [1] => 62 [2] => 63 [3] => 64 [4] => 65 [5] => 66 [6] => 67 [7] => 68 [8] => 69 [9] => 70 ) [7] => Array ( [0] => 71 [1] => 72 [2] => 73 [3] => 74 [4] => 75 [5] => 76 [6] => 77 [7] => 78 [8] => 79 [9] => 80 ) [8] => Array ( [0] => 81 [1] => 82 [2] => 83 [3] => 84 [4] => 85 [5] => 86 [6] => 87 [7] => 88 [8] => 89 [9] => 90 ) [9] => Array ( [0] => 91 [1] => 92 [2] => 93 [3] => 94 [4] => 95 [5] => 96 [6] => 97 [7] => 98 [8] => 99 [9] => 100 ) )
From the above example, we can see that using the array_chunk() function can A large array is divided into smaller arrays, each smaller array containing a specified number of elements. This function is very suitable for batch processing of data and can greatly improve program efficiency.
- for loop
Another commonly used method is to use a for loop to achieve array splitting. This method is more flexible and can adjust the cycle conditions according to the specific situation. The following is a simple example:
$big_array = range(1,20); // 生成一个包含20个元素的数组 $chunk_size = 5; // 每个小数组包含5个元素 $small_arrays = []; // 用于存放分割后的小数组 $count = count($big_array); // 获取大数组的长度 for ($i = 0; $i <p>The output result is as follows: </p><pre class="brush:php;toolbar:false">Array ( [0] => Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 ) [1] => Array ( [0] => 6 [1] => 7 [2] => 8 [3] => 9 [4] => 10 ) [2] => Array ( [0] => 11 [1] => 12 [2] => 13 [3] => 14 [4] => 15 ) [3] => Array ( [0] => 16 [1] => 17 [2] => 18 [3] => 19 [4] => 20 ) )
From the above example, we can see that using the for loop and array_slice() function can divide a large array into Multiple small arrays. This method is more flexible and can freely control the number of elements contained in each small array.
Notes
When using the array splitting function, you need to pay attention to the following points:
- The number of elements in the divided small array should be as even as possible to avoid Because a small array is too large, memory overflows or the program runs slowly.
- If you want to further process the divided small array, it is recommended to do it directly in the divided loop to avoid wasting time and memory by traversing the array multiple times.
Conclusion
Splitting a large array into multiple small arrays is one of the commonly used techniques in PHP programming. Using the array_chunk() function or for loop provided by PHP, you can quickly implement array segmentation and improve the efficiency of the program. In practical applications, it is necessary to choose the appropriate method according to the specific situation and pay attention to some details to achieve good results.
The above is the detailed content of php array is divided into several arrays. For more information, please follow other related articles on the PHP Chinese website!
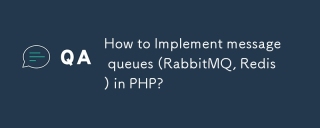
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
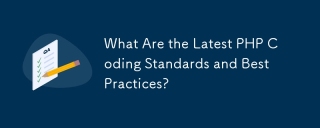
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
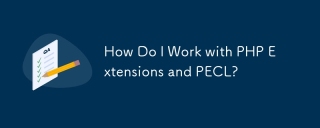
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
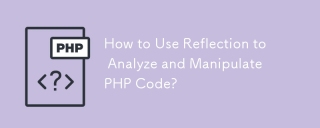
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
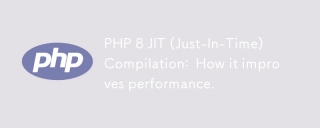
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
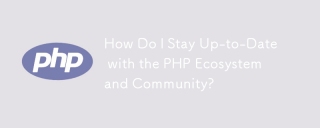
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
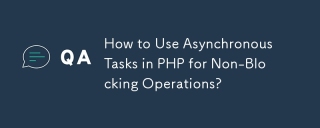
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
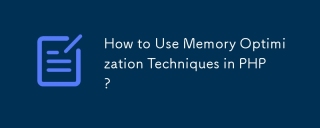
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
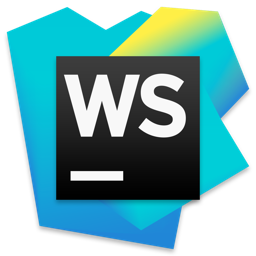
WebStorm Mac version
Useful JavaScript development tools
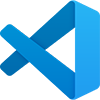
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
