(1) In TestServletRequest, store the information named "param" and value "HelloWorld" into the request range, and use the request forwarding method to redirect to another Servlet--AnotherServlet. In AnotherServlet, read the value of param from the request scope. Please complete the following Servlet program.
文件名:TestServletRequest.java @WebServlet("/TestServletRequest") public class TestServletRequest extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request._____1_____("param","HelloWorld"); RequestDispatcher rd=request.____2______("____3______"); rd._____4_____(request,response); } } 文件名:AnotherServlet.java @WebServlet("/AnotherServlet") public class AnotherServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String param= (String)request.____5______("param"); } }
1. setAttribute
2. getRequestDispatcher
3. /AnotherServlet
4. forward
5. getAttribute
(2) In the myweb application, a Servlet named HelloWorld is created, in which the request parameter values are read and output. Please complete the following Servlet program.
public class HelloWorld extends ____1____ { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //对用户提交的请求用utf-8来解码,否则会出现乱码 request.____2____("utf-8"); //通过设置Content-Type(内容类型),告诉浏览器接下来发送的是HTML,编码方式是UFT-8; response.____3____("text/html;charset=UTF-8"); //获取请求对象中name参数对应的值 String name = request.____4_____("name"); String greeting = "Hello " + name + "!"; //获取输出流对象 PrintWriter out = response.____5___(); out.println(greeting); } }
1. HttpServlet
2. setCharacterEncoding
3. setContentType
4. getParameter
5. getWriter
(3) In the myweb application, enter the user name and password on the form page, and use the "post" method to submit the form data to the Servlet program. The mapping url of the Servlet is configured as: /Process. Determine whether the entered user is "admin" in the Servlet. If so, redirect to another Servlet (Admin.java) whose mapping url is "/Admin".
Please complete the following Servlet program.
_____11_____//配置Servlet的mapping url public class Process extends HttpServlet { public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //获取请求参数值 String un = request._____2_____(“username”); if(un!=null ){ if(un._____3____(“admin”)){ response._____4_____(“____5_____”); } } } }
1. @WebServlet("/Precess")
2. getParameter
3. equals
4. sendRedirect
5. Admin
(4) In the myweb application, a Servlet is created named Servlet1, and its mapping url is: /Servlet1. Enter the address in the browser address bar to access the Servlet. Enter the address as follows:
http://localhost:8080/myweb/Servlet1?param1=111
Get the param1 parameter in this Servlet and pass it to another Servlet-Servlet2, and use forward request The method jumps to Servlet2.
Please complete the Servlet1 program below.
public class Servlet1 extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //获取名为param1的请求参数对应的值 String param1= request.____1______("___2____"); //保存到request范围中 request._____3_____(“param1”,param1); //获取转发器对象 RequestDispatcher rd=request._____4____("/Servlet2"); //使用转发请求方式跳转到Servlet2 rd.___5____(request,response); } }
1. getParament
2. paraml
3. setAttribute
4. getRequestDispatcher
5. forward
(5) Filter
@WebFilter( //仅对“/WebSite” URL格式进行过滤 urlPatterns = { " ___1____" }, initParams = { @WebInitParam(name = "site", value = "dalian") }) public class MyFilter implements Filter { protected String site; public void destroy() { } public void ___2 ___ (ServletRequest req, ServletResponse res, FilterChain fchain) throws IOException, ServletException { //如果site不为空,则输出site if(____ 3 _____) { System.out.println(“网站”+site); } ___4___.doFilter(req, res); } public void init(FilterConfig conf) throws ServletException { //获取@WebFilter注解中配置的初始化参数 this.site= ___5___.getInitParameter("site"); } }
1. /Website
2. doFilter
3. site!=null
4. fchain
5. conf
(6) In the myweb application, a Servlet named Servlet1 is created. Servlet1 stores an attribute named "name" in the session scope, and then uses redirection to jump. Go to Servlet2. Get the "name" attribute value in the session scope in Servlet2 and output it. Please complete the Servlet2 program below.
public class Servlet2 extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //获取当前会话对象 HttpSession session=request._____1_____(); //获取会话对象中的属性值 String name= (String)session._____2_____("_____3_____"); //获取输出流对象 PrintWriter out=response._____4_____(); //输出name out._____5_____(name); } }
1. getSession;
2. getAttribute;
3. name;
4. getWriter;
##5. printThe above is the detailed content of Java Web keyword fill-in-the-blank example analysis. For more information, please follow other related articles on the PHP Chinese website!
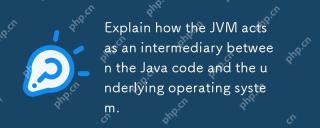
JVM works by converting Java code into machine code and managing resources. 1) Class loading: Load the .class file into memory. 2) Runtime data area: manage memory area. 3) Execution engine: interpret or compile execution bytecode. 4) Local method interface: interact with the operating system through JNI.

JVM enables Java to run across platforms. 1) JVM loads, validates and executes bytecode. 2) JVM's work includes class loading, bytecode verification, interpretation execution and memory management. 3) JVM supports advanced features such as dynamic class loading and reflection.

Java applications can run on different operating systems through the following steps: 1) Use File or Paths class to process file paths; 2) Set and obtain environment variables through System.getenv(); 3) Use Maven or Gradle to manage dependencies and test. Java's cross-platform capabilities rely on the JVM's abstraction layer, but still require manual handling of certain operating system-specific features.
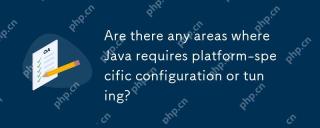
Java requires specific configuration and tuning on different platforms. 1) Adjust JVM parameters, such as -Xms and -Xmx to set the heap size. 2) Choose the appropriate garbage collection strategy, such as ParallelGC or G1GC. 3) Configure the Native library to adapt to different platforms. These measures can enable Java applications to perform best in various environments.
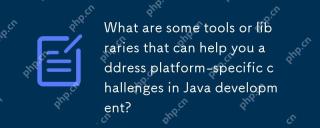
OSGi,ApacheCommonsLang,JNA,andJVMoptionsareeffectiveforhandlingplatform-specificchallengesinJava.1)OSGimanagesdependenciesandisolatescomponents.2)ApacheCommonsLangprovidesutilityfunctions.3)JNAallowscallingnativecode.4)JVMoptionstweakapplicationbehav
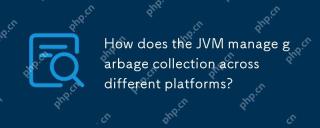
JVMmanagesgarbagecollectionacrossplatformseffectivelybyusingagenerationalapproachandadaptingtoOSandhardwaredifferences.ItemploysvariouscollectorslikeSerial,Parallel,CMS,andG1,eachsuitedfordifferentscenarios.Performancecanbetunedwithflagslike-XX:NewRa
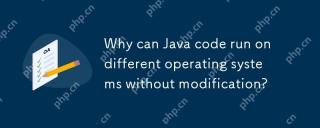
Java code can run on different operating systems without modification, because Java's "write once, run everywhere" philosophy is implemented by Java virtual machine (JVM). As the intermediary between the compiled Java bytecode and the operating system, the JVM translates the bytecode into specific machine instructions to ensure that the program can run independently on any platform with JVM installed.
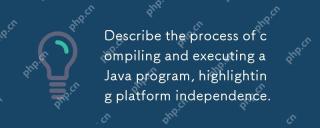
The compilation and execution of Java programs achieve platform independence through bytecode and JVM. 1) Write Java source code and compile it into bytecode. 2) Use JVM to execute bytecode on any platform to ensure the code runs across platforms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
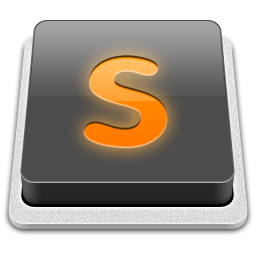
SublimeText3 Mac version
God-level code editing software (SublimeText3)
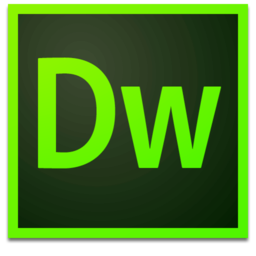
Dreamweaver Mac version
Visual web development tools
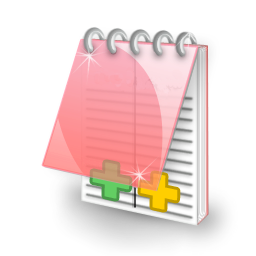
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
